Go 中的符文
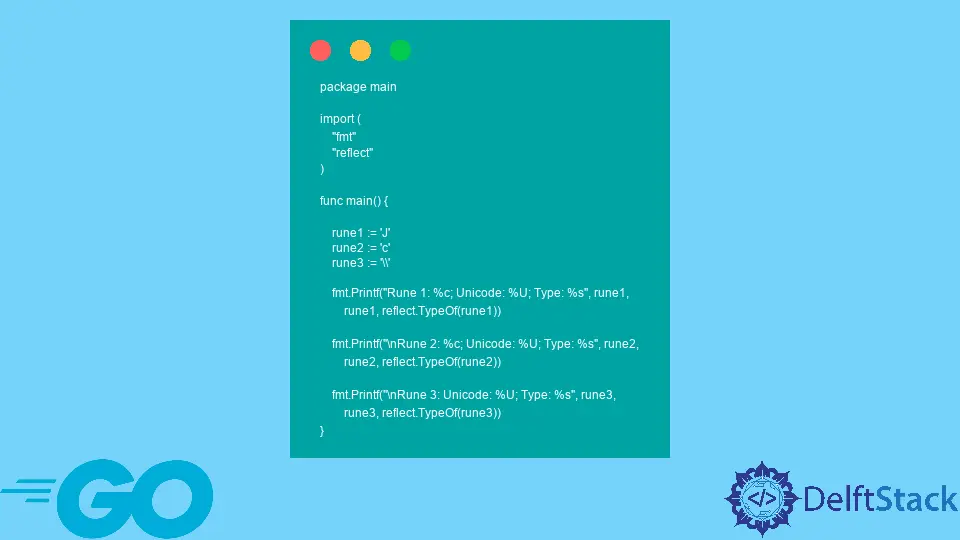
在 Go 中,rune 是 int32 資料型別的別名,表示 Unicode 程式碼點。它是指分配給 Unicode 字元的數值。
幾年前,我們使用 ASCII 字符集,它使用 7 位來表示 182 個字元,包括大寫字母、數值等。相比之下,ASCII 字符集被證明無法處理世界上龐大的數字的語言和符號。
建立 Unicode 字元編碼就是為了解決這個問題。它是 ASCII 字元編碼的超集,最多有 1114112 個 Unicode 程式碼點。
讓我們看一些例子來更好地理解。
在 Go 中使用 Rune 的示例
package main
import (
"fmt"
"reflect"
"unsafe"
)
func main() {
r := 'b'
fmt.Printf("Size: %d\n", unsafe.Sizeof(r))
fmt.Printf("Type: %s\n", reflect.TypeOf(r))
fmt.Printf("Unicode CodePoint: %U\n", r)
fmt.Printf("Character: %c\n", r)
s := "Jay Singh"
fmt.Printf("%U\n", []rune(s))
fmt.Println([]rune(s))
}
輸出:
Size: 4
Type: int32
Unicode CodePoint: U+0062
Character: b
[U+004A U+0061 U+0079 U+0020 U+0053 U+0069 U+006E U+0067 U+0068]
[74 97 121 32 83 105 110 103 104]
在 Go 中使用帶反斜槓 \
的符文
符文文字中禁止所有以反斜槓開頭的序列。
package main
import (
"fmt"
"reflect"
)
func main() {
rune1 := 'J'
rune2 := 'c'
rune3 := '\\'
fmt.Printf("Rune 1: %c; Unicode: %U; Type: %s", rune1,
rune1, reflect.TypeOf(rune1))
fmt.Printf("\nRune 2: %c; Unicode: %U; Type: %s", rune2,
rune2, reflect.TypeOf(rune2))
fmt.Printf("\nRune 3: Unicode: %U; Type: %s", rune3,
rune3, reflect.TypeOf(rune3))
}
輸出:
Rune 1: J; Unicode: U+004A; Type: int32
Rune 2: c; Unicode: U+0063; Type: int32
Rune 3: Unicode: U+005C; Type: %!s(int32=92)%!(EXTRA *reflect.rtype=int32)
比較 Go 中的 byte()
和 rune()
讓我們在這個例子中輸出一個包含非 ASCII 字元的字串的位元組陣列和符文。特殊的 Unicode 字元符文值是 214;但是,編碼需要兩個位元組。
package main
import (
"fmt"
)
func main() {
s := "GÖ"
s_rune := []rune(s)
s_byte := []byte(s)
fmt.Println(s_rune)
fmt.Println(s_byte)
}
輸出:
[71 214]
[71 195 150]
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe