How to MySql Connection in C#
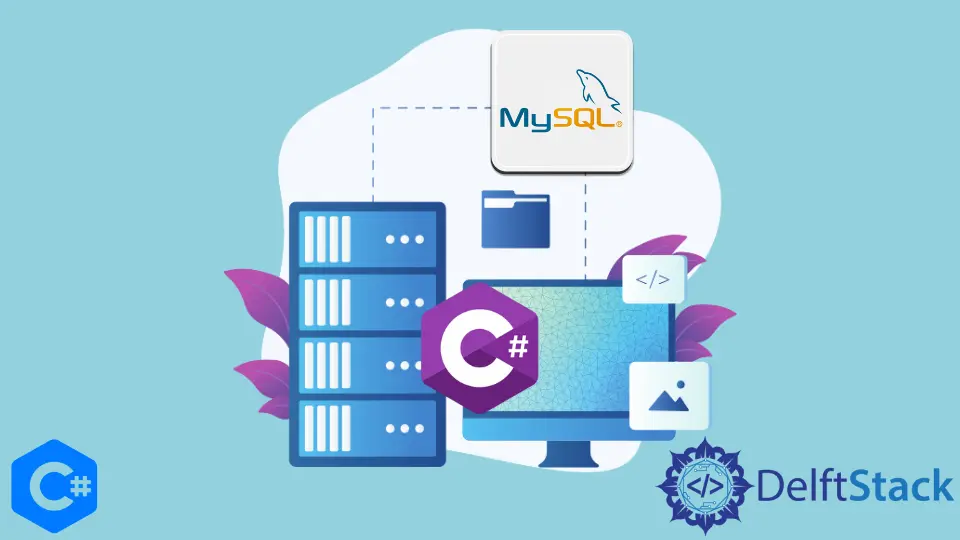
This tutorial will discuss the methods to connect to a MySql database in C#.
Connect to MySql Database With the MySql.Data
Package in C#
For this tutorial, we assume that you already have the MySql database installed on your machine and are just looking for the code to connect with it in C#. The MySql.Data
package performs operations on the MySql database in C#. The MySql.Data
is an external package and needs to be installed before using it. The following command is used to install the MySql.Data
package in Visual Studio.
Install-Package MySql.Data
We need to import the package MySql.Data.MySqlClient
for connecting to the MySql database in C#.
using MySql.Data.MySqlClient;
The MySqlConnection
class represents an open connection to a MySql database in C#. We can pass the connection string to the constructor of the MySqlConnection
class to initialize a new instance of the MySqlConnection
class that can connect to our database. The MySqlConnection.Open()
function opens the connection for performing any operation on the MySql database. The MySqlConnection.Close()
function closes the previously open connection to the MySql database. Any open connections must be closed with the MySqlConnection.Close()
function after the operations are performed. The following code example shows us how to connect to a MySql database with the MySql.Data
package in C#.
using System;
using MySql.Data.MySqlClient;
namespace mysql {
class Program {
private MySqlConnection conn;
static void connect() {
string server = "localhost";
string database = "mysqldb1";
string user = "root";
string password = "u1s2e3r4";
string port = "3306";
string sslM = "none";
string connString =
String.Format("server={0};port={1};user id={2}; password={3}; database={4}; SslMode={5}",
server, port, user, password, database, sslM);
conn = new MySqlConnection(connString);
try {
conn.Open();
Console.WriteLine("Connection Successful");
conn.Close();
} catch (MySqlException e) {
Console.WriteLine(e.Message + connString);
}
}
static void Main(string[] args) {
connect();
}
}
}
Output:
Connection Successful
We first created string variables that contain credential information about our database and combined them to form the connString
. The connString
variable is used in the constructor of the MySqlConnection
class to initialize its instance conn
. The connection to the database is opened for operations with the conn.Open()
function and closed after all the operations are performed with the conn.Close()
function in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn