How to Compile Multiple Java Files Using a Single Command in Java
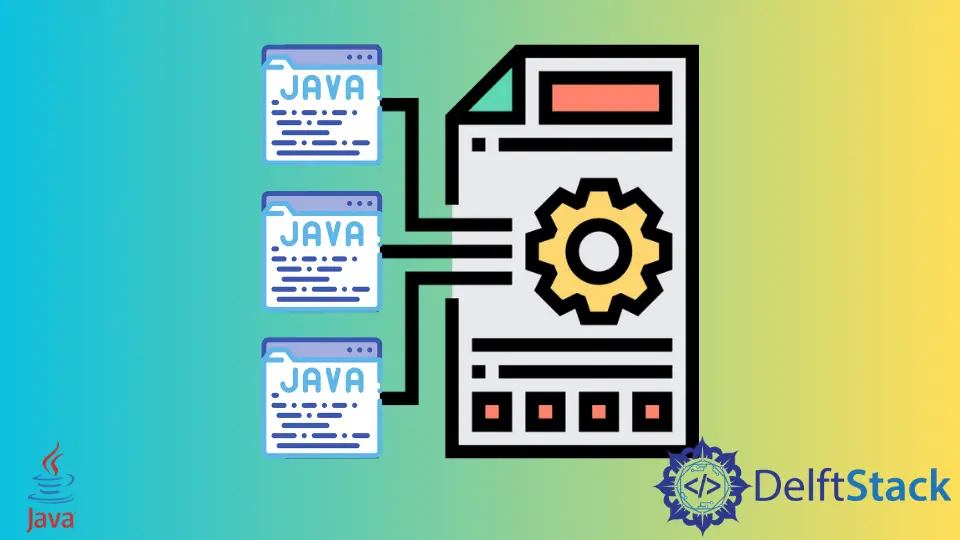
This tutorial introduces how to compile multiple java files using a single command in Java.
Compile is a term that is used to refer to a process of converting java source code to bytecode by using JDK. To execute any Java file, we need to follow two steps: compile the code and then run the compiled code.
During compilation, JDK checks the correctness and syntax of code and generates a .class
file that contains byte code that can be read by JVM only. Here, we will learn to compile single and multiple Java files by using single Java command.
So, let’s first start with compiling the Java file.
Compile a Java File
To understand how to compile multiple Java files with a single command, let’s first understand how to compile a single Java file. Java provides a javac command that compiles the source code and creates a .class file to compile a Java file.
Here, we created a Hello class and saved the code with the Hello.java name. To compile it, we used the javac
command.
See the example below.
class Hello {
public static void main(String[] args) {
System.out.println("Hello");
}
}
To compile the Java file, we used the below command. This command will create a .class file in the current directory.
javac Hello.java
To run the .class
file generated by the above command, we used the below java
command that executes the code using JVM.
java Hello
Output:
Hello
Compile Multiple Java Files
First, create two java files Hello.java and Hello2.java, and compile these by using the javac
command. We used the same command to compile the multiple Java files by providing the multiple Java file names.
See the example below.
File: Hello.java
class Hello {
public static void main(String[] args) {
System.out.println("Hello");
}
}
File: Hello2.java
class Hello2 {
public static void main(String[] args) {
System.out.println("Hello from 2");
}
}
To compile both the above Java files, we used the below command. This command will create two .class
files in the same directory as Hello.class
and Hello2.class
.
javac Hello.java Hello2.java
Compile All Java Files of the Current Directory
If we have multiple Java files in the current directory and want to compile all of them with a single Java command, we can use the below command. Here, we used a *
wild card to specify all the Java files.
javac *.java
If the Java files are in a different directory, then use the below command. Here, we specified the path of the directory and *
to compile all the Java files of the specified directory.
See the command below.
javac / root / rohan / directoryname/*.java