How to Play an Mp3 File in a Windows Form Application in C#
- Use C# Windows Form Application to Create a Simple Mp3 Player
-
Use
DirectShow
Functionality to Create an Mp3 Windows Form Application inC#
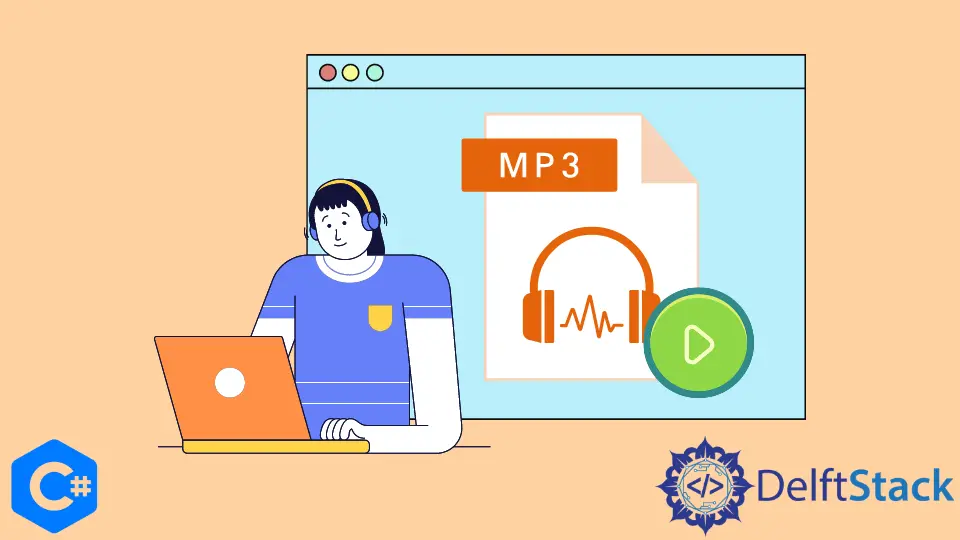
This post will demonstrate how to build a simple C# mp3 player using your C# Windows form application and a Windows Media Player.
Use C# Windows Form Application to Create a Simple Mp3 Player
First, we’ll create a new Windows form by following these steps.
-
Open Microsoft Visual Studio 2017 or updated version.
-
At the top-left corner, find
File
and open a new project. -
Then choose
Windows Forms Application
, name it, and clickOK
. -
After you click
OK
, a new empty window form will appear.
Now, we’ll design the form.
After creating the Windows form, we’ll design it. If you don’t have the Windows Media Player items in your Toolbox
, follow these steps.
-
Right-click on
Components
and clickChoose Items
in the toolbox area, a new window will appear. -
Select the
COM Components
tab, and click on theWindows Media Player
item from the list.
Adding Items:
-
Drag and drop the
Windows Media Player
onto the form, then adjust its position. -
Add a
ListBox
and name itAudiolist
to choose the mp3 file list. -
Now add a button from the toolbox and name it
ChooseButton
. We’re using a button to choose a playlist, and the listbox will display a playlist of audio files that will play inWindows Media Player
when you select one from the listbox.
We can create an MP3 Player Windows form by following these particular steps.
Libraries that are required:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
Firstly, initialize two string array-type global variables named file
and filepath
.
string[] file, filepath;
Now create an OpenFileDialog
type variable named fileOpen
, which will be used to launch a dialogue box for selecting files from the storage.
OpenFileDialog fileOpen = new OpenFileDialog();
Then we’ll apply a condition and pass the following as parameters.
if (fileOpen.ShowDialog() == System.Windows.Forms.DialogResult.OK)
The file’s name will be saved in the file
variable if this expression is true.
file = fileOpen.SafeFileNames;
Using the below command, store the whole path of the file in the path variable named filepath
.
filepath = fileOpen.FileNames;
Then we’ll use a for
loop to add audio files in ListBox
.
for (int i = 0; i < file.Length; i++) {
Audiolist.Items.Add(file[i]);
}
After that, generate a click event of ListBox
by double-clicking on it.
private void Audiolist_SelectedIndexChanged(object sender, EventArgs e) {}
This code is then pasted into the Audiolist
body. This code will transfer the list or the path to that file into your Windows Media Player, regardless of the name you give it in the ListBox
.
private void Audiolist_SelectedIndexChanged(object sender, EventArgs e) {
axWindowsMediaPlayer1.URL = filepath[Audiolist.SelectedIndex];
}
The source code for building a simple C# mp3 player using your C# Windows form application, and a Windows Media Player is below.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Mp3Player {
public partial class Form1 : Form {
string[] file, filepath;
public Form1() {
InitializeComponent();
}
private void ChooseButton_Click(object sender, EventArgs e) {
OpenFileDialog fileOpen = new OpenFileDialog();
if (fileOpen.ShowDialog() == System.Windows.Forms.DialogResult.OK) {
file = fileOpen.SafeFileNames;
filepath = fileOpen.FileNames;
for (int i = 0; i < file.Length; i++) {
Audiolist.Items.Add(file[i]);
}
}
}
private void Audiolist_SelectedIndexChanged(object sender, EventArgs e) {
axWindowsMediaPlayer1.URL = filepath[Audiolist.SelectedIndex];
}
}
}
Use DirectShow
Functionality to Create an Mp3 Windows Form Application in C#
Use DirectShow
functionality to create an Mp3 Windows form application with a Windows Media Player.
Tlbimp
transforms type definitions in a COM type library to comparable definitions in an executable assembly for a common language.
The output of Tlbimp.exe
is a binary file (an assembly) with runtime metadata for the types declared in the original type library.
To create QuartzTypeLib.dll
, follow these steps.
-
Run the
tlbimp
tool first. -
Run
TlbImp.exe %windir%\system32\quartz.dll
(This location or directory may differ from device to device.) -
The output would be
QuartzTypeLib.dll
. -
Right-click the mouse button on the project name in
Solution Explorer
, then select theAdd
menu item and thenReference
. It will addQuartzTypeLib.dll
to your project as a COM-reference. -
Expand
References
in your Project and look for theQuartzTypeLib
reference. ChangeEmbed Interop Types
to false by right-clicking it and selecting properties. -
Disable the
Prefer 32-bit
setting in Project Settings. Build tab if you don’t want to get the Exceptions.
You can use this source code to create an Mp3 Windows form application.
using QuartzTypeLib;
public sealed class DirectShowPlayer {
private FilgraphManager fgm;
public void Play(string filepath) {
FilgraphManager fgm = new FilgraphManager();
fgm.RenderFile(filepath);
fgm.Run();
}
public void Stop() {
fgm?.Stop();
}
}
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn