The if Statement With Multiple Conditions in C#
- Using Operators in C#
-
Use the
if
Statement With Multiple Logical Conditions - Use the Ternary Conditional Operator With Multiple Conditions in C#
-
Use the
switch
Statement With Multiple Conditions in C# - Conclusion
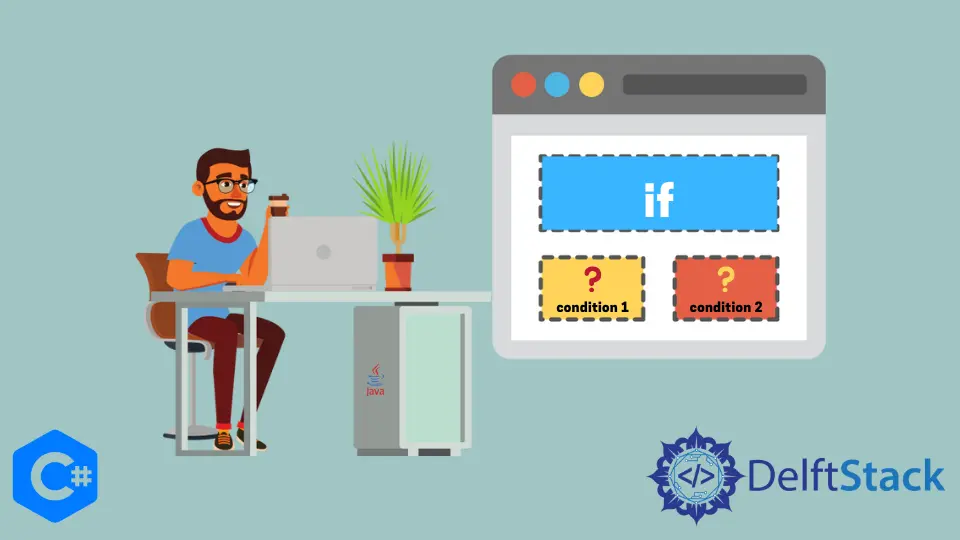
Conditional statements and operators are fundamental elements in programming that allow for decision-making and control of program flow based on certain conditions. In C#, two primary conditional branching statements are used: the if
statement and the switch
statement.
This article will introduce the use of the if
statement with multiple conditions to return a statement in C#. Further discussion is available via this reference.
Using Operators in C#
Operators in C# are symbols or keywords used to perform various operations on variables and values. They can be categorized into four main types: Arithmetic, Assignment, Comparison, and Logical operators.
Comparison operators allow for the comparison of two values in C#. Here are the commonly used comparison operators and their usage:
< |
Less than | a < b |
> |
Greater than | a > b |
== |
Equal to | a == b |
<= |
Less than or equal to | a <= b |
>= |
Greater than or equal to | a >= b |
!= |
Not Equal to | a != b |
On the other hand, logical operators are used to perform logical operations on Boolean values. There are three primary logical operators:
- Logical
AND
(&&
): Returns true if both compared statements are true. - Logical
OR
(||
): Returns true if at least one of the compared statements is true. - Logical
NOT
(!
): Negates the result of a comparison. Returns true if the result is false and vice versa.
These operators can be used independently or in combination to create complex logical conditions.
Use the if
Statement With Multiple Logical Conditions
The if
statement is a crucial control flow statement in C# that allows for conditional execution of code blocks. When using multiple logical conditions with an if
statement, you can create intricate decision-making processes.
Here’s a code snippet demonstrating the use of the if
statement with multiple logical conditions:
using System;
class Program {
public static void Main() {
string a = "Abdul", b = "Salawu", c = "Stranger", A2 = "Age";
bool checkbox = true;
string columnname = "Abdullahi Salawudeen";
if (columnname != a && columnname != b && columnname != c && (checkbox || columnname != A2)) {
Console.WriteLine(
"Columnname is neither equal to a nor b nor c nor A2, but the checkbox is checked");
} else {
Console.WriteLine("Columnname is unknown and checkbox is false");
}
}
}
Output:
Columnname is neither equal to a nor b nor c nor A2, but the checkbox is checked
In this example, we check if columnname
is not equal to a
, b
, c
, and A2
and if the checkbox is checked. Based on these conditions, we output appropriate messages.
Using Nested if
Statements in C# to Handle Multiple Conditions
In programming, making decisions and executing specific actions based on certain conditions is a fundamental aspect. One of the primary control structures to achieve this in C# is the if
statement.
Sometimes, you need to evaluate multiple conditions before deciding what action to take. This is where nested if
statements become useful.
Nested if
statements involve placing an if
statement inside another if
statement. This allows for a more complex decision-making process based on multiple conditions.
if (condition1) {
if (condition2) {
// Code to execute if both condition1 and condition2 are true
}
}
In this structure, the code inside the inner if
block will only execute if both condition1
and condition2
are true.
Let’s go through a practical example to demonstrate the usage of nested if
statements.
using System;
class Program {
static void Main(string[] args) {
int age = 25;
bool isStudent = true;
if (age >= 18) {
if (isStudent) {
Console.WriteLine("You are a student and of legal age.");
} else {
Console.WriteLine("You are of legal age.");
}
} else {
Console.WriteLine("You are underage.");
}
}
}
Output:
You are a student and of legal age.
In this example, we have nested if
statements. The outer if
checks if the age
is greater than or equal to 18.
If this is true, it evaluates the inner if
statement, checking if the person is a student (isStudent
is true). Depending on the conditions, it prints the appropriate message.
Using nested if
statements provides a structured approach to handling multiple conditions. It allows you to create a hierarchy of conditions and actions based on those conditions.
However, excessive nesting can lead to code that is hard to read and understand. It’s essential to strike a balance and consider other control structures like switch
statements or refactoring the code to make it more readable and maintainable.
Use the Ternary Conditional Operator With Multiple Conditions in C#
The ternary conditional operator (? :
) is a concise way to write an if-else
statement and can be used to evaluate conditions and return different values or execute different statements based on those conditions.
The syntax of the ternary conditional operator is as follows:
condition? expressionIfTrue : expressionIfFalse;
Here’s a brief explanation of the syntax above:
condition
: The expression to be evaluated as true or false.expressionIfTrue
: The value or expression to be returned if the condition is true.expressionIfFalse
: The value or expression to be returned if the condition is false.
Here’s a code example using the ternary operator with multiple logical conditions:
using System;
class Program {
public static void Main() {
string a = "Abdul", b = "Salawu", c = "Stranger", A2 = "Age";
bool checkbox = false;
string columnname = A2;
string result =
(columnname != a && columnname != b && columnname != c && (checkbox || columnname != A2))
? "Columnname is neither equal to a nor b nor c nor A2 nor is the checkbox true"
: "Columnname is unknown and checkbox is false";
Console.WriteLine(result);
}
}
Output:
Columnname is unknown and checkbox is false
Here, several variables are declared and initialized:
a
,b
,c
,A2
: Strings with specific values.checkbox
: A boolean variable initialized tofalse
.columnname
: A string variable assigned the value ofA2
.
Following this, we used a ternary conditional operator to assign a value to the result
variable based on certain conditions. Let’s break down the conditions:
- If
columnname
is not equal toa
,b
,c
, andA2
, and eithercheckbox
istrue
orcolumnname
is not equal toA2
, then setresult
to a specific message. - Otherwise, set
result
to another specific message.
Finally, the program prints the value of the result
variable to the console.
In a nutshell, this program determines a message based on conditions related to the values of columnname
, checkbox
, and specific strings (a
, b
, c
, A2
). Depending on these conditions, it outputs different messages to the console.
Use the switch
Statement With Multiple Conditions in C#
The switch
statement is also a control flow construct that facilitates multiple branches of execution based on the value of an expression. It’s an efficient alternative to a series of if
statements when you need to compare a single value to multiple possible cases.
Here’s the basic syntax of a switch
statement:
switch (expression) {
case value1:
// code to execute for value1
break;
case value2:
// code to execute for value2
break;
// ...
default:
// code to execute if no cases match
break;
}
The expression
is evaluated, and the corresponding case
block is executed based on the matched value. The break
statement is crucial to exit the switch
block after executing the appropriate case.
When dealing with multiple conditions, each case
block in a switch
statement represents a different condition or value to evaluate. This allows for a cleaner and more organized approach to handling diverse scenarios.
Let’s see a practical example to illustrate the switch
statement in handling multiple conditions.
using System;
class Program {
static void Main(string[] args) {
char grade = 'B';
switch (grade) {
case 'A':
Console.WriteLine("Excellent!");
break;
case 'B':
Console.WriteLine("Good job!");
break;
case 'C':
Console.WriteLine("Satisfactory.");
break;
default:
Console.WriteLine("Invalid grade.");
break;
}
}
}
Output:
Good job!
In this example, we use a switch
statement to evaluate the grade
variable and provide appropriate feedback based on the grade achieved.
Conclusion
This article has provided a comprehensive overview of conditional statements and operators in C#. We have introduced the if
statement with multiple conditions, demonstrating its usage through code examples.
Additionally, we have discussed using nested if
statements for handling complex conditions, illustrating their application with practical examples. The ternary conditional operator and the switch
statement were also introduced as efficient alternatives for managing multiple conditions, showcasing their syntax and usage through code illustrations.