How to Avoid Creating Duplicate Objects in Django
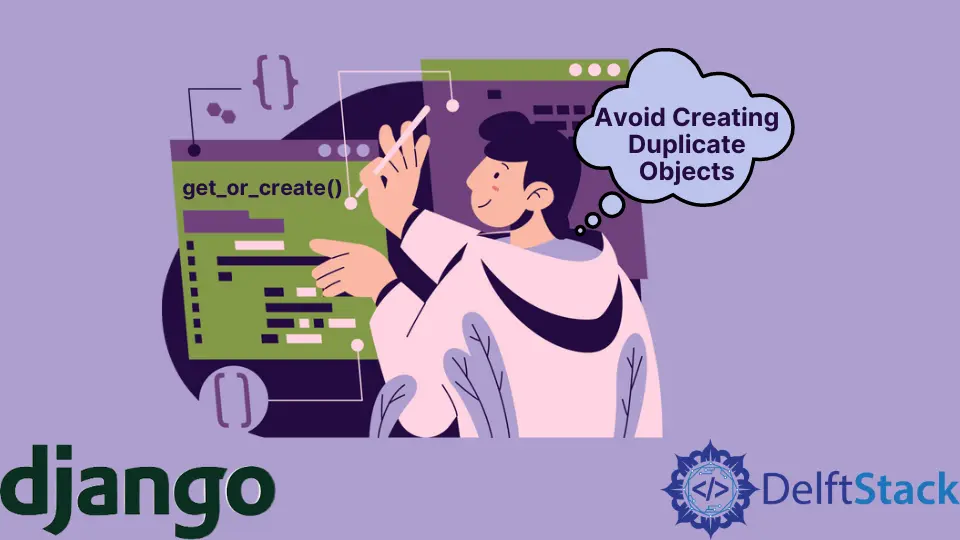
This article will tackle the get_or_create()
method in Django. A method used to avoid redundant fields in our query set.
Use the get_or_create()
Method in Django
In Django, we have a way to avoid defined get or create code using the get_or_create()
method. When we create duplicate objects multiple times, this method helps us avoid creating them multiple times.
try:
friend = Friend.objects.get(name="Harry jeams")
except DoesNotExist:
friend = Friend(name="Harry jeams")
friend.save()
In this block, when we get a friend
object from our query set, suppose we could not find this object, then we try to create this object. If we are going to receive several requests for executing other instructions simultaneously, it looks like a racing situation.
It might be possible that the process raises an exception as no object exists in the query set because multiple and duplicate objects have been created cause of threading.
We have an efficient way to correctly use the get_or_create
method, and this method responds quickly with a single operation.
friend, friend_to_friend = Friend.objects.get_or_create(name="Harry jeams")
The first thing the get_or_create()
method does is try to get an object using the get()
method. Unfortunately, if the first try fails to get an object, it will create an object in our query set.
If the output shows an "IntegrityError"
, it will only get an object and return it. If both processes execute simultaneously and get instructions to process and try to create an object, both will create objects that would be the duplicate object in our query set.
Since this will create duplicates, we need the unique object in our model field.
class Friend(models.Model):
friend_first_name = models.CharField(max_length=255)
friend_last_name = models.CharField(max_length=255)
friend, friend_to_friend = Friend.objects.get_or_create(
first_name="Harry", last_name="jeams"
)
The following code works to get and create a unique object in the query set.
Code Example:
class Friend(models.Model):
friend_first_name = models.CharField(max_length=255)
friend_last_name = models.CharField(max_length=255)
class SubClass:
unique = ("first_name", "last_name")
friend, friend_to_friend = Author.objects.get_or_create(
first_name="Harry", last_name="jeams"
)
Django’s get_or_create()
function returns two variables. The first is an object, and the second is a Boolean variable.
Sometimes we only need to get the object that it returns, so we use this syntax.
Syntax:
friend, friend_to_friend = Friend.objects.get_or_create(
name="Harry jeams", defaults={"list_of_friend": 0, "place": "college"}
)
If we have only one field in our query set, we can use defaults__exact
instead of the default parameter in the get_or_create()
method.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn