在 C# 中建立石頭剪刀布遊戲
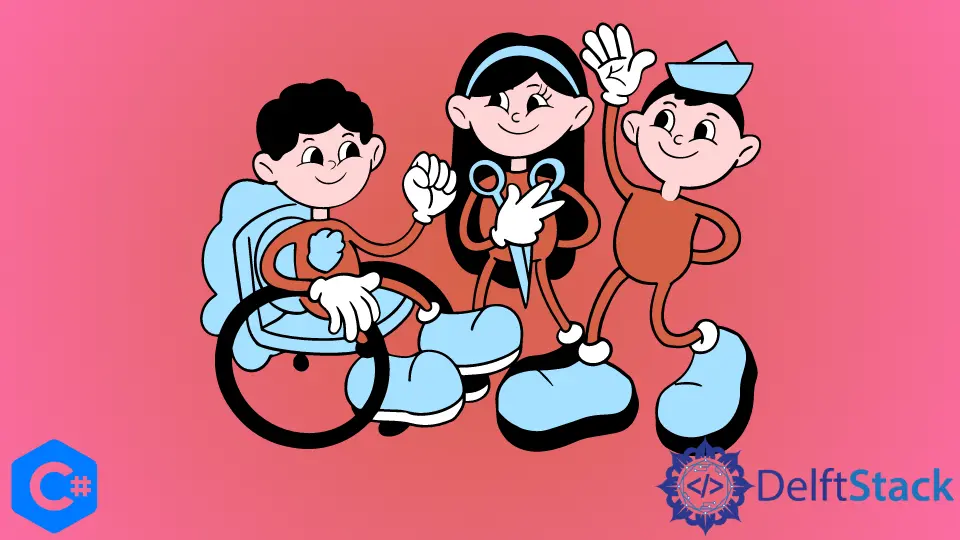
本文將討論使用 C# 開發石頭剪刀布遊戲的過程。我們將使用 Visual Studio 建立控制檯應用程式、名稱和專案。
在 C#
中建立石頭剪刀布遊戲
為了製作這個遊戲,我們將遵循以下步驟:
-
接受使用者的輸入。
-
隨機生成輸出。
-
比較所有可能的選擇。
-
宣佈獲勝者。
-
重新開始遊戲。
該程式的執行將包括多種功能和方法。一旦接收到使用者的輸入,系統會將其與系統產生的輸入進行比較。
該系統產生的輸入將是隨機的;因此,它可能提供三個選項中的任何一個:石頭、布或剪刀。一旦接收到兩個輸入,它將進行條件渲染。
條件渲染將作為決定是否宣佈獲勝者或沒有獲勝者的基礎,並進行抽籤。
using System;
namespace Hello_World {
class Program {
static void Main(string[] args) {}
}
}
這些行將位於程式碼的開頭,並將在正常程式流程開始時保留。
string ui, si;
int r;
Console.Write("What do you choose?\n\n Rock/ Paper/ Scissors? ");
ui = Console.ReadLine();
Random rnd = new Random();
r = rnd.Next(1, 4);
si
和 ui
是使用者輸入和系統輸入作為字串的兩個變數,因此使用者應該輸入他們選擇的完整拼寫。r
變數是為計算機將生成的隨機數用於選擇的整數型別變數。
Console.Write("What do you choose?\n\n Rock/ Paper/ Scissors? ")
語句寫在螢幕上並要求使用者輸入他們的選擇。ui = Console.ReadLine()
語句從使用者那裡獲取輸入並將其儲存到 ui
變數中。
Random rnd = new Random()
語句為隨機數建立變數 rnd
以用於隨後生成隨機數。r = rnd.Next(1, 4)
語句生成 1 到 3 範圍內的隨機數並將其儲存到 r
變數中。
switch (r) {
case 1:
si = "Rock";
Console.WriteLine("Computer chose Rock");
if (ui == "Rock" || ui == "rock") {
Console.WriteLine("\nIt is a draw");
} else if (ui == "Paper" || ui == "paper") {
Console.WriteLine("\nYou Win!");
} else {
Console.WriteLine("\nYou Lose!");
}
break;
}
在 switch()
中,如果 r
變數包含 1 到 4 之間的任何數字,我們會考慮選項。在情況 1 中,我們檢查隨機數是否為 1,如果是,我們設定系統輸入 si
變搖滾。
之後,我們使用 Console.WriteLine("Computer choose Rock")
語句在控制檯上寫入,該語句會在螢幕上列印計算機選擇 Rock。然後我們使用 if
條件來檢視使用者輸入的內容供他們選擇。
if (ui == "Rock"||ui == "rock")
語句檢查使用者是否也輸入了 rock,那麼這是一個平局,它將通過 Console.WriteLine("\nIt is a draw")
。如果不是,那麼我們將通過 else if(ui == "Paper"||ui == "paper")
來檢查,如果它不是石頭,那麼使用者輸入的是布?
如果 yes
,則使用者贏了,這將由 Console.WriteLine("\nYou Win!");
列印。
如果使用者沒有輸入我們檢查的兩個選項,很明顯使用者輸入了剪刀,所以使用者輸了。所以,我們最後使用了 else
,它會列印 You Lose!
。
最後,break
語句用於退出 switch()
。
case 2:
si = "Paper";
Console.WriteLine("Computer chose Paper");
if (ui == "Rock" || ui == "rock") {
Console.WriteLine("\nYou Lose!");
} else if (ui == "Paper" || ui == "paper") {
Console.WriteLine("It is a draw");
} else {
Console.WriteLine("You win");
}
break;
在情況 2 中,我們檢查隨機數是否為 2,然後我們將系統輸入 si
變數設定為布。之後,我們使用 Console.WriteLine("Computer choose Paper")
在控制檯上書寫,它會在螢幕上列印計算機選擇布的資訊。
然後,我們使用 if
條件來檢視使用者輸入的內容供他/她選擇。if (ui == "Rock"||ui == "rock")
語句檢查使用者是否也輸入了 rock,則使用者將輸,並通過 Console.WriteLine(" 列印在螢幕上\n 你鬆了!");
。
如果不是,那麼我們通過 else if(ui == "Paper"||ui == "paper")
檢查它是否不搖滾,那麼使用者輸入的是布嗎?如果 yes
,則為平局。它將由 Console.WriteLine("It is a draw");
列印。
如果使用者沒有輸入我們檢查過的兩個選項,很明顯使用者輸入了剪刀,因此使用者贏得了遊戲。所以,我們最後使用了 else
,它將由 Console.WriteLine("You win");
列印。
break
語句用於退出 switch()
。
case 3:
si = "Scissors";
Console.WriteLine("Computer chose Scissors");
if (ui == "Rock" || ui == "rock") {
Console.WriteLine("\nYou Win!");
} else if (ui == "Paper" || ui == "paper") {
Console.WriteLine("You Lose");
} else {
Console.WriteLine("It is a draw");
}
break;
在案例 3 中,我們檢查隨機數是否為 3,然後我們將系統輸入 si
變數設定為剪刀。之後,我們在控制檯上通過 Console.WriteLine("Computer choose Scissors")
行在螢幕上列印計算機選擇了剪刀。
然後我們使用 if
條件來檢視使用者輸入的內容供他們選擇。if (ui == "Rock"||ui == "rock")
行檢查使用者是否也輸入了 rock,然後使用者將獲勝,並由 Console.WriteLine("\nYou Win!");
一行列印到螢幕上。
如果不是,那麼我們通過 else if(ui == "Paper"||ui == "paper")
行檢查,如果它不是石頭,那麼使用者輸入的是布嗎?如果 yes
,則使用者將輸掉遊戲。它將由 Console.WriteLine("You Lose");
列印出來。
如果使用者沒有輸入我們檢查的兩個選項,很明顯使用者輸入了剪刀,所以遊戲是平局。所以我們最後使用了 else
,它會被 Console.WriteLine("It is a draw");
列印出來。
default:
Console.WriteLine("invalid entry!");
break;
在 switch
作用域的末尾,我們還使用了 default:
,這是一種在沒有匹配大小寫時執行的預設情況。如果 r
變數的值不是 1 到 3,這是一種安全預防措施。
然後我們將用 Console.WriteLine("invalid entry!");
在螢幕上列印 invalid entry!
。
如果你願意,你可能不會在程式碼中建立和使用 si
變數,因為決策是使用隨機數變數 r
做出的。
如果你希望使用者輸入 yes
或 no
再次玩遊戲,那麼你應該使用如下程式碼。
using System;
namespace Hello_World {
class Program {
static void Main(string[] args) {
bool play = true;
string q;
while (play) {
// all the above code of the game goes here
Console.WriteLine("\nDo you want to play again? ");
q = Console.ReadLine();
if (q == "yes" || q == "Yes") {
continue;
} else if (q == "no" || q == "No") {
play = false;
} else {
Console.WriteLine("invalid entry!\nQuiting...!");
play = false;
}
}
}
}
}
我們建立了一個布林型別的 play
變數,用於 while
迴圈。最初,它設定為 true,因此迴圈將無限執行,直到 play
變數設定為 false。
你可以將所有遊戲程式碼放在 while
迴圈中,然後,你可以使用 Console.WriteLine("\nDo you want to play again? ");
要求使用者再次玩或不玩。無論使用者輸入什麼,都將使用 q= Console.ReadLine();
行儲存在 q
變數中。
之後,我們將再次使用 if
條件來檢視使用者輸入的內容。
if(q=="yes"||q=="Yes")
語句檢查使用者的輸入,如果它等於是或否。如果使用者輸入了 yes
,則 continue
語句用於跳過其餘的迴圈語句並從頭開始迴圈。
如果使用者沒有輸入 yes
而是輸入 no
,則條件 else if(q=="no"||q=="No")
為真,play
變數為由 play=false;
行設定為 false,迴圈將停止,程式將終止。
如果使用者輸入了除 yes
或 no
之外的任何其他內容,則 invalid entry
將通過 Console.WriteLine("invalid entry!\nQuiting...!");
列印在螢幕上。而 play
將再次為假,程式將終止。
完整原始碼:
using System;
namespace Hello_World {
class Program {
static void Main(string[] args) {
bool play = true;
string q;
while (play) {
string ui, si;
int r;
Console.Write("What do you choose?\n\n Rock/ Paper/ Scissors? ");
ui = Console.ReadLine();
Random rnd = new Random();
r = rnd.Next(1, 4);
switch (r) {
case 1:
si = "Rock";
Console.WriteLine("Computer chose Rock");
if (ui == "Rock" || ui == "rock") {
Console.WriteLine("\nIt is a draw");
} else if (ui == "Paper" || ui == "paper") {
Console.WriteLine("\nYou Win!");
} else {
Console.WriteLine("\nYou Lose!");
}
break;
case 2:
si = "Paper";
Console.WriteLine("Computer chose Paper");
if (ui == "Rock" || ui == "rock") {
Console.WriteLine("\nYou Lose!");
} else if (ui == "Paper" || ui == "paper") {
Console.WriteLine("It is a draw");
} else {
Console.WriteLine("You win");
}
break;
case 3:
si = "Scissors";
Console.WriteLine("Computer chose Scissors");
if (ui == "Rock" || ui == "rock") {
Console.WriteLine("\nYou Win!");
} else if (ui == "Paper" || ui == "paper") {
Console.WriteLine("You Lose");
} else {
Console.WriteLine("It is a draw");
}
break;
default:
Console.WriteLine("invalid entry!");
break;
}
Console.WriteLine("\nDo you want to play again? ");
q = Console.ReadLine();
if (q == "yes" || q == "Yes") {
continue;
} else if (q == "no" || q == "No") {
play = false;
} else {
Console.WriteLine("invalid entry!\nQuiting...!");
play = false;
}
}
}
}
}
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn