C# 中的 goto 語句
Fil Zjazel Romaeus Villegas
2023年10月12日
Csharp
Csharp Statement
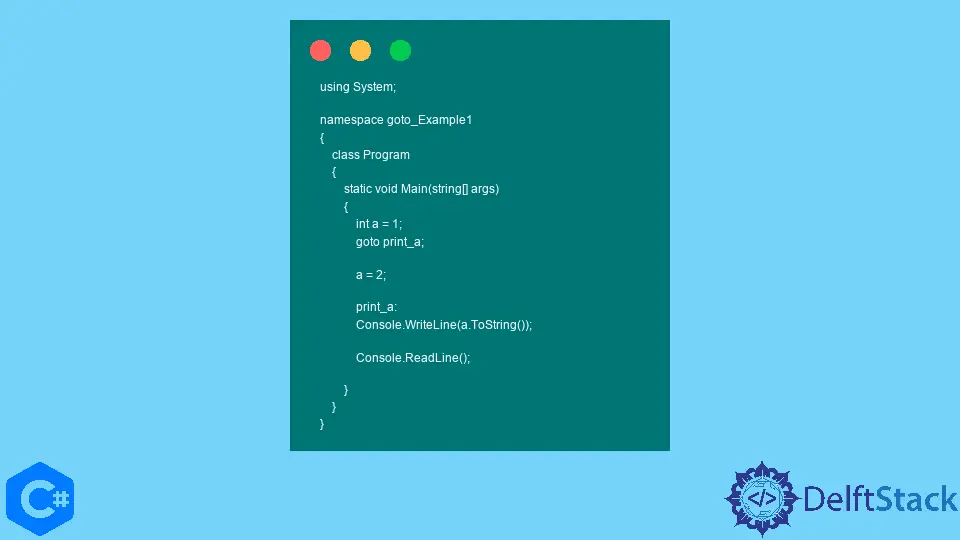
本教程將演示如何在 C# 中使用 goto
語法,並提供一些程式碼中的實際使用示例。
goto
是一個無條件跳轉語句,一旦遇到程式將自動轉到程式碼的新部分。要使用 goto
,你必須同時擁有一個由標籤標記的語句和一個呼叫該標籤的例項。
要建立標籤,請在呼叫標籤時要執行的語句之前新增其名稱和冒號。
例子:
using System;
namespace goto_Example1 {
class Program {
static void Main(string[] args) {
int a = 1;
goto print_a;
a = 2;
print_a:
Console.WriteLine(a.ToString());
Console.ReadLine();
}
}
}
在本例中,我們將整數變數 a
初始化為等於 1。由於我們立即呼叫 goto
跳轉到語句 print_a
,所以 a
永遠不會設定為等於 2。因此,當我們將 a
的值列印到控制檯時,釋出的是 1 而不是 2。
輸出:
1
何時在 C#
中使用 goto
然而,goto
現在並不常用,因為它被批評為惡化程式碼的可讀性,因為如果它需要你跳轉到完全不同的部分,邏輯流程就不那麼清晰了。
然而,仍有一些情況下 goto
可以帶來好處並提高可讀性。例如,它可以轉義巢狀迴圈和 switch
語句。
例子:
using System;
namespace goto_Example2 {
class Program {
static void Main(string[] args) {
// Intialize the integer variable a
int a = 2;
// Run the function test input
TestInput(a);
// Change the value of a and see how it affects the output from TestInput()
a = 1;
TestInput(a);
a = 3;
TestInput(a);
Console.ReadLine();
}
static void TestInput(int input) {
// In this example function, we only want to accept either 1 or 2 as values.
// If we accept the value 1, we want to add to it and then run case 2;
// Print the original value
Console.WriteLine("Input Being Tested: " + input.ToString());
switch (input) {
case 1:
input++;
// If goto isn't called, the input value will not be modified, and its final value would
// have been unchanged
goto case 2;
case 2:
input = input / 2;
break;
default:
break;
}
Console.WriteLine("Final Value: " + input.ToString() + "\n");
}
}
}
在上面的示例中,我們建立了一個示例函式來根據傳遞的值執行不同的操作。正在考慮三個案例。第一個是值是否等於 1。如果是這種情況,我們將新增到輸入值,然後使用 goto
函式繼續到情況 2。如果沒有呼叫 goto
,輸入值將保持不變。
在情況 2 中,我們將輸入除以 2。最後,傳遞的任何其他值都將屬於預設情況,根本不會被修改。最後,列印出最終值並顯示案例 1 和 2 將產生相同的絕對值。由於 goto
,即使最初不符合案例規範,也可以通過跳轉到其語句來應用案例。
輸出:
Input Being Tested: 2
Final Value: 1
Input Being Tested: 1
Final Value: 1
Input Being Tested: 3
Final Value: 3
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe