How to Set Up C# on Mac OS
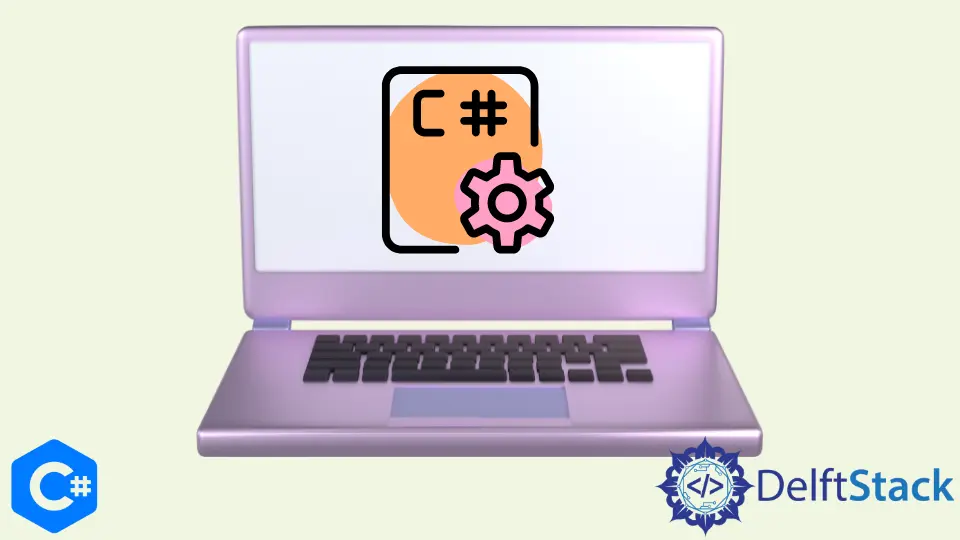
This article will demonstrate the complete setup and basic syntax of the C# language on Mac OS.
Install and Set Up C# on a Mac OS
The following are the steps to install and set up C# on your Mac.
-
The first step is to get an IDE on your computer. Visual Studio Code is the best option because it has all of the features you need and is very easy to use.
-
Visit the Visual Studio website. From the four selections, choose Visual Studio Code and click the
Download for Mac
button. -
Unzip the package and drag Visual Studio Code into your Applications folder once downloaded. After that, you may access it from the same folder.
-
The C# extension can then be downloaded by entering the Extensions pane within Visual Studio Code with the shortcut Shift+Cmd+X or by selecting
Code > Preferences > Extensions
. -
Type
C#
into the search bar in the extensions display and find Microsoft’s one. Click install and restart your Visual Studio Code after installing the C# extension.
Start a New Project in C# on Mac OS
Open the Terminal found in Applications > Utilities
on your Mac to start your first project. For example, write dotnet new console -o projectName
on your Terminal to build a console application.
Then, open Visual Studio Code and navigate to the folder you defined in Terminal. Finally, open the Program.cs
file from the left-hand Explorer to begin programming.
C# Syntax in Its Most Basic Form
When it comes to understanding fundamentals, C# is comparatively a simple language. It’s not as easy to learn as Python, but it’s also not as difficult as C++.
We’ll start with the most basic programming tasks: Showing text on the screen and accepting user input.
When you open the Program.cs
file, you’ll get the following boilerplate code:
using System;
namespace projectName {
class Program {
static void Main(string[] args) {
Console.WriteLine("Hello World");
}
}
}
Output:
Hello World
Let’s experiment with a few lines of code to see what we can come up with.
Declare a variable data
of string data type.
string data;
We need to show a message to ask something from the user. Let’s ask their name.
Console.WriteLine("What's your name?");
Then we need to retrieve the user’s input and store it to the data
variable we defined. To do this, we utilize a function named Console.ReadLine()
that accepts user input.
data = Console.ReadLine();
After that, it’s only a matter of displaying the information we’ve gathered on the screen.
Console.WriteLine("Hello " + data);
Because the user’s name was saved in the data
variable, this line will output the static Hello
with the name provided.
Complete code:
using System;
namespace ProjectName {
class Program {
static void Main(string[] args) {
string data;
Console.WriteLine("What's your name?");
data = Console.ReadLine();
Console.WriteLine("Hello " + data);
}
}
}
Output:
What's your name?
Henry
Hello Henry
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn