The goto Statement in C#
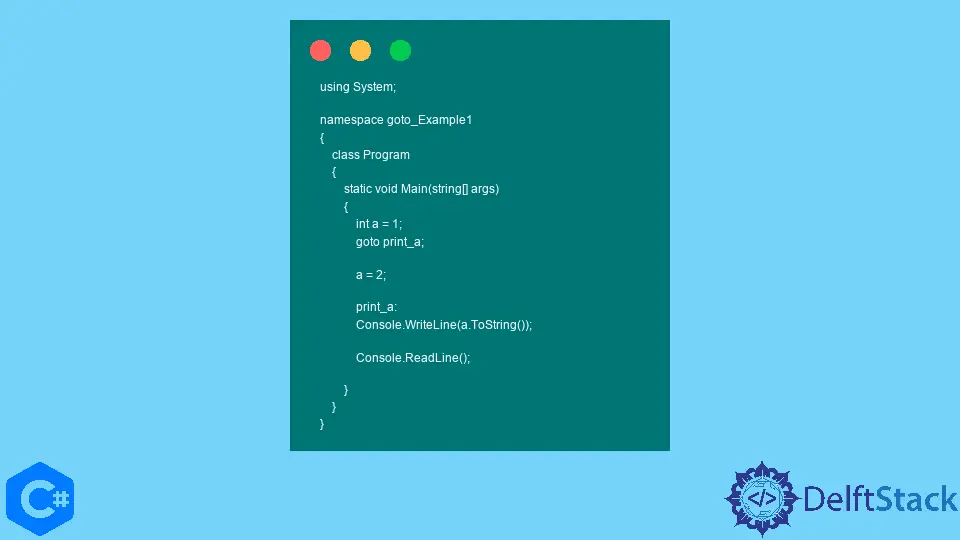
This tutorial will demonstrate how to use the goto
syntax in C# and provide some examples of practical use in your code.
goto
is an unconditional jump statement that the program will automatically go to a new part of the code once encountered. To use goto
, you must have both a statement marked by a label and an instance calling to the label.
To create a label, add its name with a colon before the statement you want to be run when the label is called.
Example:
using System;
namespace goto_Example1 {
class Program {
static void Main(string[] args) {
int a = 1;
goto print_a;
a = 2;
print_a:
Console.WriteLine(a.ToString());
Console.ReadLine();
}
}
}
We initialize the integer variable a
to equal one in this example. Since we immediately call goto
to jump to the statement print_a
, a
is never set to equal two. Therefore, when we print the value of a
to the console, what’s published is 1 and not 2.
Output:
1
When to Use goto
in C#
However, goto
is not as commonly used now, as it has been criticized for worsening the readability of code because the flow of logic is not as clear if it requires you to jump to a completely different section.
However, there are still some instances when goto
can be beneficial and improve readability. For example, it can escape nested loops and switch
statements.
Example:
using System;
namespace goto_Example2 {
class Program {
static void Main(string[] args) {
// Intialize the integer variable a
int a = 2;
// Run the function test input
TestInput(a);
// Change the value of a and see how it affects the output from TestInput()
a = 1;
TestInput(a);
a = 3;
TestInput(a);
Console.ReadLine();
}
static void TestInput(int input) {
// In this example function, we only want to accept either 1 or 2 as values.
// If we accept the value 1, we want to add to it and then run case 2;
// Print the original value
Console.WriteLine("Input Being Tested: " + input.ToString());
switch (input) {
case 1:
input++;
// If goto isn't called, the input value will not be modified, and its final value would
// have been unchanged
goto case 2;
case 2:
input = input / 2;
break;
default:
break;
}
Console.WriteLine("Final Value: " + input.ToString() + "\n");
}
}
}
In the example above, we created a sample function to do different things depending on the value passed. Three cases are being considered. The first is if the value is equal to 1. If this is the case, we will add to the input value and then proceed to case 2 using the goto
function. The input value would have remained unchanged had goto
not been called.
In case 2, we divide the input by 2. Lastly, any other value passed will fall under the default case and not be modified at all. Finally, the final value is printed and shows that cases 1 and 2 will result in the same absolute value. Because of goto
, the case can be applied even if it did not initially meet the case specifications by jumping to its statement.
Output:
Input Being Tested: 2
Final Value: 1
Input Being Tested: 1
Final Value: 1
Input Being Tested: 3
Final Value: 3