How to Allow Only Numeric Input in HTML
-
Use
type="number"
in theinput
Tag to Allow Only Numeric Input in HTML - Write Client-Side Validation in JavaScript to Allow Only Numeric Input in HTML
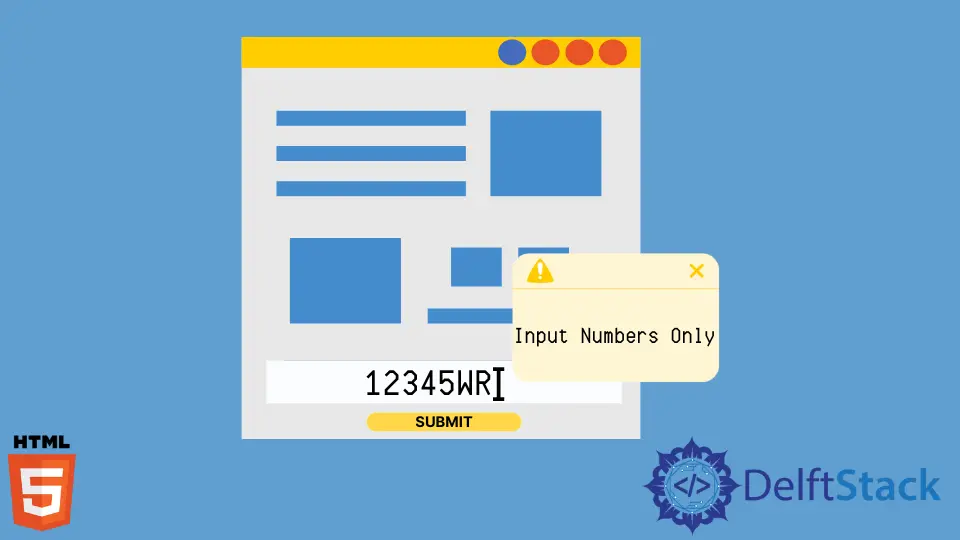
This article will introduce a few methods to allow only the numeric input in the text input field in HTML.
Use type="number"
in the input
Tag to Allow Only Numeric Input in HTML
In HTML, we use the input
tag to take the inputs from the user.
We can specify the input type with the type
attribute in the input
tag. The input
tag accepts various input types like text, numbers, passwords, email, etc.
We can use the type
attribute to allow only the numeric input in HTML. For example, create an input
tag with the type
attribute as number
.
Next, create a submit button. The form will not be submitted when we type any characters rather than numbers.
However, the behavior is different among the browsers.
In Firefox, the example below allows any input in the input area. But it does not let the value be submitted when clicking the button.
In the case of Chrome, it does not let any other characters be typed rather than the numeric characters. Firefox allows the other inputs to be pasted, but Chrome does not allow it.
For instance, if we copy some random text and paste it in the input area in Firefox, the text will be pasted, but this does not happen in Chrome. This behavior is also the same when dragging and dropping contents to the text area.
So, to add some validation, we can disable the drag and drop and paste operations in the text area. For instance, write the ondrop
and onpaste
attributes in the input
tag and write return false;
as their values.
By doing this, the copy-paste and drag and drop feature will be disabled in the text area.
Example Code:
<form action="">
<input type="number" ondrop="return false;" onpaste="return false;" />
<button type="submit" value="Submit">Click</button>
</form>
Write Client-Side Validation in JavaScript to Allow Only Numeric Input in HTML
While the method above does not work perfectly in all browsers, there is a need to add some client-side validation to allow typing only numeric values in the text area. For that, we need to write some JavaScript.
We need to add such validation where the input from the value 0-9
can only be accepted. For that, we have an event onkeypress
in JavaScript.
The onkeypress
event executes when the user presses the key. In the onkeypress
event, we can return only the values between 0-9
.
We can use the charCode
property to get the character code of the pressed input.
For example, write the onkeypress
event as an attribute in the input
tag.
Inside the attribute return the statement event.charCode>=48
and event.charCode<=57
using the &&
operator as shown in the example below. Then, write the required
attribute at the end of the input
tag.
In the example below, 48
and 57
are the Unicode character code of 0
and 9
, respectively. The JavaScript will only return the value from 0-9
.
It is how we can use the onkeypress
event along with the charCode
property to allow only numeric input in HTML.
Example Code:
<form>
<input type="number" ondrop="return false;" onpaste="return false;"
onkeypress="return event.charCode>=48 && event.charCode<=57" required/>
<button type="submit" value="Submit">Click</button>
</form>
Sushant is a software engineering student and a tech enthusiast. He finds joy in writing blogs on programming and imparting his knowledge to the community.
LinkedIn