Rune in Go
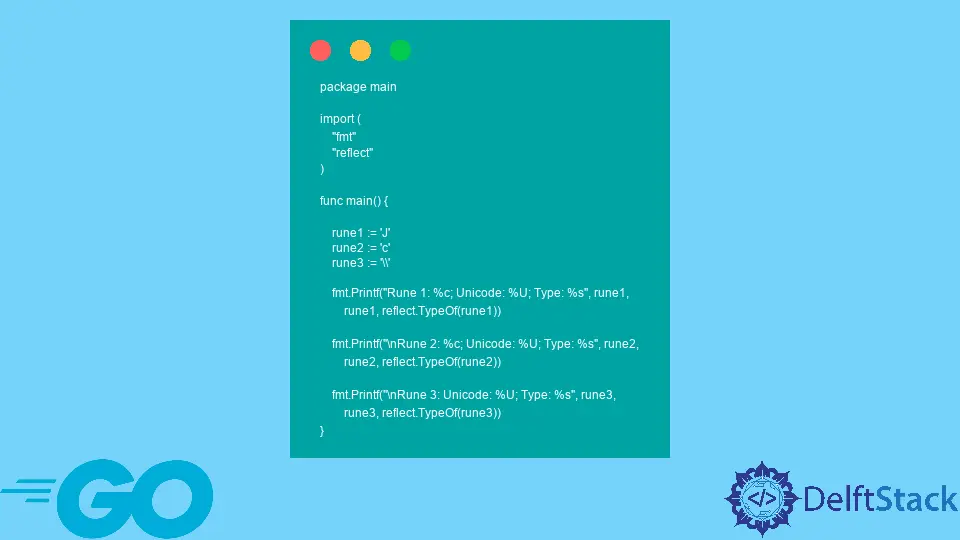
In Go, a rune is an alias for the int32 data type, representing Unicode code points. It refers to the numerical value allocated to a Unicode character.
A few years ago, we utilized the ASCII character set, which employs seven bits to represent one hundred and eighty-two characters, including capital letters, numerical values, etc. In contrast, the ASCII character set proved incapable of handling the world’s huge number of languages and symbols.
The Unicode character encoding was created to overcome this problem. It is a superset of ASCII character encoding, with a maximum of 1114112 Unicode code points.
Let’s look at some examples to have a better understanding.
Example Using Rune in Go
package main
import (
"fmt"
"reflect"
"unsafe"
)
func main() {
r := 'b'
fmt.Printf("Size: %d\n", unsafe.Sizeof(r))
fmt.Printf("Type: %s\n", reflect.TypeOf(r))
fmt.Printf("Unicode CodePoint: %U\n", r)
fmt.Printf("Character: %c\n", r)
s := "Jay Singh"
fmt.Printf("%U\n", []rune(s))
fmt.Println([]rune(s))
}
Output:
Size: 4
Type: int32
Unicode CodePoint: U+0062
Character: b
[U+004A U+0061 U+0079 U+0020 U+0053 U+0069 U+006E U+0067 U+0068]
[74 97 121 32 83 105 110 103 104]
Use Rune With Backslash \
in Go
All sequences that begin with a backslash are forbidden in rune literals.
package main
import (
"fmt"
"reflect"
)
func main() {
rune1 := 'J'
rune2 := 'c'
rune3 := '\\'
fmt.Printf("Rune 1: %c; Unicode: %U; Type: %s", rune1,
rune1, reflect.TypeOf(rune1))
fmt.Printf("\nRune 2: %c; Unicode: %U; Type: %s", rune2,
rune2, reflect.TypeOf(rune2))
fmt.Printf("\nRune 3: Unicode: %U; Type: %s", rune3,
rune3, reflect.TypeOf(rune3))
}
Output:
Rune 1: J; Unicode: U+004A; Type: int32
Rune 2: c; Unicode: U+0063; Type: int32
Rune 3: Unicode: U+005C; Type: %!s(int32=92)%!(EXTRA *reflect.rtype=int32)
Compare byte()
and rune()
in Go
Let’s output the byte array and rune of a string that contains non-ASCII characters in this example. The special Unicode character rune value is 214; however, encoding takes two bytes.
package main
import (
"fmt"
)
func main() {
s := "GÖ"
s_rune := []rune(s)
s_byte := []byte(s)
fmt.Println(s_rune)
fmt.Println(s_byte)
}
Output:
[71 214]
[71 195 150]