How to Create the Currency Input in HTML
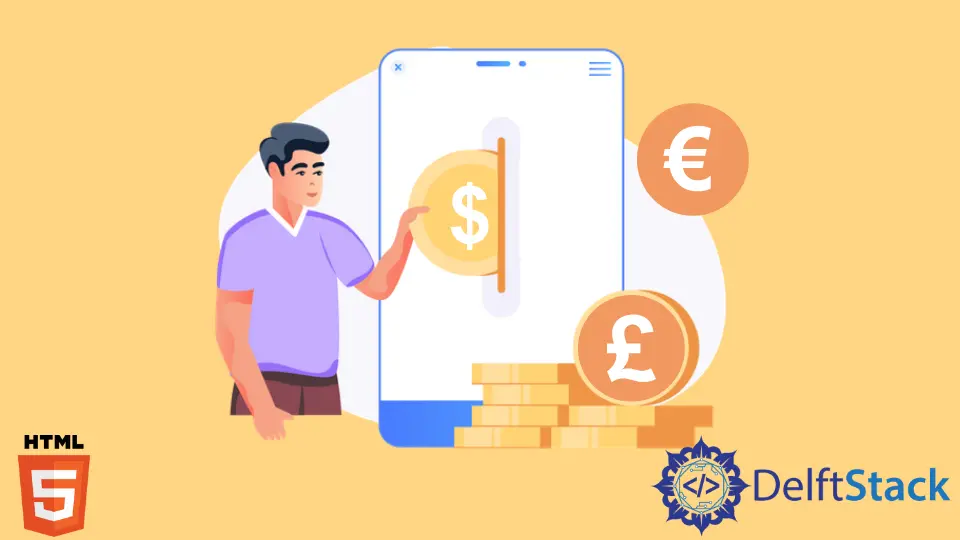
In this article, users will learn to convert the normal HTML <input>
field to the currency input field. We will use HTML and jQuery to achieve our goal.
Use the JavaScript toFixed()
Method to Convert Normal <input>
to Currency Input in HTML
In HTML, a normal <input>
element is available, and we can set its type according to our requirements. We cannot make the type a currency input by just setting the type to number
; we need to make more changes using either JavaScript or jQuery.
For example, we can create the <input>
field in HTML like below; it contains some attributes. The type
attribute suggests the type of input that it takes.
The min
and max
attributes suggest the minimum and maximum number values it takes, respectively. The id
attribute is the unique id of the <input>
element and using which we can access the element in jQuery.
<input type="number" id="currencyInput" min="0.1" max="5000000.0" value="99.9" />
We will use jQuery to convert the above <input>
field to a currency input. We have created the anonymous function and stored it in the inovkeCurrencyInput
variable.
Users can see how we can create an anonymous function in jQuery below.
$.fn.inovkeCurrencyInput = function() {
// function code
}
Now, we will add the change
event listener using jQuery on the input element inside the function. It will help us to detect the changes whenever we type something in the number input.
Here is the jQuery code to add the change
event listener inside the invokeCurrencyInput()
function.
$(this).change(function() {
});
After that, we will access the value of the number input using the valueAsNumber
property whenever a user adds or deletes something from the number input. Also, we will access the min
and max
attributes of the input field using the attr()
method.
After accessing the min
and max
attributes, we will parse the float value from it using the parseFloat()
JavaScript method, as shown below.
var currencyvalue = this.valueAsNumber;
var maxValue = parseFloat($(this).attr('max'));
var minValue = parseFloat($(this).attr('min'));
Now, we have minimum and maximum values that the currency input field can accept. Also, we have the current value of the input field stored in the currencyValue
variable.
Using the if
statement, we will check if currencyValue
is between the minValue
and maxValue
.
if (currencyvalue > maxValue) {
currencyvalue = maxValue;
}
if (currencyvalue < minValue) {
currencyvalue = minValue;
}
We will use the number.toFixed(precision)
method to round the currencyValue
to a particular precision. In our case, the precision value is 1, so whenever users enter an input value with more than 1 precision, an input field automatically rounds up the number value and sets single precision.
Here, we have shown how to use the number.toFixed()
method. Also, we will set the final formatted currencyValue
as a value of the <input>
element.
currencyvalue = currencyvalue.toFixed(1);
$(this).val(currencyvalue);
At last, we will call the invokeCurrencyInput()
function on the <input>
element wherever the document loads. Here, we access the <input>
element using its id.
$(document).ready(function() {
$('input#currencyInput').inovkeCurrencyInput();
});
Complete Source Code- HTML + jQuery:
We have also added the jQuery CDN in the below example code. Users must add it to invoke jQuery with the HTML code.
<html>
<body>
$
<input
type="number"
id="currencyInput"
min="0.1"
max="5000000.0"
value="99.9"
/>
<script
src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.js"
integrity="sha512-CX7sDOp7UTAq+i1FYIlf9Uo27x4os+kGeoT7rgwvY+4dmjqV0IuE/Bl5hVsjnQPQiTOhAX1O2r2j5bjsFBvv/A=="
crossorigin="anonymous"
referrerpolicy="no-referrer"
></script>
<script>
$.fn.inovkeCurrencyInput = function () {
$(this).change(function () {
var currencyvalue = this.valueAsNumber;
var maxValue = parseFloat($(this).attr("max"));
if (currencyvalue > maxValue) currencyvalue = maxValue;
var minValue = parseFloat($(this).attr("min"));
if (currencyvalue < minValue) currencyvalue = minValue;
currencyvalue = currencyvalue.toFixed(1);
$(this).val(currencyvalue);
});
};
$(document).ready(function () {
$("input#currencyInput").inovkeCurrencyInput();
});
</script>
</body>
</html>
The code will initially show the 99.9
value in the input field. If the user enters the value outside the 0.1
and 5000000.0
, it will set the 0.1
and 5000000.0
values accordingly; otherwise, our code will round up or down the value to a single precision digit and shows it in the input field.
Users need to press the enter key to submit and round the value.
In this way, we can convert the normal input field to the currency input field, which has limits of minimum and maximum, and also, users can enter values to some number of precision digits.