How to Get the Unix Timestamp in C#
-
C# Program to Get the Unix Timestamp Using the
DateTime.Now.Subtract().TotalSeconds
Method -
C# Program to Get the Unix Timestamp Using the
DateTimeOffset.Now.ToUnixTimeSeconds()
Method -
C# Program to Get the Unix Timestamp Using the
TimeSpan
Struct Methods - Conclusion
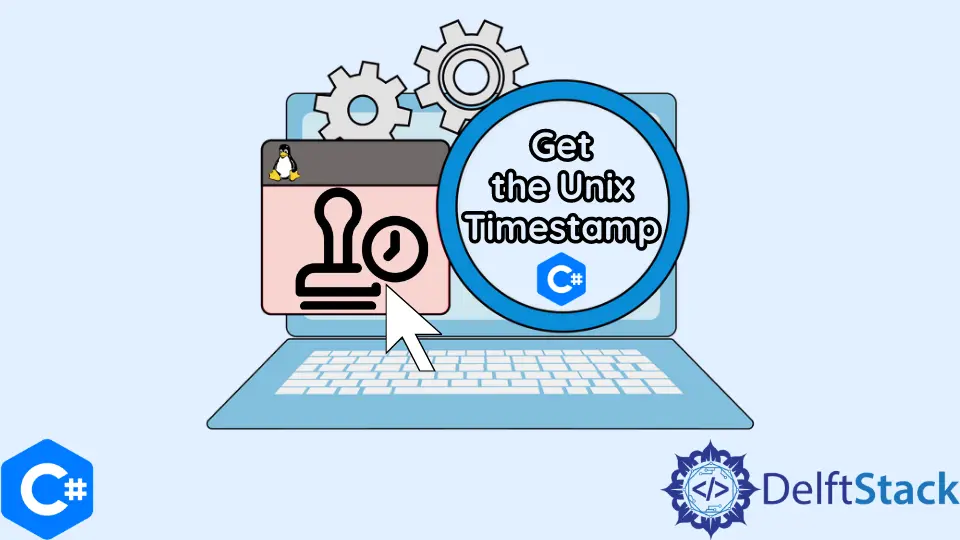
A Unix timestamp is mainly used in the Unix operating systems. But it is helpful for all operating systems because it represents the time of all time zones.
Unix timestamps represent the time in seconds. The Unix epoch started on 1st January 1970, so the Unix timestamp is the number of seconds between a specific date and the Unix epoch.
In C#, there are different methods to get a Unix timestamp. In this article, we are going to put our focus on these methods.
C# Program to Get the Unix Timestamp Using the DateTime.Now.Subtract().TotalSeconds
Method
In C#, obtaining the Unix timestamp can be achieved in various ways. One of the commonly used methods involves utilizing the DateTime.Now
property and the Subtract
method.
This combination allows for a straightforward calculation of the elapsed time since the Unix epoch.
Let’s explore a simple yet effective example of how to get the Unix timestamp in C# using DateTime.Now
and the Subtract
method.
Code Input:
using System;
class Program {
static void Main() {
// Get the current UTC time
DateTime currentTime = DateTime.Now.ToUniversalTime();
// Calculate the Unix timestamp
long unixTimestamp =
(long)(currentTime - new DateTime(1970, 1, 1, 0, 0, 0, DateTimeKind.Utc)).TotalSeconds;
// Display the result
Console.WriteLine("Unix Timestamp: " + unixTimestamp);
}
}
We begin by declaring a DateTime
variable named currentTime
to store the current UTC time. The DateTime.Now
property provides the current local time, and we convert it to UTC using ToUniversalTime()
to maintain consistency in our timestamp calculation.
Next, we calculate the Unix timestamp by subtracting the Unix epoch (January 1, 1970) from the current UTC time. The result is a TimeSpan
representing the elapsed time.
To convert the TimeSpan
into seconds, we access the TotalSeconds
property, which provides the total number of seconds represented by the TimeSpan
.
Finally, we display the Unix timestamp using Console.WriteLine()
.
Code Output:
Unix Timestamp: 1701851743
C# Program to Get the Unix Timestamp Using the DateTimeOffset.Now.ToUnixTimeSeconds()
Method
One elegant approach involves using the DateTimeOffset.Now.ToUnixTimeSeconds
method chain. This method chain not only gets the current time but also converts it to a Unix timestamp in a concise manner.
Let’s delve into a practical example that demonstrates how to get the Unix timestamp in C# using the DateTimeOffset.Now.ToUnixTimeSeconds
method chain.
Code Input:
using System;
class Program {
static void Main() {
// Get the current UTC time with offset
DateTimeOffset currentDateTimeOffset = DateTimeOffset.Now;
// Extract Unix timestamp using ToUnixTimeSeconds method
long unixTimestamp = currentDateTimeOffset.ToUnixTimeSeconds();
// Display the result
Console.WriteLine("Unix Timestamp: " + unixTimestamp);
}
}
We initiate by creating a DateTimeOffset
variable named currentDateTimeOffset
. This object encapsulates the current date and time along with the system’s offset from Coordinated Universal Time (UTC).
Following that, we utilize the ToUnixTimeSeconds
method directly on our currentDateTimeOffset
variable. This method is a convenient addition to the DateTimeOffset
structure that directly converts the instance to a Unix timestamp measured in seconds.
Finally, we print the Unix timestamp to the console using Console.WriteLine()
.
Code Output:
Unix Timestamp: 1701851743
C# Program to Get the Unix Timestamp Using the TimeSpan
Struct Methods
One approach involves leveraging the TimeSpan
structure and its TotalSeconds
property. This method offers a straightforward and efficient way to calculate the elapsed time between a specific date and time and the Unix epoch.
Let’s explore a complete example demonstrating how to obtain the Unix timestamp in C# using TimeSpan
and the TotalSeconds
method.
Code Input:
using System;
class Program {
static void Main() {
// Get the current UTC time
DateTime currentTime = DateTime.UtcNow;
// Calculate the Unix timestamp using TimeSpan
TimeSpan elapsedTime = currentTime - new DateTime(1970, 1, 1, 0, 0, 0, DateTimeKind.Utc);
long unixTimestamp = (long)elapsedTime.TotalSeconds;
// Display the result
Console.WriteLine("Unix Timestamp: " + unixTimestamp);
}
}
We start by obtaining the current UTC time using DateTime.UtcNow
. It’s crucial to work with UTC time to ensure consistency and avoid issues related to time zone differences or daylight saving time changes.
Following that, we calculate the elapsed time by subtracting the Unix epoch (January 1, 1970) from the current UTC time. This subtraction operation results in a TimeSpan
object representing the time difference between the two points in time.
To convert this TimeSpan
into seconds, we access the TotalSeconds
property. This property provides the total number of seconds represented by the TimeSpan
, effectively giving us the Unix timestamp.
Finally, we display the Unix timestamp to the console using Console.WriteLine()
.
Code Output:
Unix Timestamp: 1701851743
Conclusion
In this exploration of Unix timestamp acquisition methods in C#, we’ve delved into the elegance of code using DateTime.Now
and Subtract
, the conciseness of DateTimeOffset.Now.ToUnixTimeSeconds
, and the simplicity of TimeSpan
and TotalSeconds
.
Each method offers a unique perspective, providing developers with options tailored to specific scenarios. Whether aiming for clarity, precision, or concise code, these techniques showcase the flexibility inherent in C# for managing time-related computations.
As developers embrace these methodologies, they enhance their ability to work seamlessly with timestamps, a skill indispensable in diverse programming landscapes.