How to Create a Button Click Event in C#
-
An Overview of the Button Click Events in
C#
-
Implementation of Button Click Event in
C#
-
List of Events Performed in
C#
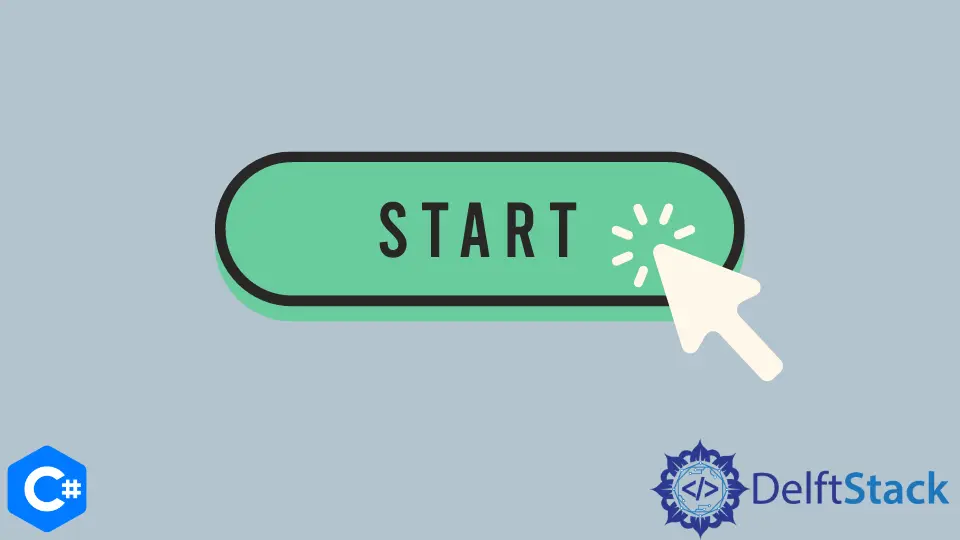
In the following post, we will learn how to build a button click event in C#. The button may be used in various contexts and for multiple purposes; however, the approach to accomplish this objective is practically the same.
An Overview of the Button Click Events in C#
Any website, software, or application has to include at least one button to function correctly. It makes it possible for the user to engage with the software or application.
You may recycle them for use in various applications, and they are available in various forms, sizes, colors, and other characteristics. Windows button controls are represented in the .NET
framework via the Button
class, which is a descendant of the ButtonBase
class.
Forms have their namespace declared in the System.Windows
namespace. To carry out the necessary activity following our specifications, we may use the Button
OnClick()
function in conjunction with the click event.
The button server control’s namespace is called System.Web.UI.WebControls
. Using button controls, users can upload pages to a web server.
One kind of control is a button, which is a submit button by default. The OnClick()
function of the button is the one that triggers the click event of the button control.
A button click event is triggered whenever a control button is selected and clicked. The click event is used rather commonly when a button control does not have an associated command name, such as in the case of a submit button.
We can specify the command name using a button’s CommandName
property. The page is sent back to the web server when the user clicks the submit button, which does not have a command name.
Implementation of Button Click Event in C#
This example code will generate a label control and two buttons for the user interface. Both buttons have an event for the click, and we have assigned a click event handler to each individually.
After a postback, the label control will indicate which button was selected when clicked. This happens because the specified button’s click event is triggered when a button is clicked.
protected void B1_C(object sender, System.EventArgs e) {
L1.Text = "first button.";
}
protected void B2_C(object sender, System.EventArgs e) {
L1.Text = "second button.";
}
The characteristics of the two buttons are outlined in the following paragraphs.
<asp:Button
ID="B1"
Height="45"
Width="150"
runat="server"
Text="First Button"
OnClick="B1_C"
/>
<asp:Button
ID="B2"
Height="45"
Width="150"
runat="server"
Text="Second Button"
OnClick="B2_C"
/>
We also have the capability of clicking the button dynamically. The next section will show you how to dynamically construct buttons and click events inside an application built using C# Windows Forms.
int i = 1;
private void B1_C(object sender, EventArgs e) {
Button btn = (sender as Button);
MessageBox.Show($"{btn.Name} clicked.", "Message", MessageBoxButtons.OK,
MessageBoxIcon.Information);
}
private void B2_C(object sender, EventArgs e) {
string n = $"Button_{i++}";
Button b = new Button() { Name = n, Text = n };
b.Size = new System.Drawing.Size(100, 25);
b.Location = new System.Drawing.Point(190, i * 35);
b.Click += B1_C;
this.Controls.Add(b);
}
Using the new
keyword results in the dynamic production of a new button. Try including this strategy in your job since it is one of the most effective methods.
Through working with this example, you have understood how to dynamically build a button and add events to the button dynamically.
List of Events Performed in C#
The following is a list of additional clicks that may be done for specific events.
Event Name | Event Action |
---|---|
MouseClick |
When you click the button with the mouse, this thing happens. |
MouseDoubleClick |
When you double-click the mouse on the button, this happens. |
MouseHover |
This action occurs when the mouse pointer is over a button. |
KeyPress |
When the control has focus, this event happens when the character, space, or backspace key is pushed. |
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn