How to Check if Object Exists in Django
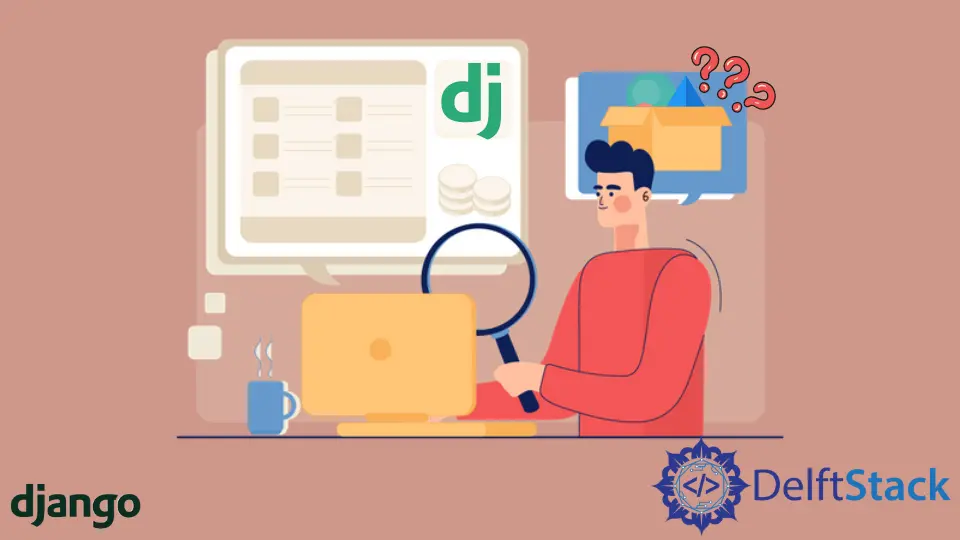
In this explanation of the common issue, you will see how to check if an object exists in the model, and you will also learn how to handle exceptions to show the convenient error to the user.
Use the exists()
Method to Check if an Object Exists in the Model in Django
For example, we have a model that is called Audio_store
. This class has a record
field that contains FileField
.
class Audio_store(models.Model):
record = models.FileField(upload_to="documents/")
class Meta:
db_tables = "Audia_store"
Now we need to check whether some value is present or not. Django has a built-in function to check whether an object does exist or not.
The exists()
function can be used for different situations, but we use it with the if
condition.
Suppose we have the Audio_get
function in the views.py
file, and in this function, we will return an HTTP response if the condition goes True
or False
. Let’s see if it is working or not.
def Audio_get(request):
vt = Audio_store.objects.all()
if vt.exists():
return HttpResponse("object found")
else:
return HttpResponse("object not found")
Before running the server, we need to make sure that we have added the URL in the urls.py
file. When we hit the URL, it will pull out the defined function from the views.py
file, and in our case, we defined the Audio_get
function.
Let’s run the server, open your browser, and go through with the localhost where your Django server is running.
We can see no error has occurred, and we got an HTTP response.
To handle a missing object from a dynamic URL, we can use the get_object_or_404
class. When we are trying to use an object that does not exist, we see an exception that is not user-friendly.
We can show the user (page not found
) error instead of the built-in Django exception; it will be a valid error. To use this error, we took an example code to raise an error if the Django server fails to find the object.
from .models import Product
from django.shortcuts import render, get_object_or_404
def dynamic_loockup_view(request, id):
# raise an exception "page not found"
obj = get_object_or_404(Product, id=id)
OUR_CONTEXT = {"object": obj}
return render(request, "products/product_detail.html", OUR_CONTEXT)
It is going to raise a 404 page
error.
One more way to do this is to put it inside a try
block. We need to import the Http404
class, which will raise that 404 page
like this get_object_or_404
class.
from django.http import Http404
from django.shortcuts import render
def dynamic_loockup_view(request, id):
try:
obj = Product.objects.get(id=id)
except Product.DoesNotExist:
# raise an exception "page not found"
raise Http404
OUR_CONTEXT = {"object": obj}
return render(request, "products/product_detail.html", OUR_CONTEXT)
It will also raise a 404 page
using Http404
when the Product
or model object does not exist.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn