How to Declare a Global Variable in C#
-
Declare a Global Variable by Defining a
public static
Variable Inside a Public Class inC#
-
Declare a Global Variable by Defining a
public static
Property Inside a Public Class inC#
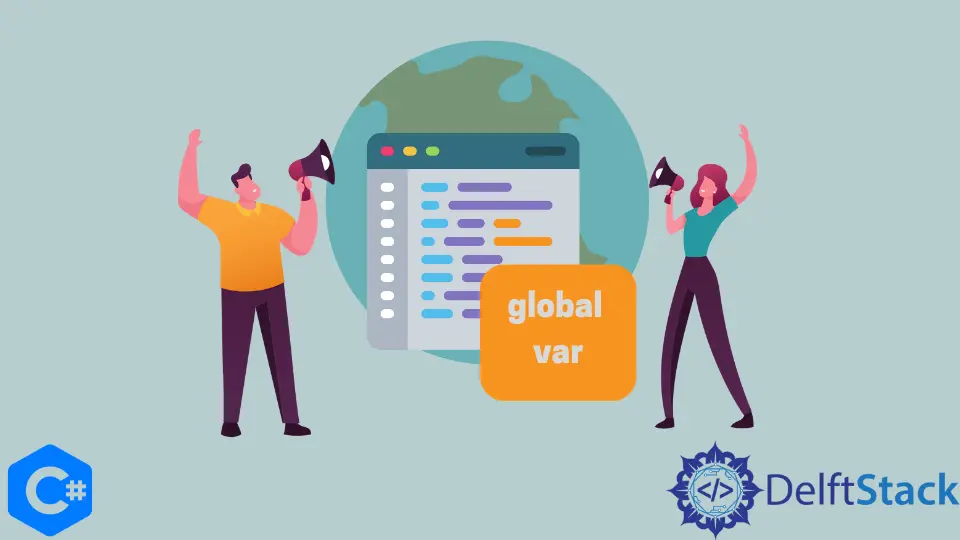
In this tutorial, we will discuss methods to declare a global variable in C#.
Declare a Global Variable by Defining a public static
Variable Inside a Public Class in C#
A global variable is a variable that can be accessed in all the classes throughout our program. Unfortunately, there is no built-in method or keyword to achieve this goal. So, we have to rely on user-defined approaches for this. We can declare a public static
variable inside a public
class to use it as a global variable in C#. The following code example shows us how to declare a global variable with a public
class in C#.
using System;
namespace create_global_variable {
public static class Global { public static string name; }
class Program {
static void Main(string[] args) {
Global.name = "Delft Stack";
Console.WriteLine(Global.name);
}
}
}
Output:
Delft Stack
In the above code, we declared a public static
variable name
. The public
keyword indicates that name
can be accessed outside the class and the static
keyword indicates that we don’t have to create a class object to access it. The variable name
can be accessed anywhere inside the create_global_variable
namespace using Global.name
. If we want to access the Global.name
variable outside the create_global_variable
namespace, we have to define the Global
class outside the create_global_variable
namespace.
Declare a Global Variable by Defining a public static
Property Inside a Public Class in C#
If you don’t want to declare a public
variable inside a class, you can use a property
instead. The following code example shows us how to use the public static
property to declare a global variable in C#.
using System;
namespace create_global_variable {
public class Global {
public static string name;
public static String Name { get; set; }
}
class Program {
static void Main(string[] args) {
Global.Name = "Delft Stack";
Console.WriteLine(Global.Name);
}
}
}
Output:
Delft Stack
In the above code, we declared a public static
property Name
. The public
keyword indicates that Name
can be accessed outside the class and the static
keyword indicates that we don’t have to create a class object to access it. The property Name
can be accessed anywhere inside the create_global_variable
namespace by using Global.Name
. If we want to access the Global.Name
property outside the create_global_variable
namespace, we have to define the Global
class outside the create_global_variable
namespace.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn