How to Change the Color of One Word in a String of Text in HTML
- Change the Color of One Word in a String of Text in HTML
- Change the Color of One Word in a String of Text in HTML Using Internal CSS
- Change the Color of One Word in a String of Text in HTML Using JavaScript
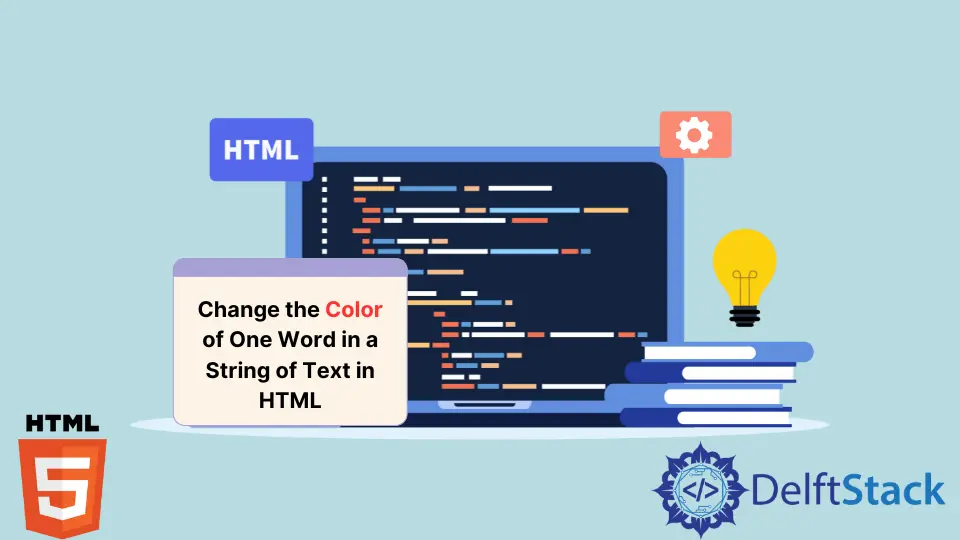
The main topic of this article is utilizing CSS to highlight or change the color of any particular word in a text. We’ll go over several techniques for implementing this feature.
We will learn to color a text using internal and inline CSS. Later, we’ll look at how to use JavaScript to implement the same functionality.
Change the Color of One Word in a String of Text in HTML
We commonly see on websites that a single word in a text has a different color than the others; of course, it captures the users’ attention. Let’s discuss how we can do the same on our web pages.
In the earlier versions of HTML, we had a <font>
tag that can be used to implement this feature like this:
<font color="red">Red</font>
But the tag is removed in HTML5 and is no longer supported. So, we will learn about an HTML <span>
tag to help us do the task.
The <span>
element is an inline container to mark up a section of a text or a section of a page. The id or class attribute of the <span>
tag allows simple styling with CSS and modifications with JavaScript.
The <span>
tag also allows us to apply inline styling, similar to the div
element. However, <span>
is an inline element, whereas div
is a block-level element.
Check the example below to understand this.
<html>
<body>
<p>Hello, I am
<span style="color: red">Red</span>
</p>
</body>
</html>
CSS’s color
property gives the text a specific color. There are many ways to specify the required color; in the above example, we select the color by its name.
HTML can recognize 16 color names which are black, white, grey, silver, maroon, red, purple, fuchsia, green, lime, olive, yellow, navy, blue, teal, and aqua. New browsers can recognize 140 CSS color names.
You can check all the HTML-recognized color names from here. As we mentioned, many other ways to specify the required color exist.
Let’s have a look at different methods.
RGB Colors
RGB stands for red, green, blue. It uses an additive color scheme in which the three primary colors, Red, Green, and Blue, are combined to create each color.
The red, green, and blue parameters each have a value between 0 and 255 that describes the color’s intensity. This indicates that there are 256 x 256 x 256 = 16,777,216 distinct colors.
For instance, rgb(255, 0, 0)
is rendered red because the color red is set to its greatest value, 255, while the other two colors, green and blue, are set to 0. Set all color parameters to zero like this rgb(0,0,0)
to display black.
You can see the RGB value of different colors from here.
<html>
<body>
<p>Hello, I am
<span style="color: rgb(241, 196, 15 )">Yellow</span>
</p>
</body>
</html>
RGBA Colors
RGBA colors are an extension of RGB colors, including an Alpha channel that determines a color’s opacity. The syntax for an RGBA color value is:
rgba (red, green, blue, alpha)
The value of the alpha parameter ranges from 0.0 (complete transparency) to 1.0 (full visibility). You can also use this property for the background colors, as sometimes we need different background colors with various opacity.
HEX Colors
Hex colors use hexadecimal values to represent colors from different color models. Hexadecimal colors are represented by the #RRGGBB
, where RR
stands for red, GG
for green, and BB
for blue.
The hexadecimal integers that specify the color’s intensity can range from 00
to FF
; an easy example is #0000FF
. Because the blue component is at its highest value of FF
while the red and green parts are at their lowest value of 00
, the color is entirely blue.
You can see the hex value of different colors from here.
<html>
<body>
<p>Hello, I am
<span style="color: #0000FF">Blue</span>
</p>
</body>
</html>
HSL Colors
HSL is an acronym that stands for Hue, Saturation, and Lightness. Let’s take a deeper look at each term.
- Hue: The hue ranges from 0 to 360 degrees on the color wheel. Red is 0; yellow is 60; green is 120; blue is 240, etc.
- Saturation: This quantity is measured as a percentage, with 100% denoting fully saturated (i.e., no shades of grey), 50% denoting 50% grey but with still-visible color, and 0% indicating entirely unsaturated (i.e., completely grey and color invisible).
- Lightness: This is also a percentage: 0% is black, and 100% is white. The amount of light we wish to give a color is expressed as a percentage, with 0% being black (where there is no light), 50% representing neither dark nor light, and 100% representing white (complete lightness).
The syntax for HSL color values is:
hsl(hue, saturation, lightness)
You can see the HSL value of different colors from here.
<html>
<body>
<p>Hello, I am
<span style="color: hsl(23, 97%, 50% )">Orange</span>
</p>
</body>
</html>
HSLA Colors
HSLA colors are an extension of HSL with an Alpha channel specifying a color’s opacity. An HSLA color value is determined with:
hsla(hue, saturation, lightness, alpha)
The value of the alpha parameter is a number having a range strictly between 0.0, which means fully transparent, and 1.0, which means not transparent.
Change the Color of One Word in a String of Text in HTML Using Internal CSS
We have seen in detail all the methods of giving color in CSS. We have been using inline CSS for everything up until this point.
However, inline CSS is not a suggested method because it is only tied to the element. We must rewrite much if we want the same functionality on different page regions.
So let’s color our text using Internal CSS, defined in the HTML <head>
tag inside a <style>
tag.
<html>
<head>
<title>CSS Color Property</title>
<style>
#rgb{
color:rgb(255,0,0);
}
#rgba{
color:rgba(255,0,0,0.5);
}
#hex{
color:#EE82EE;
}
#hsl{
color:hsl(0,50%,50%);
}
#hsla{
color:hsla(0,50%,50%,0.5);
}
#built{
color:green;
}
</style>
</head>
<body>
<h1>
Hello this is <span id="built">Built-in color</span> format.
</h1>
<h1>
Hello this is <span id="rgb">RGB</span> format.
</h1>
<h1>
Hello this is <span id="rgba">RGBA</span> format.
</h1>
<h1>
Hello this is <span id="hex">Hexadecimal</span> format.
</h1>
<h1>
Hello this is <span id="hsl">HSL</span> format.
</h1>
<h1>
Hello this is <span id="hsla">HSLA</span> format.
</h1>
</body>
</html>
Change the Color of One Word in a String of Text in HTML Using JavaScript
We can change the color of a specific word in a sentence using JavaScript. We need to give an ID to our <span>
tag and then get that element from JavaScript using document.getElementById(ID-name)
and call the style
property on it. Here’s how.
<html>
<body onload="myFunction()">
<p>Hello, I am <span id="color-text">Magenta.</span></p>
<script>
function myFunction() {
document.getElementById("color-text").style.color = "magenta";
}
</script>
</body>
</html>