在 C# 中的 Windows 窗体应用程序中播放 Mp3 文件
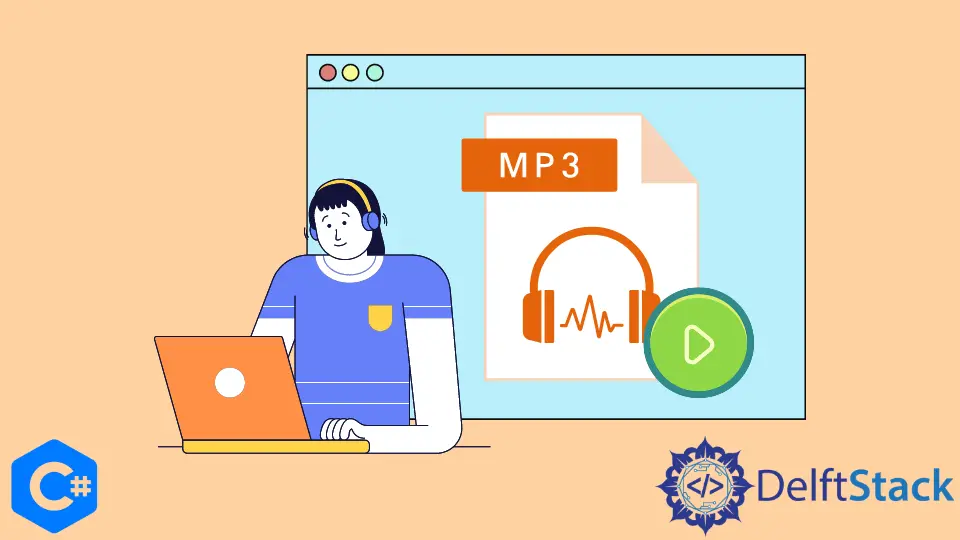
这篇文章将演示如何使用你的 C# Windows 窗体应用程序和 Windows Media Player 构建一个简单的 C# mp3 播放器。
使用 C# Windows 窗体应用程序创建一个简单的 Mp3 播放器
首先,我们将按照以下步骤创建一个新的 Windows 窗体。
-
打开 Microsoft Visual Studio 2017 或更新版本。
-
在左上角,找到
文件
并打开一个新项目。 -
然后选择
Windows Forms Application
,为其命名,然后单击OK
。 -
点击
OK
后,会出现一个新的空窗体。
现在,我们将设计表单。
创建 Windows 窗体后,我们将对其进行设计。如果你的工具箱
中没有 Windows Media Player 项目,请按照以下步骤操作。
-
右键单击
Components
,然后单击工具箱区域中的Choose Items
,将出现一个新窗口。 -
选择
COM Components
选项卡,然后单击列表中的Windows Media Player
项。
添加项目:
-
将
Windows Media Player
拖放到窗体上,然后调整其位置。 -
添加一个
ListBox
并将其命名为Audiolist
以选择 mp3 文件列表。 -
现在从工具箱中添加一个按钮并将其命名为
ChooseButton
。我们使用一个按钮来选择一个播放列表,当你从列表框中选择一个时,列表框将显示将在Windows Media Player
中播放的音频文件的播放列表。
我们可以按照这些特定步骤创建一个 MP3 Player Windows 窗体。
需要的库:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
首先,初始化两个字符串数组类型的全局变量 file
和 filepath
。
string[] file, filepath;
现在创建一个名为 fileOpen
的 OpenFileDialog
类型变量,它将用于启动一个对话框以从存储中选择文件。
OpenFileDialog fileOpen = new OpenFileDialog();
然后我们将应用一个条件并将以下内容作为参数传递。
if (fileOpen.ShowDialog() == System.Windows.Forms.DialogResult.OK)
如果该表达式为真,文件名将保存在 file
变量中。
file = fileOpen.SafeFileNames;
使用以下命令,将文件的整个路径存储在名为 filepath
的路径变量中。
filepath = fileOpen.FileNames;
然后我们将使用 for
循环在 ListBox
中添加音频文件。
for (int i = 0; i < file.Length; i++) {
Audiolist.Items.Add(file[i]);
}
之后,双击产生 ListBox
的点击事件。
private void Audiolist_SelectedIndexChanged(object sender, EventArgs e) {}
然后将此代码粘贴到 Audiolist
正文中。此代码会将列表或该文件的路径传输到你的 Windows Media Player 中,无论你在 ListBox
中为其指定的名称如何。
private void Audiolist_SelectedIndexChanged(object sender, EventArgs e) {
axWindowsMediaPlayer1.URL = filepath[Audiolist.SelectedIndex];
}
下面是使用 C# Windows 窗体应用程序和 Windows Media Player 构建简单 C# mp3 播放器的源代码。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Mp3Player {
public partial class Form1 : Form {
string[] file, filepath;
public Form1() {
InitializeComponent();
}
private void ChooseButton_Click(object sender, EventArgs e) {
OpenFileDialog fileOpen = new OpenFileDialog();
if (fileOpen.ShowDialog() == System.Windows.Forms.DialogResult.OK) {
file = fileOpen.SafeFileNames;
filepath = fileOpen.FileNames;
for (int i = 0; i < file.Length; i++) {
Audiolist.Items.Add(file[i]);
}
}
}
private void Audiolist_SelectedIndexChanged(object sender, EventArgs e) {
axWindowsMediaPlayer1.URL = filepath[Audiolist.SelectedIndex];
}
}
}
使用 DirectShow
功能在 C#
中创建 Mp3 Windows 窗体应用程序
使用 DirectShow
功能创建带有 Windows Media Player 的 Mp3 Windows 表单应用程序。
Tlbimp
将 COM 类型库中的类型定义转换为通用语言的可执行程序集中的可比较定义。
Tlbimp.exe
的输出是一个二进制文件(一个程序集),其中包含原始类型库中声明的类型的运行时元数据。
要创建 QuartzTypeLib.dll
,请按照下列步骤操作。
-
首先运行
tlbimp
工具。 -
运行
TlbImp.exe %windir%\system32\quartz.dll
(此位置或目录可能因设备而异。) -
输出将是
QuartzTypeLib.dll
。 -
在
Solution Explorer
中的项目名称上单击鼠标右键,然后选择Add
菜单项,然后选择Reference
。它会将QuartzTypeLib.dll
添加到你的项目中作为 COM 引用。 -
在你的项目中展开
References
并查找QuartzTypeLib
参考。通过右键单击并选择属性将Embed Interop Types
更改为 false。 -
禁用项目设置中的
Prefer 32-bit
设置。如果你不想获得例外,请构建选项卡。
你可以使用此源代码创建 Mp3 Windows 表单应用程序。
using QuartzTypeLib;
public sealed class DirectShowPlayer {
private FilgraphManager fgm;
public void Play(string filepath) {
FilgraphManager fgm = new FilgraphManager();
fgm.RenderFile(filepath);
fgm.Run();
}
public void Stop() {
fgm?.Stop();
}
}
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn