Java Conditional Compilation
- Understanding Conditional Compilation in Java
- Using Git Branches for Conditional Compilation
- Utilizing Git Tags for Version-Specific Compilation
- Conclusion
- FAQ
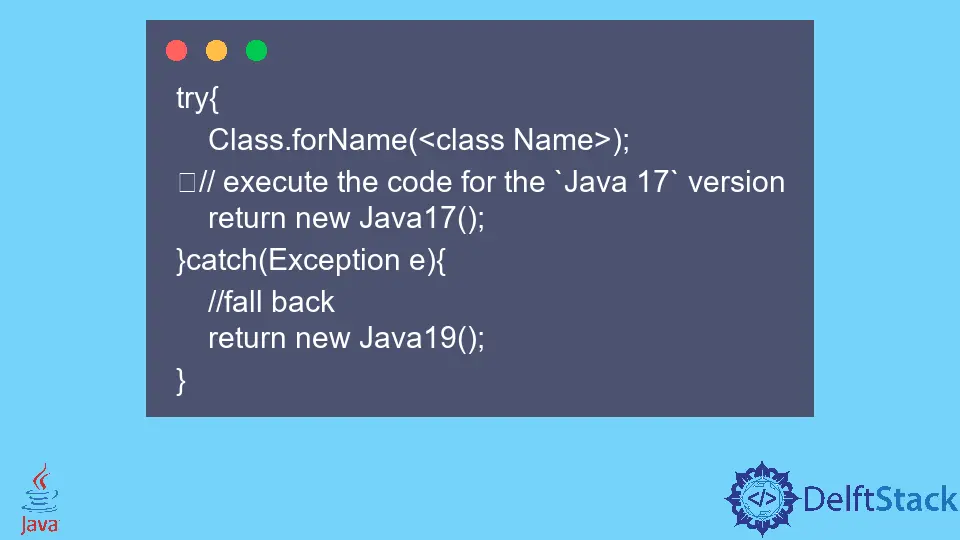
Java conditional compilation is a powerful technique that allows developers to compile different Java code snippets based on specific conditions or Java versions. This capability is particularly useful in large projects where code needs to adapt to different environments or versions without cluttering the codebase.
In this article, we will explore various methods to implement conditional compilation in Java, focusing on using Git for version control. Whether you’re maintaining legacy code or developing new features, understanding how to conditionally compile Java code can significantly enhance your development workflow. Let’s dive into the methods and examples that will help you master this essential skill.
Understanding Conditional Compilation in Java
Conditional compilation in Java isn’t a built-in feature like it is in some other programming languages. Instead, developers often rely on preprocessor-like techniques, version control systems, or build tools to achieve similar results. This can be especially critical when you need to maintain compatibility across various Java versions or when you want to enable or disable features based on certain conditions.
In this section, we will explore how to use Git to manage conditional compilation. Git allows you to create branches and manage different versions of your code effectively. By leveraging Git commands, you can streamline your workflow and ensure that your code is compiled conditionally based on the branch or tag you are working with.
Using Git Branches for Conditional Compilation
One effective way to manage conditional compilation in Java is to create different branches in your Git repository. Each branch can represent a different version of your code, allowing you to compile specific features or changes depending on the branch you are currently working on.
Here’s how you can set up your Git branches for conditional compilation:
- Create a new branch for your feature or version.
- Switch to that branch and make your changes.
- Use Git commands to compile your code based on the branch.
Here’s an example of how to create and switch branches in Git:
git checkout -b feature/new-feature
This command creates a new branch called feature/new-feature
and switches to it. You can now make your changes in this branch without affecting the main codebase.
Output:
Switched to a new branch 'feature/new-feature'
Once you’ve made your changes, you can compile your Java code. If you want to switch back to the main branch, use:
git checkout main
Output:
Switched to branch 'main'
By using branches, you can easily manage different versions of your code, allowing for conditional compilation based on the branch you’re working with. This method keeps your code organized and minimizes the risk of errors when switching between different versions.
Utilizing Git Tags for Version-Specific Compilation
Another effective method for conditional compilation in Java is to use Git tags. Tags in Git allow you to mark specific points in your repository’s history, which can represent different versions of your application or significant releases. By tagging your commits, you can easily compile code that corresponds to a specific version.
Here’s how to use Git tags for conditional compilation:
- Tag your current version.
- Checkout the tag when you need to compile that version.
First, let’s create a tag for your current version:
git tag -a v1.0 -m "Release version 1.0"
This command creates a new tag v1.0
and adds a message to it.
Output:
Tagged version 1.0
To compile the code for this version, you can check out the tag:
git checkout v1.0
Output:
Note: checking out 'v1.0'.
Using tags allows you to maintain a clean history of your project and easily compile different versions of your Java code. This method is particularly useful for teams working on multiple releases simultaneously, ensuring that everyone is on the same page regarding which version of the code they are working with.
Conclusion
Java conditional compilation is an essential skill for developers looking to maintain flexible and adaptable codebases. By leveraging Git branches and tags, you can effectively manage different versions of your Java code, allowing for easy compilation based on specific conditions. This approach not only enhances your workflow but also helps maintain the integrity of your code. As you implement these techniques, you will find that your development process becomes more streamlined and efficient, enabling you to focus on building great software.
FAQ
-
What is conditional compilation in Java?
Conditional compilation in Java refers to the practice of compiling different code snippets based on specific conditions or Java versions, often using version control systems like Git. -
How can I manage different versions of Java code?
You can manage different versions of Java code by using Git branches and tags to represent various features or releases, allowing for conditional compilation based on the branch or tag you are working with. -
Is there a built-in feature for conditional compilation in Java?
No, Java does not have a built-in feature for conditional compilation. Developers typically use Git or build tools to achieve similar results. -
How do Git branches help in conditional compilation?
Git branches allow you to create separate lines of development, enabling you to make changes and compile code specific to each branch without affecting the main codebase. -
What is the advantage of using Git tags for version management?
Git tags provide a way to mark specific points in your repository’s history, making it easy to compile and maintain different versions of your Java application.