How to Create Rock Paper Scissors Game in C#
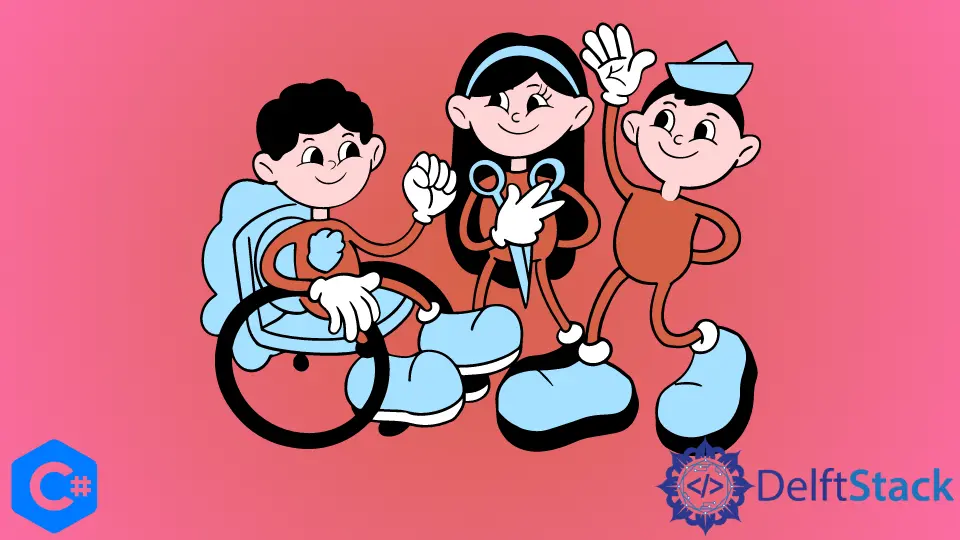
This article will talk about the process of developing a game of Rock, Paper, and Scissors with C#. We will use the Visual Studio to create a console App, name, and project.
Create Rock Paper Scissors Game in C#
To make this game, we shall follow these steps:
-
Take the input from the user.
-
Randomly generate the output.
-
Compare all the possible choices.
-
Declare the winner.
-
Restart the game.
This program’s operation will include a variety of functions and methods. Once the user’s input has been received, the system will compare it to the input produced by the system.
This system’s produced input will be random; thus, it might supply any of the three options: rock, paper, or scissors. It will do conditional rendering once it receives both inputs.
The conditional rendering will serve as the foundation to determine whether a winner will be announced or whether there will be no winner, and a draw will be held.
using System;
namespace Hello_World {
class Program {
static void Main(string[] args) {}
}
}
These lines will be at the start of the code and will remain as the normal program flow starts.
string ui, si;
int r;
Console.Write("What do you choose?\n\n Rock/ Paper/ Scissors? ");
ui = Console.ReadLine();
Random rnd = new Random();
r = rnd.Next(1, 4);
si
and ui
are the two variables for user input and system input as a string, so the user should type complete spellings of their choice. The r
variable is the integer type variable made for the random number the computer will generate for the choice.
The Console.Write("What do you choose?\n\n Rock/ Paper/ Scissors? ")
statement is written on the screen and asks the user to enter their choice. The ui = Console.ReadLine()
statement takes the input from the user and stores it into the ui
variable.
The Random rnd = new Random()
statement creates the variable rnd
for the random number to be used afterward to generate the random numbers. The r = rnd.Next(1, 4)
statement generates the random in the range 1 to 3 and stores it into the r
variable.
switch (r) {
case 1:
si = "Rock";
Console.WriteLine("Computer chose Rock");
if (ui == "Rock" || ui == "rock") {
Console.WriteLine("\nIt is a draw");
} else if (ui == "Paper" || ui == "paper") {
Console.WriteLine("\nYou Win!");
} else {
Console.WriteLine("\nYou Lose!");
}
break;
}
In switch()
, we consider the options if the r
variable holds any number from 1 to 4. In case 1, we check if the random number is one, and if so, we set the system input si
variable to rock.
After that, we write on the console using Console.WriteLine("Computer chose Rock")
statement that prints on the screen that the computer chose rock. Then we use if
conditions to see what the user entered for their choice.
The if (ui == "Rock"||ui == "rock")
statement checks if the user also entered rock, then it is a draw and it will be printed on the screen by Console.WriteLine("\nIt is a draw")
. If not, then we’ll check by else if(ui == "Paper"||ui == "paper")
to see if its not rock, then is it paper that the user entered?
If yes
, then the user won, which will be printed by Console.WriteLine("\nYou Win!");
.
If the user did not enter both options we checked, it is obvious that the user entered scissors, so the user lost. So, we used else
at the end, which will print You Lose!
.
Lastly, the break
statement is used to exit the switch()
.
case 2:
si = "Paper";
Console.WriteLine("Computer chose Paper");
if (ui == "Rock" || ui == "rock") {
Console.WriteLine("\nYou Lose!");
} else if (ui == "Paper" || ui == "paper") {
Console.WriteLine("It is a draw");
} else {
Console.WriteLine("You win");
}
break;
In case 2, we check that if the random number is 2, then we set the system input si
variable to paper. After that, we write on the console using Console.WriteLine("Computer chose Paper")
, which prints on the screen that the computer chose paper.
Then, we used if
conditions to see what the user entered for his/her choice. The if (ui == "Rock"||ui == "rock")
statement checks if the user also entered rock, then the user will lose, and it will be printed on the screen by Console.WriteLine("\nYou Lose!");
.
If not, then we check by else if(ui == "Paper"||ui == "paper")
to see if its not rock, then is it paper that user entered? If yes
, then it is a draw. And it will be printed by Console.WriteLine("It is a draw");
.
If the user did not enter both options we had checked, it is obvious that the user entered scissors, so the user wins the game. So, we used else
at the end, which will be printed by Console.WriteLine("You win");
.
The break
statement is used to exit out of switch()
.
case 3:
si = "Scissors";
Console.WriteLine("Computer chose Scissors");
if (ui == "Rock" || ui == "rock") {
Console.WriteLine("\nYou Win!");
} else if (ui == "Paper" || ui == "paper") {
Console.WriteLine("You Lose");
} else {
Console.WriteLine("It is a draw");
}
break;
In case 3, we check that if the random number is 3, then we set the system input si
variable to scissors. After that, we write on the console by Console.WriteLine("Computer chose Scissors")
line that prints on the screen that the computer chose scissors.
Then we use if
conditions to see what the user entered for their choice. The if (ui == "Rock"||ui == "rock")
line checks if the user also entered rock, then the user will win, and it will be printed on the screen by the Console.WriteLine("\nYou Win!");
line.
If not then we check by else if(ui == "Paper"||ui == "paper")
line to see if its not rock, then is it paper that user entered? If yes
, then the user will lose the game. And it will be printed by Console.WriteLine("You Lose");
line.
If the user did not enter both options we had checked, it is obvious that the user entered scissors, so the game is a draw. So we used else
at the end, which will be printed by Console.WriteLine("It is a draw");
.
default:
Console.WriteLine("invalid entry!");
break;
At the end of the switch
scope, we also used default:
, a kind of default case that runs when no case is matched. This is a safety precaution if the r
variable has any other value than 1 to 3.
Then we will print on the screen invalid entry!
using Console.WriteLine("invalid entry!");
.
If you want, you may not create and use the si
variable in your code because the decisions are made with the random number variable r
.
If you want the user to type yes
or no
to play the game again, then you should use the code as follows.
using System;
namespace Hello_World {
class Program {
static void Main(string[] args) {
bool play = true;
string q;
while (play) {
// all the above code of the game goes here
Console.WriteLine("\nDo you want to play again? ");
q = Console.ReadLine();
if (q == "yes" || q == "Yes") {
continue;
} else if (q == "no" || q == "No") {
play = false;
} else {
Console.WriteLine("invalid entry!\nQuiting...!");
play = false;
}
}
}
}
}
We created a play
variable of bool type, which is used in the while
loop. Initially, it is set to true, so the loop will infinitely run until the play
variable is set to false.
You can place all your game code in the while
loop, and after that, you can ask the user to play again or not using Console.WriteLine("\nDo you want to play again? ");
. Whatever the user will enter will be stored in the q
variable using the line q= Console.ReadLine();
.
After that, we will again use the if
conditions to see what was entered by the user.
The if(q=="yes"||q=="Yes")
statement checks the user’s input, if it is equivalent to yes or no. If the user has entered yes
, then the continue
statement is used to skip the rest of the loop statements and start the loop from the beginning.
If the user has not entered yes
but has entered no
instead, then the condition else if(q=="no"||q=="No")
becomes true, and the play
variable is set to false by the line play=false;
, the loop will stop, and the program will terminate.
And if the user has entered anything else except yes
or no
, then invalid entry!
will be printed on the screen by Console.WriteLine("invalid entry!\nQuiting...!");
. And play
will again be false, and the program will terminate.
Full Source Code:
using System;
namespace Hello_World {
class Program {
static void Main(string[] args) {
bool play = true;
string q;
while (play) {
string ui, si;
int r;
Console.Write("What do you choose?\n\n Rock/ Paper/ Scissors? ");
ui = Console.ReadLine();
Random rnd = new Random();
r = rnd.Next(1, 4);
switch (r) {
case 1:
si = "Rock";
Console.WriteLine("Computer chose Rock");
if (ui == "Rock" || ui == "rock") {
Console.WriteLine("\nIt is a draw");
} else if (ui == "Paper" || ui == "paper") {
Console.WriteLine("\nYou Win!");
} else {
Console.WriteLine("\nYou Lose!");
}
break;
case 2:
si = "Paper";
Console.WriteLine("Computer chose Paper");
if (ui == "Rock" || ui == "rock") {
Console.WriteLine("\nYou Lose!");
} else if (ui == "Paper" || ui == "paper") {
Console.WriteLine("It is a draw");
} else {
Console.WriteLine("You win");
}
break;
case 3:
si = "Scissors";
Console.WriteLine("Computer chose Scissors");
if (ui == "Rock" || ui == "rock") {
Console.WriteLine("\nYou Win!");
} else if (ui == "Paper" || ui == "paper") {
Console.WriteLine("You Lose");
} else {
Console.WriteLine("It is a draw");
}
break;
default:
Console.WriteLine("invalid entry!");
break;
}
Console.WriteLine("\nDo you want to play again? ");
q = Console.ReadLine();
if (q == "yes" || q == "Yes") {
continue;
} else if (q == "no" || q == "No") {
play = false;
} else {
Console.WriteLine("invalid entry!\nQuiting...!");
play = false;
}
}
}
}
}
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn