How to Open a Form Using a Button in C#
-
Use the
Form.Show()
Method to Open a New Form Using a Button inC#
-
Use the
Form.ShowDialog()
Method to Open a New Form Using a Button inC#
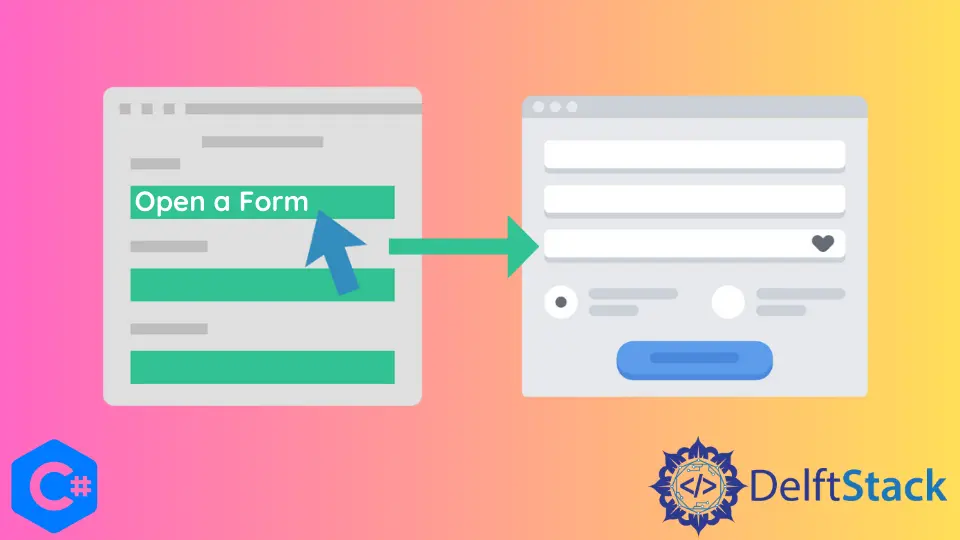
This tutorial will teach you how to access forms using buttons when developing applications in C#.
Use the Form.Show()
Method to Open a New Form Using a Button in C#
It belongs to the System.Windows.Forms
namespace and shows or displays a new form to the user. It only works for non-modal forms and can efficiently display, show, or control them.
Before calling this method, its Owner
property must be set to owner
so the new form can access this property to get information about the owning form. The new form will be visible after setting its Visible
property to true
.
You need to instantiate it as an object by creating a new object from the active form’s class. Use this instantiated object to call the Show()
method, and you can access the new form.
Code - Form1.cs
:
using System;
using System.Windows.Forms;
namespace openFormfromClickEvent {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
// create an object of `Form2` form in the current form
Form2 f2 = new Form2();
// hide the current form
this.Hide();
// use the `Show()` method to access the new non-modal form
f2.Show();
}
}
}
Output:
Using a button to access another form can have multiple benefits as you can replicate a form as needed and easily and effectively modal some basic workflow of your C# applications. In Winforms, you can structure your applications by handling forms via Click
events to manipulate the GUI.
Use the Form.ShowDialog()
Method to Open a New Form Using a Button in C#
Similar to the Form.Show()
method, It opens a new form as a model dialog box in your C# application, and every action in this dialog box is determined by its DialogResult
property.
You can set the DialogResult
property of the form programmatically or by assigning the enumeration value of the modal form to the DigitalResult
property of a button. The Form.ShowDialog()
method returns a value that can be used to determine how to process actions in the new modal form.
As a modal dialog box is set to Cancel
, it forces the form to hide, unlike the non-modal forms. When a form is no longer in service or required by your C# application, the Dispose
method becomes helpful because using the Close
method can hide a form instead of closing it, which means it can be shown again without creating a new instance of the modal form.
This method does not specify a form of control as its parent as a currently active form becomes the owner (parent) of the dialog box or modal form. If there comes a need to specify the owner of this method, use the Form.ShowDialog(IWin32Window)
version of the method, which shows the form as a modal dialog box with the specified owner.
Code - Form1.cs
:
using System;
using System.Windows.Forms;
namespace openFormfromClickEvent {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
// create an object of `Form2` form in the current form
Form2 f2 = new Form2();
// hide the current form
this.Hide();
// use the `ShowDialog()` method to access the new modal form
f2.ShowDialog();
}
}
}
Output:
To hide an active form, use this.Hide()
method and to close a form use this.Close()
method in a button_Click
event.
C# application developers must know about event handling to perform some actions on a form. The Form.cs [Design]
of your C# project in Visual Studio contains the visual representation of your form elements, and by double-click, a design element can automatically create an event handler method that controls and respond to events generated from controls and a button clicked event is one of them.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub