Anagram in Java
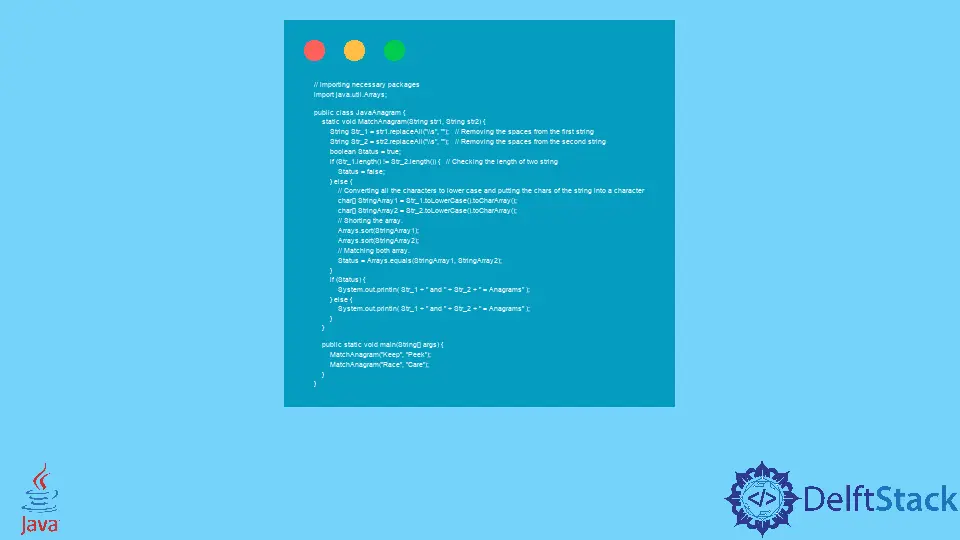
If you like to play with words, you may already know the term anagram. If two string contains the same character, but in a different word, they are called anagram of each other.
For example, if there are two words, Race
and Care
, then if you look at them, you will see that they contain the same character but in a different order.
So we can call the word Race
and Care
anagrams to each other.
In this article, we will see how we can find whether two words are anagrams or not. We will cover the topic with examples and explanations to make the topic easier.
Find Anagram in Java
In our example below, we illustrated how we could check whether the two given sentences are anagrams. Our example code will be like the following:
// importing necessary packages
import java.util.Arrays;
public class JavaAnagram {
static void MatchAnagram(String str1, String str2) {
String Str_1 = str1.replaceAll("\\s", ""); // Removing the spaces from the first string
String Str_2 = str2.replaceAll("\\s", ""); // Removing the spaces from the second string
boolean Status = true;
if (Str_1.length() != Str_2.length()) { // Checking the length of two string
Status = false;
} else {
// Converting all the characters to lower case and putting the chars of the string into a
// character
char[] StringArray1 = Str_1.toLowerCase().toCharArray();
char[] StringArray2 = Str_2.toLowerCase().toCharArray();
// Shorting the array.
Arrays.sort(StringArray1);
Arrays.sort(StringArray2);
// Matching both array.
Status = Arrays.equals(StringArray1, StringArray2);
}
if (Status) {
System.out.println(Str_1 + " and " + Str_2 + " = Anagrams");
} else {
System.out.println(Str_1 + " and " + Str_2 + " = Anagrams");
}
}
public static void main(String[] args) {
MatchAnagram("Keep", "Peek");
MatchAnagram("Race", "Care");
}
}
We already commanded the purpose of each line. The main steps that we follow in the programs are,
-
First, we removed all the spaces from the sentence.
-
Then, we checked whether the two sentences had the same length.
-
If they are the same length, we first converted all the characters to lowercase.
-
Now, we took all the characters of these two sentences in two different arrays and sorted the array.
-
Lastly, we matched whether both arrays contain the same element.
-
If they are matched, both sentences are anagrams.
After running the example, you will see the below output in your console:
Keep and Peek = anagrams
Race and Care = anagrams
Find Anagram in Java Using XOR
In our below example, we will illustrate how we can find anagrams in Java using bitwise XOR. The code will be as follows:
public class JavaAnagram {
public static void main(String[] args) {
// Declaring two string
String STR_1 = "Race";
String STR_2 = "Care";
if (AnagramChecking(STR_1, STR_2))
System.out.println(STR_1 + " & " + STR_2 + " = Anagrams");
else
System.out.println(STR_1 + " & " + STR_2 + " = Not Anagrams");
}
public static boolean AnagramChecking(String STR_1, String STR_2) {
// Remove all white spaces, convert to lower case & character array
char[] StringArr1 = STR_1.replaceAll("\\s", "").toLowerCase().toCharArray();
char[] StringArr2 = STR_2.replaceAll("\\s", "").toLowerCase().toCharArray();
if (StringArr1.length != StringArr2.length) // Matching the length
return false;
int DoXOR = 0;
for (int i = 0; i < StringArr1.length; i++) // Performing XOR operation
{
DoXOR ^= StringArr1[i] ^ StringArr2[i];
}
return DoXOR == 0 ? true : false;
}
}
We have indicated the purpose of each line. Now, after running the example code, you will see the below output in your console:
Race & Care = Anagrams
Find Anagram in Java Using HashMap
In our below example, we will illustrate how we can find anagrams in Java using HashMap. The code will be as follows:
// importing necessary packages
import java.util.HashMap;
public class JavaAnagram {
public static void main(String[] args) {
// Declaring two string
String STR_1 = "Race";
String STR_2 = "Care";
if (AnagramCheck(STR_1.toLowerCase(), STR_2.toLowerCase()))
System.out.println(STR_1 + " & " + STR_2 + " = Anagrams");
else
System.out.println(STR_1 + " & " + STR_2 + " = Not Anagrams");
}
public static boolean AnagramCheck(String STR_1, String STR_2) {
if (STR_1.length() != STR_2.length()) // Matching the length
return false;
HashMap<Character, Integer> MyMap = new HashMap<Character, Integer>(); // Declaring a hashmap
for (int i = 0; i < STR_1.length(); i++) {
char ch = STR_1.charAt(i);
if (MyMap.containsKey(ch))
MyMap.put(ch, MyMap.get(ch) + 1);
else
MyMap.put(ch, 1);
}
for (int i = 0; i < STR_2.length(); i++) {
char ch = STR_2.charAt(i);
if (MyMap.containsKey(ch)) {
if (MyMap.get(ch) == 1)
MyMap.remove(ch);
else
MyMap.put(ch, MyMap.get(ch) - 1);
} else
return false;
}
if (MyMap.size() > 0)
return false;
return true;
}
}
Now, after running the example code, you will see the below output in your console:
Race & Care = Anagrams
Please note that the code examples shared here are in Java, and you must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn