How to Calculate the Difference Between Two Dates in C#
-
Calculate Difference Between Two Dates in C# Using
-
Operator -
Calculate Difference Between Two Dates in C# Using
DateTime.Substract
Method
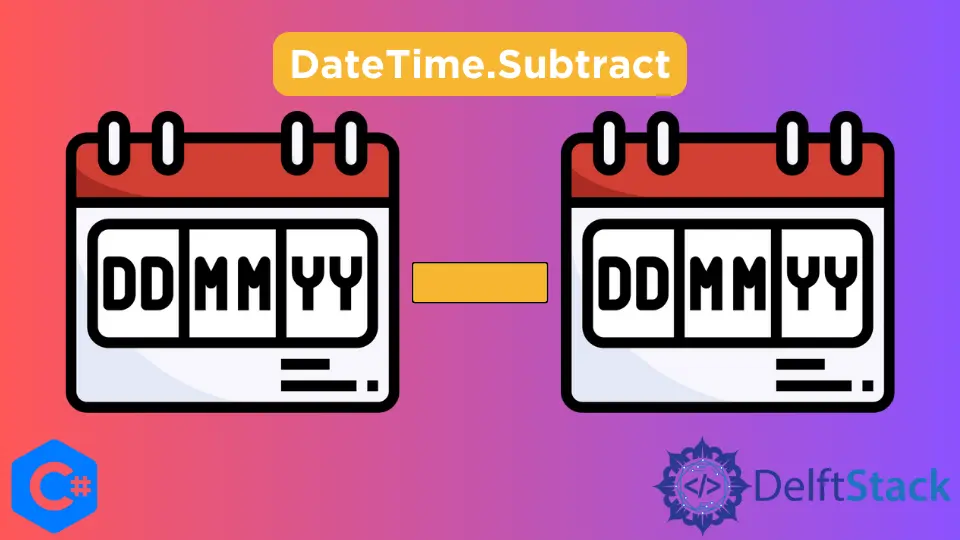
In this article, we will explore various methods of calculating the difference between two dates in C#. We will use DateTime
structure for the representation of Date and TimeSpan
structure to store the result.
We will use the following definition of date, specifying an instant of date using DateTime
constructor.
DateTime sample_date = new DateTime(2010, 6, 14);
As you might notice, the elements of the date in the declaration include the year, month, and day.
Now, Let’s look at the various methods to calculate the difference between two days in terms of the number of days.
Calculate Difference Between Two Dates in C# Using -
Operator
The simplest of the solution is subtracting the two dates using -
operator. Since the objects are of the same data type DateTime
, they can be directly subtracted. The following code depicts this method.
using System;
public class Sample {
public static void Main() {
DateTime date_1 = new DateTime(2019, 02, 12);
DateTime date_2 = new DateTime(2020, 04, 10);
/*Subtraction (minus) of same data types yields difference in days*/
Console.WriteLine("Difference in days: " + (date_2 - date_1).Days);
}
}
Output:
Difference in days: 423
Calculate Difference Between Two Dates in C# Using DateTime.Substract
Method
The DateTime.Subtract
method is another efficient way to achieve this. It returns the difference between two dates and the result can be stored in TimeSpan
data type.
using System;
public class Sample {
public static void Main() {
DateTime date_1 = new DateTime(2019, 02, 12);
DateTime date_2 = new DateTime(2020, 04, 10);
TimeSpan Diff_dates = date_2.Subtract(date_1);
Console.WriteLine("Difference in days: " + Diff_dates.Days);
}
}
Output:
Difference in days: 423
DateTime
structure can also be used to specify the time of day along with the date. In this case, while using DateTime.Subtract
method, ensure the dates are in the same time zones. Otherwise, the result will include the difference between timezones.
Moreover, using the dual set of properties of TimeSpan
class we can also extract more information if needed. Like in the sample code below, we have used Total
prefix - TotalDays
, to obtain the result in fractional values.
using System;
public class Sample {
public static void Main() {
/*Definations include time of day */
DateTime date_1 = new DateTime(2019, 02, 12, 8, 0, 0);
DateTime date_2 = new DateTime(2020, 04, 10, 12, 0, 0);
TimeSpan Diff_dates = date_2.Subtract(date_1);
Console.WriteLine("Difference in Days = " + Diff_dates.Days);
Console.WriteLine("Exact timespan in Days = " + Diff_dates.TotalDays);
}
}
Output:
Difference in Days = 423
Exact timespan in Days = 423.166666666667
While finalizing the method appropriate for your usage, note that the -
operator method doesn’t take into account the timezone factor of dates. So, if the dates you are using specify timezones, DateTime.Subtract
method becomes the obvious choice.