How to Hide HTML Buttons and Show Them Using Onclick
-
Use the CSS
display
Property to Show the Hidden Buttons in HTML -
Use the jQuery
show()
Method to Show the Hidden Buttons in HTML
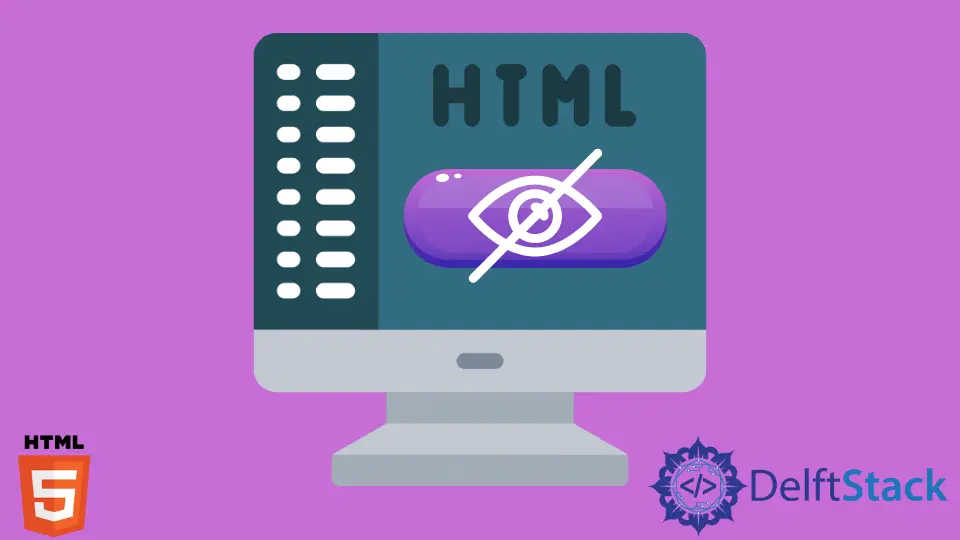
This tutorial will introduce a few methods to hide the HTML buttons and make them visible using the onclick
event.
Use the CSS display
Property to Show the Hidden Buttons in HTML
We can hide an HTML button first by setting its display
property to none
. Then, we can set the display
property to inline
or block
using JavaScript.
The display property inline
or block
will show the hidden HTML buttons. The difference between display: inline
and display: block
is that the inline component can have two or more components in a line or row.
But block
components can have only one component in a line or row.
For example, create a button and name it Show
. Set the onclick
attribute of the button to makeChange()
.
The makeChange()
function is called with the click of the button Show
. Then create the other three buttons and name them Button1
, Button2
and Button3
.
Set the id
of Button1
as b1
, Button2
as b2
, and Button3
as b3
. In CSS, select the buttons by their id
and set the display
property to none
.
Next, in JavaScript, create a function makeChange()
. Inside that function, set the display property of each button to block
.
Select the specific button by its id
as document.getElementById("b1")
for the first button. Then, set the display
by assigning document.getElementById("b1")style.display
to block
.
Repeat it for the other two buttons.
Example Code:
<button onclick="makeChange();">Show</button>
<button id="b1">Button1</button>
<button id="b2">Button2</button>
<button id="b3">Button3</button>
#b1, #b2, #b3 {
display: none;
}
function makeChange() {
document.getElementById('b1').style.display = 'block';
document.getElementById('b2').style.display = 'block';
document.getElementById('b3').style.display = 'block';
}
Use the jQuery show()
Method to Show the Hidden Buttons in HTML
We can also use the jQuery show()
function to show the hidden HTML elements. The show()
function only displays the selected HTML components whose display
property is set to none
.
It doesn’t work for HTML elements whose visibility
property is set to none
. We will use the same method as above to hide the buttons.
We will also reuse the HTML structure used in the method above.
After setting the display
property of the button to none
, create a makeChange()
function in JavaScript. Inside the function, select the buttons with their id
and call the jQuery show()
method as $('#b1, #b2, #b3').show()
.
When the Show
button is clicked, the hidden buttons will be displayed. Thus, we can use the jQuery show()
method to display the hidden buttons in HTML.
Example Code:
<button onclick="makeChange();">Show</button>
<button id="b1">Button1</button>
<button id="b2">Button2</button>
<button id="b3">Button3</button>
#b1, #b2, #b3 {
display: none;
}
function makeChange() {
$('#b1, #b2, #b3').show();
}