How to Create Animation in Java
- Setting Up Your JavaFX Project
- Creating a Simple Animation
- Adding Key Frames for Complex Animations
- Conclusion
- FAQ
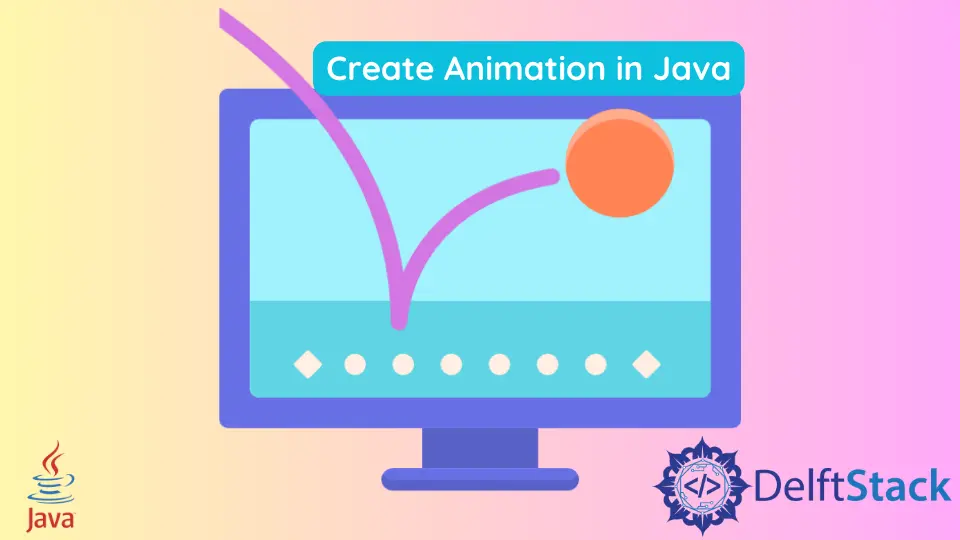
Creating animations can add a dynamic flair to your Java applications, making them more engaging and visually appealing. Whether you want to create simple animations for games or complex transitions for user interfaces, Java provides robust libraries and frameworks to help you achieve your goals.
In this tutorial, we will explore various methods to create animations in Java, focusing on the essential components and techniques you need to know. By the end of this guide, you will have a solid understanding of how to implement animations effectively within your Java projects.
Java offers several ways to create animations, primarily through the use of libraries like JavaFX and Swing. Each library has its own strengths and is suited for different types of applications. JavaFX is more modern and provides a rich set of features for developing animations, while Swing is a classic choice for desktop applications.
In this article, we will focus on using JavaFX to create animations, as it offers a more straightforward approach with better performance. We will cover the basics of setting up a JavaFX project, creating a simple animation, and exploring the use of key frames and transitions.
Setting Up Your JavaFX Project
Before diving into the animation code, you need to ensure that you have JavaFX set up in your development environment. If you are using an IDE like IntelliJ IDEA or Eclipse, you can easily integrate JavaFX by adding the necessary libraries.
- Download the JavaFX SDK from the official website.
- Configure your IDE to include the JavaFX library.
- Create a new Java project and set up the main application file.
Here’s a simple JavaFX application structure to get you started:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class AnimationExample extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("JavaFX Animation Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Output:
JavaFX application window with the title "JavaFX Animation Example"
This code sets up a basic JavaFX application with a window. The start
method is the entry point for the JavaFX application, and it creates a simple scene. Once you have this structure in place, you can start adding animations.
Creating a Simple Animation
Now that you have your JavaFX application set up, let’s create a simple animation. We will animate a circle moving across the screen. First, you need to import the necessary JavaFX classes for shapes and animations.
import javafx.animation.TranslateTransition;
import javafx.scene.shape.Circle;
import javafx.util.Duration;
public class AnimationExample extends Application {
@Override
public void start(Stage primaryStage) {
Circle circle = new Circle(50);
circle.setTranslateX(100);
circle.setTranslateY(100);
TranslateTransition transition = new TranslateTransition();
transition.setNode(circle);
transition.setDuration(Duration.seconds(2));
transition.setToX(200);
transition.setCycleCount(TranslateTransition.INDEFINITE);
transition.setAutoReverse(true);
transition.play();
StackPane root = new StackPane();
root.getChildren().add(circle);
Scene scene = new Scene(root, 400, 300);
primaryStage.setTitle("JavaFX Circle Animation");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this code, we create a Circle
object and set its initial position. The TranslateTransition
class is used to animate the circle’s movement. We specify the duration of the animation, the target position, and set it to cycle indefinitely while reversing back to the start position. When you run this code, you will see a circle smoothly moving back and forth across the screen.
Adding Key Frames for Complex Animations
For more complex animations, you can use key frames to define specific points in the animation timeline. This allows for greater control over the animation’s behavior. Here’s how to implement key frames in JavaFX.
import javafx.animation.KeyFrame;
import javafx.animation.Timeline;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
public class AnimationExample extends Application {
@Override
public void start(Stage primaryStage) {
Rectangle rectangle = new Rectangle(100, 50, Color.BLUE);
rectangle.setTranslateX(150);
rectangle.setTranslateY(100);
Timeline timeline = new Timeline();
timeline.getKeyFrames().addAll(
new KeyFrame(Duration.seconds(0), e -> rectangle.setTranslateX(150)),
new KeyFrame(Duration.seconds(1), e -> rectangle.setTranslateX(300)),
new KeyFrame(Duration.seconds(2), e -> rectangle.setTranslateX(150))
);
timeline.setCycleCount(Timeline.INDEFINITE);
timeline.play();
StackPane root = new StackPane();
root.getChildren().add(rectangle);
Scene scene = new Scene(root, 400, 300);
primaryStage.setTitle("JavaFX Rectangle Animation");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this example, we create a Rectangle
and use a Timeline
to define key frames at different points in time. Each key frame specifies the position of the rectangle at that moment. This method allows for precise control over the animation, enabling you to create more intricate movements. You can easily expand this by adding more key frames or changing the properties of the shapes being animated.
Conclusion
Creating animations in Java is a rewarding way to enhance your applications. With JavaFX, you have access to powerful tools and libraries that make it easier to implement smooth and engaging animations. By understanding the basics of setting up your project, creating simple animations, and using key frames for more complex effects, you can elevate your Java applications to a new level of interactivity. So, go ahead and experiment with different shapes, transitions, and animations to see what you can create!
FAQ
-
What is JavaFX?
JavaFX is a software platform for creating and delivering desktop applications with rich user interfaces. -
Can I use JavaFX for mobile applications?
While JavaFX is primarily designed for desktop applications, there are ways to deploy JavaFX applications on mobile platforms. -
What are key frames in JavaFX?
Key frames are specific points in time within an animation that define the properties of an animated object. -
Is JavaFX still actively maintained?
Yes, JavaFX is actively maintained and continues to receive updates and improvements. -
Can I create animations without using JavaFX?
Yes, you can create animations using the older Swing library, but JavaFX is recommended for new projects due to its modern features.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook