How to Make a Delay Timer in C#
-
Use the
Thread.Sleep()
Method to Make a Delay in C# -
Use the
Task.Delay()
Method to Make a Delay in C# -
Use the
System.Timers.Timer
Class to Make a Delay in C# -
Use the
System.Threading.Timer
Class to Make a Delay in C# - Conclusion
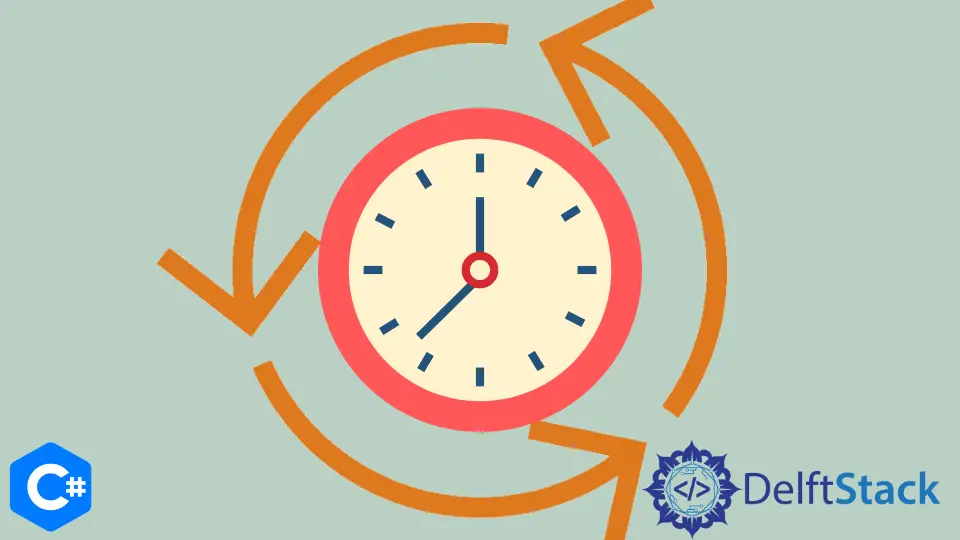
When writing C# applications, incorporating delays can be crucial for various scenarios, from timing operations to creating pauses in execution.
In this article, we’ll explore four different methods to add delays in C#: Thread.Sleep()
, Task.Delay()
, System.Timers.Timer
, and System.Threading.Timer
.
Each method has its use cases, and we’ll provide working examples for a 2-second delay using each approach.
Use the Thread.Sleep()
Method to Make a Delay in C#
One straightforward way to implement a delay in your C# program is by using the Thread.Sleep()
method. This method is part of the System.Threading
namespace in C#.
It has a simple syntax:
Thread.Sleep(int milliseconds);
Here, the milliseconds
parameter is an integer representing the duration of the delay in milliseconds. The method instructs the program to pause execution for a specified amount of time.
Let’s take a look at an example that demonstrates the use of Thread.Sleep()
to create a 2-second delay:
using System;
using System.Threading;
class DelayExample {
static void Main() {
Console.WriteLine("The delay starts.");
int delayMilliseconds = 2000;
Thread.Sleep(delayMilliseconds);
Console.WriteLine("The delay ends.");
}
}
Here, we first include the necessary namespace with using System.Threading;
.
We then print The delay starts.
to the console, indicating the point in the program before the delay is introduced. The variable delayMilliseconds
is set to 2000
, representing a 2-second delay.
The Thread.Sleep(delayMilliseconds);
line is where the delay occurs. The program will pause execution for the specified duration.
After the delay, The delay ends.
is printed to the console, marking the point in the program after the delay has concluded.
When you run this program, you will observe the following output:
This output confirms that the program pauses for 2 seconds between the The delay starts.
and The delay ends.
messages. The Thread.Sleep()
method effectively introduces the desired delay, allowing you to control the timing of your C# programs.
Use the Task.Delay()
Method to Make a Delay in C#
In addition to Thread.Sleep()
, C# offers another method for introducing delays in a more modern and asynchronous manner—Task.Delay()
. This method is part of the System.Threading.Tasks
namespace and is particularly useful when working with asynchronous programming.
The Task.Delay()
method has a flexible syntax, supporting various overloads. For a basic delay, the syntax is as follows:
await Task.Delay(int milliseconds);
Here, the milliseconds
parameter is an integer representing the duration of the delay in milliseconds. The await
keyword is used because Task.Delay()
is often employed in asynchronous contexts, allowing other operations to proceed while waiting.
Let’s take a look at an example that demonstrates how to use Task.Delay()
to create a 2-second delay:
using System;
using System.Threading.Tasks;
class DelayExample {
static async Task Main() {
Console.WriteLine("Before the delay");
int delayMilliseconds = 2000;
await Task.Delay(delayMilliseconds);
Console.WriteLine("After the delay");
}
}
To begin with, we have included the required namespace by using the statement using System.Threading.Tasks;
. The Main
method serves as the entry point for the program and is marked with the async
keyword, allowing the use of await
.
Similar to the previous example, we print Before the delay
to the console, indicating the point in the program before the delay is introduced. The variable delayMilliseconds
is set to 2000
, representing a 2-second delay.
The await Task.Delay(delayMilliseconds);
line is where the delay occurs. Unlike Thread.Sleep()
, Task.Delay()
doesn’t block the entire thread; it releases control, making it more suitable for asynchronous scenarios.
After the delay, After the delay
is printed to the console, marking the point in the program after the delay has concluded.
Output:
Before the delay
After the delay
Task.Delay()
provides an asynchronous way to introduce delays in your C# programs, enabling more responsive and efficient code execution.
Use the System.Timers.Timer
Class to Make a Delay in C#
In addition to the synchronous Thread.Sleep()
and the asynchronous Task.Delay()
, C# provides a more versatile way to implement delays using System.Timers.Timer
. This approach is especially beneficial when you need to schedule periodic tasks or perform actions after a specific interval.
To use it for creating a delay, you can set the interval and handle the Elapsed
event. The basic syntax is as follows:
Timer timer = new Timer();
timer.Interval = intervalInMilliseconds;
timer.Elapsed += TimerElapsed;
timer.AutoReset = false; // Set to true for periodic execution
timer.Start();
Here, intervalInMilliseconds
is the time between timer events, and TimerElapsed
is the method that will be executed when the timer elapses. The AutoReset
property determines whether the timer should reset and continue firing events.
Let’s see an example demonstrating the use of System.Timers.Timer
for a 2-second delay:
using System;
using System.Timers;
class DelayExample {
static void Main() {
Console.WriteLine("Before the delay");
Timer timer = new Timer();
timer.Interval = 2000;
timer.Elapsed += TimerElapsed;
timer.AutoReset = false;
timer.Start();
Console.ReadLine();
}
private static void TimerElapsed(object sender, ElapsedEventArgs e) {
Console.WriteLine("After the delay");
}
}
In this example, we start by printing Before the delay
to the console, signaling the point in the program before the delay. We then create an instance of System.Timers.Timer
and configure it to elapse every 2 seconds.
The TimerElapsed
method is the callback executed when the timer elapses. In this case, it prints After the delay
to the console.
The Console.ReadLine()
is added to prevent the application from exiting immediately, allowing time for the timer to elapse.
Output:
Before the delay
After the delay
System.Timers.Timer
provides a flexible and event-driven approach to implementing delays, offering more control over the timing of your C# programs.
Use the System.Threading.Timer
Class to Make a Delay in C#
For precise control over timed operations in C#, the System.Threading.Timer
class offers another powerful tool. Unlike the previously discussed System.Timers.Timer
, this class is part of the System.Threading
namespace and operates on a thread pool thread.
Using System.Threading.Timer
involves creating an instance of the timer, specifying a callback method, and setting the due time and period. The basic syntax is as follows:
Timer timer = new Timer(TimerCallback, null, dueTimeInMilliseconds, Timeout.Infinite);
Here, TimerCallback
is the method to be executed, null
is the state object (can be used to pass data to the callback method), dueTimeInMilliseconds
is the initial delay, and Timeout.Infinite
means the timer doesn’t repeat.
Here’s an example that demonstrates the use of System.Threading.Timer
to create a 2-second delay:
using System;
using System.Threading;
class DelayExample {
static void Main() {
Console.WriteLine("Before the delay");
Timer timer = new Timer(TimerCallback, null, 2000, Timeout.Infinite);
Console.ReadLine();
}
private static void TimerCallback(object state) {
Console.WriteLine("After the delay");
}
}
Similar to the previous example, we start by printing Before the delay
to the console, signaling the point in the program before the delay. We then create an instance of System.Threading.Timer
and configure it with a 2-second delay.
The TimerCallback
method is the callback executed when the timer elapses. In this case, it prints After the delay
to the console.
The Console.ReadLine()
is added to prevent the application from exiting immediately, allowing time for the timer to elapse.
Output:
Before the delay
After the delay
System.Threading.Timer
provides a flexible and thread-safe approach to implementing delays, making it suitable for various timing scenarios in C# programs.
Conclusion
In this article, we’ve covered four different methods to add delays in C#. Depending on your application’s requirements and the need for synchronous or asynchronous delays, you can choose the method that best fits your scenario.
Whether it’s a simple Thread.Sleep()
, an asynchronous Task.Delay()
, or the flexibility of timer-based delays, these methods provide you with the tools to control the timing of your C# programs.