Django에서 중복 객체 생성 방지
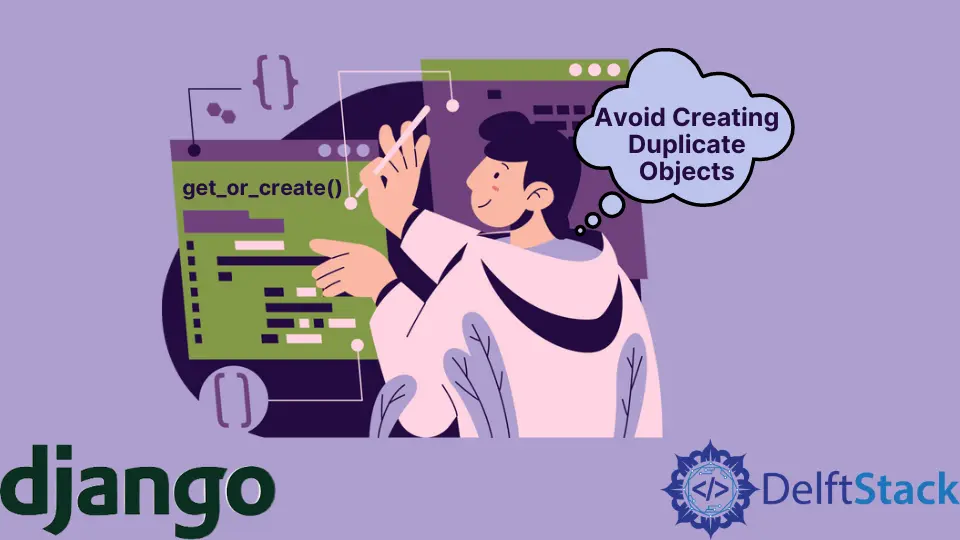
이 기사에서는 Django의 get_or_create()
메소드를 다룰 것입니다. 쿼리 세트에서 중복 필드를 방지하는 데 사용되는 방법입니다.
Django에서 get_or_create()
메서드 사용
Django에는 get_or_create()
메소드를 사용하여 정의된 get 또는 create 코드를 피하는 방법이 있습니다. 중복 개체를 여러 번 만들 때 이 방법을 사용하면 개체를 여러 번 만들지 않아도 됩니다.
try:
friend = Friend.objects.get(name="Harry jeams")
except DoesNotExist:
friend = Friend(name="Harry jeams")
friend.save()
이 블록에서 쿼리 세트에서 친구
개체를 가져올 때 이 개체를 찾을 수 없다고 가정하고 이 개체를 만들려고 합니다. 다른 명령을 동시에 실행하기 위한 여러 요청을 받을 경우 경주 상황처럼 보입니다.
스레딩으로 인해 여러 개의 중복 개체가 생성되어 쿼리 집합에 개체가 없으므로 프로세스에서 예외가 발생할 수 있습니다.
get_or_create
메서드를 올바르게 사용하는 효율적인 방법이 있으며 이 메서드는 단일 작업으로 빠르게 응답합니다.
friend, friend_to_friend = Friend.objects.get_or_create(name="Harry jeams")
get_or_create()
메서드가 수행하는 첫 번째 작업은 get()
메서드를 사용하여 개체를 가져오는 것입니다. 안타깝게도 첫 번째 시도에서 개체를 가져오지 못하면 쿼리 세트에 개체가 생성됩니다.
출력에 "IntegrityError"
가 표시되면 개체를 가져와서 반환하기만 합니다. 두 프로세스가 동시에 실행되고 처리 명령을 받고 객체 생성을 시도하면 둘 다 쿼리 세트에서 중복 객체가 될 객체를 생성합니다.
이렇게 하면 복제본이 생성되므로 모델 필드에 고유한 개체가 필요합니다.
class Friend(models.Model):
friend_first_name = models.CharField(max_length=255)
friend_last_name = models.CharField(max_length=255)
friend, friend_to_friend = Friend.objects.get_or_create(
first_name="Harry", last_name="jeams"
)
다음 코드는 쿼리 세트에서 고유한 개체를 가져오고 만드는 데 작동합니다.
코드 예:
class Friend(models.Model):
friend_first_name = models.CharField(max_length=255)
friend_last_name = models.CharField(max_length=255)
class SubClass:
unique = ("first_name", "last_name")
friend, friend_to_friend = Author.objects.get_or_create(
first_name="Harry", last_name="jeams"
)
Django의 get_or_create()
함수는 두 개의 변수를 반환합니다. 첫 번째는 개체이고 두 번째는 부울 변수입니다.
때때로 반환하는 객체만 가져오면 되므로 이 구문을 사용합니다.
통사론:
friend, friend_to_friend = Friend.objects.get_or_create(
name="Harry jeams", defaults={"list_of_friend": 0, "place": "college"}
)
쿼리 세트에 필드가 하나만 있는 경우 get_or_create()
메서드의 기본 매개변수 대신 defaults__exact
를 사용할 수 있습니다.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn