How to Create Responsive Fonts in CSS
- Use the Viewport Value to Create Responsive Fonts in CSS
- Use Media Queries to Create Responsive Fonts in CSS
-
Use the
calc()
Function to Create Responsive Fonts in CSS -
Use the
clamp()
Function to Create Responsive Fonts in CSS
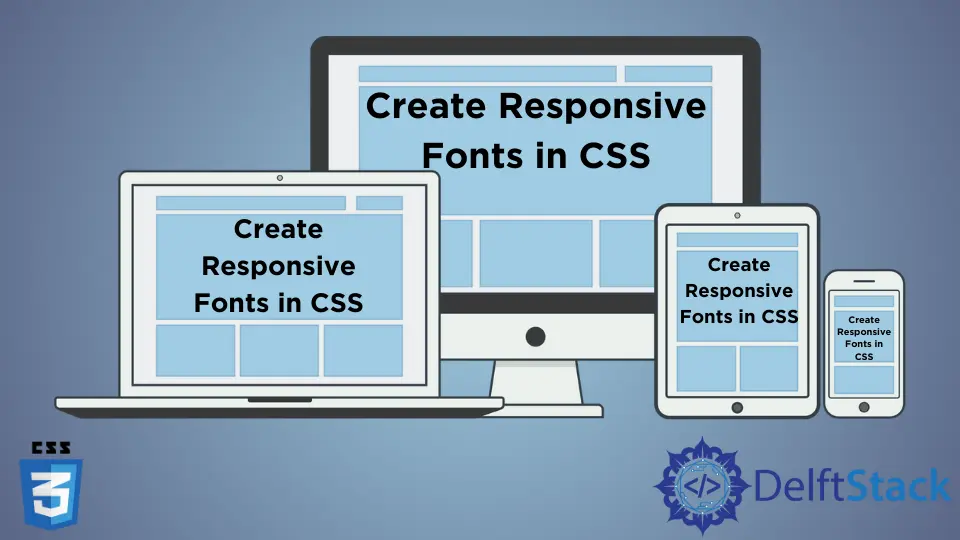
The article explains the ways to implement responsive fonts only with using CSS. We will introduce several ways how modern CSS and CSS3 make the fonts look readable across devices of different screen sizes.
Use the Viewport Value to Create Responsive Fonts in CSS
One of the easiest ways to make fonts responsive is to resize the fonts according to the viewport’s size or the browser’s window size. There are various viewport units, in relation to the axes of the browser window, provided by CSS that are very useful in making responsive web pages. Some viewport units are vw
, vh
, vmin
, and vmax
. We can get the information of these units from the CSS units. We can use these viewport units instead of em
, px
, or pt
to make the responsive fonts.
For example, create each of the h1
, h2
, and p
tags. Write some text of your choice between these tags. In CSS, set the font-size
property of the h1
, h2
, and p
tags to 5.9vw
, 3.0vh
and 2vmin
respectively.
When we resize the browser, the viewport of the browser changes, and the font size of the texts will also change accordingly. In this way, we can use the viewport units in the font size to create a responsive font in CSS.
Example Code:
<h1> Hey boy </h1>
<h2> Hey girl </h2>
<p> Hey kid </p>
h1 {
font-size: 5.9vw;
}
h2 {
font-size: 3.0vh;
}
p {
font-size: 2vmin;
}
Use Media Queries to Create Responsive Fonts in CSS
We can use the media queries to create responsive fonts in CSS. We can also utilize media queries to change the font size at a certain height/width of the device. We use the @media
keyword to write the media query.
For example, set the font-size
propety to 3em
. Next write the media query with the condition of max-width:320px
. Then, set the font-size
property to 2em
.
Example Code:
body{
font-size: 3em;
}
@media only screen and (max-width: 320px) {
body {
font-size: 2em;
}
}
<h1> Hey boy </h1>
<h2> Hey girl </h2>
<p> Hey kid </p>
Here, the font size will reduce when the code above is implemented and is run on devices with a screen size less than 320px
. The font is set as 2em
for devices having a height below 320px
. And fonts will be at 3em
for devices having a screen size greater than 320px
.
The demerit of this method is we might need to add more media queries if the design starts breaking in devices with a specific size.
Use the calc()
Function to Create Responsive Fonts in CSS
Since most modern browsers nowadays support the calc()
method in CSS, it makes writing responsive code easier. Additionally, this method is also more effective and achieves the task without making the code messy. We can use the calc()
function to make the font size responsive. The result of the function is the value of the property.
For example, set the font-size
property of body
tag to the calc()
function. Inside the function, write 0.75em + 1vw
.
Example Code:
body {
font-size: calc(0.75em + 1vw);
}
<h1> Hey boy </h1>
<h2> Hey girl </h2>
<p> Hey kid </p>
The font size will vary as the value of 1vw
changes on devices with different screen sizes. Thus, we can create a responsive font in CSS.
Use the clamp()
Function to Create Responsive Fonts in CSS
The clamp()
function clamps the values between the maximum and minimum values. The function takes three parameters as an argument, the first one is the minimum value, the second one is the preferred value, and the last one is the maximum permitted value. We can use the clamp()
function to set any property such as length
, angle
, percentage
, number
, etc. We can use the function to make the responsive font in CSS.
Example Code:
h1{
font-size : clamp(2rem, 10vw, 5rem);
}
<h1> Hey boy </h1>
<h2> Hey girl </h2>
<p> Hey kid </p>
In the example above, the value 2rem
is the minimum-allowed font size. Similarly, the next value, 10vw
, is the default font size and, the last one, 5rem
, is the maximum allowed font size. Here, we need to note that the value in the middle should be relative as in vw
, ch
, or vh
and not absolute like, px
and pt
.