Java에서 바코드 생성
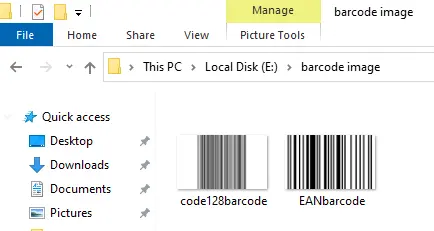
바코드는 바와 공백으로 구성됩니다. 너비가 다른 평행선 패턴의 형태로 기계가 읽을 수 있는 문자와 숫자의 표현일 뿐입니다.
이러한 패턴은 특정 제품의 번호, 위치 또는 이름을 식별하기 위해 소매 ID 카드, 상점 품목 및 우편물에 부착됩니다. 바코드 기호에는 여백(quiet zone), 시작 문자, 중지 문자, 데이터 문자 및 여백(quiet zone)의 다섯 가지 구성 요소가 있습니다.
바코드의 종류
바코드를 식별하기 위해 아래에 표시된 바코드 유형을 살펴보겠습니다.
- 선형(1D) 바코드
- 매트릭스(2D) 바코드
가장 많이 사용되는 바코드 형식은 UPC, Code 39, Extended Code39, Code 128, EAN 128 및 QR 코드입니다.
Java에서 가장 많이 사용되는 두 가지 라이브러리인 itextpdf
및 zxing
을 사용하여 바코드를 생성할 수 있습니다.
Java에서 zxing
라이브러리를 사용하여 바코드 생성
zxing
라이브러리를 사용하여 Java에서 바코드를 생성할 수 있습니다. zxing
은 얼룩말 횡단을 의미합니다.
이 라이브러리를 사용하려면 IntelliJ IDEA에서 다음 단계를 따라야 합니다.
-
Java 언어로 새 프로젝트를 만들고 시스템 maven을 빌드합니다.
-
pom.xml
을 열고 다음 코드를 작성합니다.<dependencies> <dependency> <groupId>com.google.zxing</groupId> <artifactId>core</artifactId> <version>3.3.0</version> </dependency> <dependency> <groupId>com.google.zxing</groupId> <artifactId>javase</artifactId> <version>3.3.0</version> </dependency> </dependencies>
-
maven 변경 사항 로드
버튼을 누르거나 단축키 ctrl+shift+o를 누릅니다.
종속성을 동기화할 때 프로그램에서 zxing
을 가져올 수 있습니다.
예:
이 코드는 주로 사용되는 두 바코드인 Code 128과 EAN 8의 이미지를 생성합니다. 컴퓨터에서 이미지의 경로를 만들어야 합니다.
package org.example;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.client.j2se.MatrixToImageWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.oned.Code128Writer;
import com.google.zxing.oned.EAN8Writer;
import java.nio.file.Paths;
public class Main {
public static void main(String[] args) {
try {
// Content for encode function of code128writer
String text = "http://www.google.com";
// Path where we save our barcode
String path = "E:\\barcode image\\code128barcode.jpg";
// Barcode code128 writer object
Code128Writer c128writer = new Code128Writer();
// Represents a 2D matrix of bits. Create a bar code with a width of 500 and a height of 300
BitMatrix matrix = c128writer.encode(text, BarcodeFormat.CODE_128, 500, 300);
MatrixToImageWriter.writeToPath(matrix, "jpg", Paths.get(path));
System.out.println("Barcode image file is created...");
// Digits for encode function of EAN_8
String digits = "12345678";
// Path where we save our barcode
path = "E:\\barcode image\\EANbarcode.jpg";
// Barcode EAN8 writer object
EAN8Writer EANwriter = new EAN8Writer();
/*Represents a 2D matrix of bits.
Create an EAN bar code of width 500 and height 300*/
matrix = EANwriter.encode(digits, BarcodeFormat.EAN_8, 500, 300);
MatrixToImageWriter.writeToPath(matrix, "jpg", Paths.get(path));
System.out.println("Barcode image file is created...");
} catch (Exception e) {
e.printStackTrace();
}
}
}
출력:
itextpdf
라이브러리를 사용하여 Java에서 바코드 생성
itextpdf
라이브러리 덕분에 Java로 바코드를 생성하고 PDF 형식으로 쉽게 저장할 수 있습니다. 이 라이브러리를 사용하려면 다음 단계를 따라야 합니다.
-
여기에서
itextpdf
라이브러리를 다운로드하십시오. -
IntelliJ IDEA를 엽니다.
-
Java 언어로 새 프로젝트를 만듭니다.
-
프로젝트 폴더를 마우스 오른쪽 버튼으로 클릭합니다.
-
모듈 설정을 열거나 F4를 누르십시오.
-
종속성
탭을 클릭합니다. -
추가
버튼을 누르거나 Alt+삽입을 누릅니다. -
itextpdf
웹사이트에서 다운로드한itextpdf.jar
을 선택합니다.
Java 코드에서 itextpdf
를 가져와서 바코드를 생성할 수 있습니다.
예:
이 예제의 도움으로 Code 128 및 EAN 13 바코드를 PDF 형식으로 저장할 수 있습니다.
package org.example;
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.Image;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.pdf.Barcode128;
import com.itextpdf.text.pdf.BarcodeEAN;
import com.itextpdf.text.pdf.PdfContentByte;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.FileOutputStream;
public class Main {
public static void main(String[] args) {
Main.barcodePDF("mybarcodepdf.pdf", "Zeshan");
}
public static void barcodePDF(String pdfFilename, String myString) {
// declare object of document class of the itextpdf library
Document doc = new Document();
PdfWriter pdfWriter = null;
try {
// initialize docWriter object with the path of the pdf file
// mention the path of your computer
pdfWriter =
PdfWriter.getInstance(doc, new FileOutputStream("E:\\my barcode pdf\\" + pdfFilename));
// set pdf file properties
doc.addAuthor("zeshan");
doc.addCreationDate();
doc.addProducer();
doc.addCreator("google.com");
doc.addTitle("java barcode generation");
doc.setPageSize(PageSize.A4);
doc.open();
/*PdfContentByte is an object that contains the user-positioned text and
graphic contents of a page*/
PdfContentByte cb = pdfWriter.getDirectContent();
// type of barcode
Barcode128 code128 = new Barcode128();
// remove all leading and trail space and pass to set code
code128.setCode(myString.trim());
code128.setCodeType(Barcode128.CODE128);
/*create the image of the barcode with null bar color and null text color
set its position and scale percent*/
Image code128Image = code128.createImageWithBarcode(cb, null, null);
code128Image.setAbsolutePosition(10, 700);
code128Image.scalePercent(150);
// Add image to pdf file
doc.add(code128Image);
// Type of barcode
BarcodeEAN codeEAN = new BarcodeEAN();
// Remove all leading and trail space and pass to set code
codeEAN.setCode(myString.trim());
// Set barcode type
codeEAN.setCodeType(BarcodeEAN.EAN13);
/* Create an image of the barcode with a null bar color and
null text color its position and scale percent */
Image codeEANImage = code128.createImageWithBarcode(cb, null, null);
codeEANImage.setAbsolutePosition(10, 600);
codeEANImage.scalePercent(150);
// Add image to pdf file
doc.add(codeEANImage);
System.out.println("Barcode in PDF file is created...");
} catch (Exception ex) {
ex.printStackTrace();
} finally {
// close doc and docwriter if they are not null
if (doc != null) {
doc.close();
}
if (pdfWriter != null) {
pdfWriter.close();
}
}
}
}
출력:
결론
바코드는 제품 데이터를 막대와 영숫자 문자로 새기므로 매장에 물건을 입력하거나 초과 창고에서 재고를 추적하는 것이 훨씬 빠르고 쉽습니다. 용이성과 속도 외에도 바코드의 두드러진 비즈니스 우위는 정확성, 내부 제어 및 가격 절감을 포함합니다.
바코드에는 다양한 형식이 있습니다. 우리는 상황에 따라 다른 형식을 사용합니다.
zxing
및 itextpdf
라이브러리의 도움으로 모든 바코드 형식을 사용할 수 있습니다. 다른 라이브러리도 있지만 이 두 라이브러리가 쉽고 빠릅니다.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn