Java でバーコードを生成する
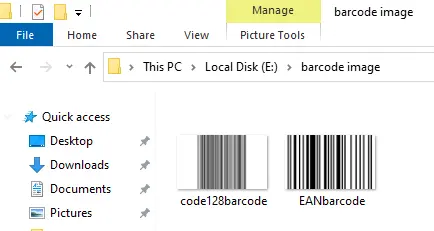
バーコードは、バーとスペースで構成されています。 これは、さまざまな幅の平行線のパターンの形式で文字と数字を機械で読み取り可能なものにすぎません。
これらのパターンは、特定の製品の番号、場所、または名前を識別するために、小売店の ID カード、店舗の商品、および郵便に添付されています。 バーコード シンボルには、クワイエット ゾーン、スタート キャラクタ、ストップ キャラクタ、データ キャラクタ、および別のクワイエット ゾーンの 5つのコンポーネントがあります。
バーコードの種類
バーコードを識別するために、以下に示すバーコードの種類を見てみましょう。
- リニア (1D) バーコード
- マトリックス (2D) バーコード
最もよく使用されるバーコード形式には、UPC、Code 39、Extended Code39、Code 128、EAN 128、および QR コードがあります。
itextpdf
と zxing
という 2つの最もよく使用されるライブラリを使用して、Java でバーコードを生成できます。
zxing
ライブラリを使用して Java でバーコードを生成する
zxing
ライブラリを使用して、Java でバーコードを生成できます。 zxing
は横断歩道を意味します。
このライブラリを使用するには、IntelliJ IDEA で次の手順に従う必要があります。
-
言語 Java とビルド システム maven で新しいプロジェクトを作成します。
-
pom.xml
を開き、次のコードを記述します。<dependencies> <dependency> <groupId>com.google.zxing</groupId> <artifactId>core</artifactId> <version>3.3.0</version> </dependency> <dependency> <groupId>com.google.zxing</groupId> <artifactId>javase</artifactId> <version>3.3.0</version> </dependency> </dependencies>
-
load maven changes
ボタンを押すか、ショートカット キー ctrl+shift+o を押します。
依存関係を同期すると、プログラムに zxing
をインポートできます。
例:
このコードは、主に使用される 2つのバーコード、Code 128 と EAN 8 の画像を生成します。コンピューター上に画像のパスを作成する必要があることを忘れないでください。
package org.example;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.client.j2se.MatrixToImageWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.oned.Code128Writer;
import com.google.zxing.oned.EAN8Writer;
import java.nio.file.Paths;
public class Main {
public static void main(String[] args) {
try {
// Content for encode function of code128writer
String text = "http://www.google.com";
// Path where we save our barcode
String path = "E:\\barcode image\\code128barcode.jpg";
// Barcode code128 writer object
Code128Writer c128writer = new Code128Writer();
// Represents a 2D matrix of bits. Create a bar code with a width of 500 and a height of 300
BitMatrix matrix = c128writer.encode(text, BarcodeFormat.CODE_128, 500, 300);
MatrixToImageWriter.writeToPath(matrix, "jpg", Paths.get(path));
System.out.println("Barcode image file is created...");
// Digits for encode function of EAN_8
String digits = "12345678";
// Path where we save our barcode
path = "E:\\barcode image\\EANbarcode.jpg";
// Barcode EAN8 writer object
EAN8Writer EANwriter = new EAN8Writer();
/*Represents a 2D matrix of bits.
Create an EAN bar code of width 500 and height 300*/
matrix = EANwriter.encode(digits, BarcodeFormat.EAN_8, 500, 300);
MatrixToImageWriter.writeToPath(matrix, "jpg", Paths.get(path));
System.out.println("Barcode image file is created...");
} catch (Exception e) {
e.printStackTrace();
}
}
}
出力:
itextpdf
ライブラリを使用して Java でバーコードを生成する
itextpdf
ライブラリの助けを借りて、Java でバーコードを生成し、それらを PDF 形式で簡単に保存できます。 このライブラリを使用するには、次の手順に従う必要があります。
-
ここ から
itextpdf
ライブラリをダウンロードします。 -
IntelliJ IDEA を開きます。
-
Java 言語で新しいプロジェクトを作成します。
-
プロジェクト フォルダーを右クリックします。
-
モジュール設定を開くか、F4 を押します。
-
依存関係
タブをクリックします。 -
add
ボタンを押すか、Alt+Insert を押します。 -
itextpdf
Web サイトからダウンロードしたitextpdf.jar
を選択します。
itextpdf
を Java コードにインポートして、バーコードを生成できます。
例:
この例を利用して、Code 128 および EAN 13 バーコードを PDF 形式で保存できます。
package org.example;
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.Image;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.pdf.Barcode128;
import com.itextpdf.text.pdf.BarcodeEAN;
import com.itextpdf.text.pdf.PdfContentByte;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.FileOutputStream;
public class Main {
public static void main(String[] args) {
Main.barcodePDF("mybarcodepdf.pdf", "Zeshan");
}
public static void barcodePDF(String pdfFilename, String myString) {
// declare object of document class of the itextpdf library
Document doc = new Document();
PdfWriter pdfWriter = null;
try {
// initialize docWriter object with the path of the pdf file
// mention the path of your computer
pdfWriter =
PdfWriter.getInstance(doc, new FileOutputStream("E:\\my barcode pdf\\" + pdfFilename));
// set pdf file properties
doc.addAuthor("zeshan");
doc.addCreationDate();
doc.addProducer();
doc.addCreator("google.com");
doc.addTitle("java barcode generation");
doc.setPageSize(PageSize.A4);
doc.open();
/*PdfContentByte is an object that contains the user-positioned text and
graphic contents of a page*/
PdfContentByte cb = pdfWriter.getDirectContent();
// type of barcode
Barcode128 code128 = new Barcode128();
// remove all leading and trail space and pass to set code
code128.setCode(myString.trim());
code128.setCodeType(Barcode128.CODE128);
/*create the image of the barcode with null bar color and null text color
set its position and scale percent*/
Image code128Image = code128.createImageWithBarcode(cb, null, null);
code128Image.setAbsolutePosition(10, 700);
code128Image.scalePercent(150);
// Add image to pdf file
doc.add(code128Image);
// Type of barcode
BarcodeEAN codeEAN = new BarcodeEAN();
// Remove all leading and trail space and pass to set code
codeEAN.setCode(myString.trim());
// Set barcode type
codeEAN.setCodeType(BarcodeEAN.EAN13);
/* Create an image of the barcode with a null bar color and
null text color its position and scale percent */
Image codeEANImage = code128.createImageWithBarcode(cb, null, null);
codeEANImage.setAbsolutePosition(10, 600);
codeEANImage.scalePercent(150);
// Add image to pdf file
doc.add(codeEANImage);
System.out.println("Barcode in PDF file is created...");
} catch (Exception ex) {
ex.printStackTrace();
} finally {
// close doc and docwriter if they are not null
if (doc != null) {
doc.close();
}
if (pdfWriter != null) {
pdfWriter.close();
}
}
}
}
出力:
まとめ
バーコードは製品データをバーと英数字に刻み込み、店舗で商品を入力したり、過剰な倉庫で在庫を追跡したりするのをはるかに迅速かつ簡単にします。 使いやすさとスピードに加えて、バーコードの優れたビジネス エッジには、正確性、内部統制、および価格の節約が含まれます。
バーコードには多くの形式があります。 さまざまな状況でさまざまな形式を使用します。
zxing
および itextpdf
ライブラリの助けを借りて、すべてのバーコード形式を使用できます。 他にもライブラリがありますが、この 2つは簡単で高速です。
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn