How to Remove Illegal Characters in Filename in C#
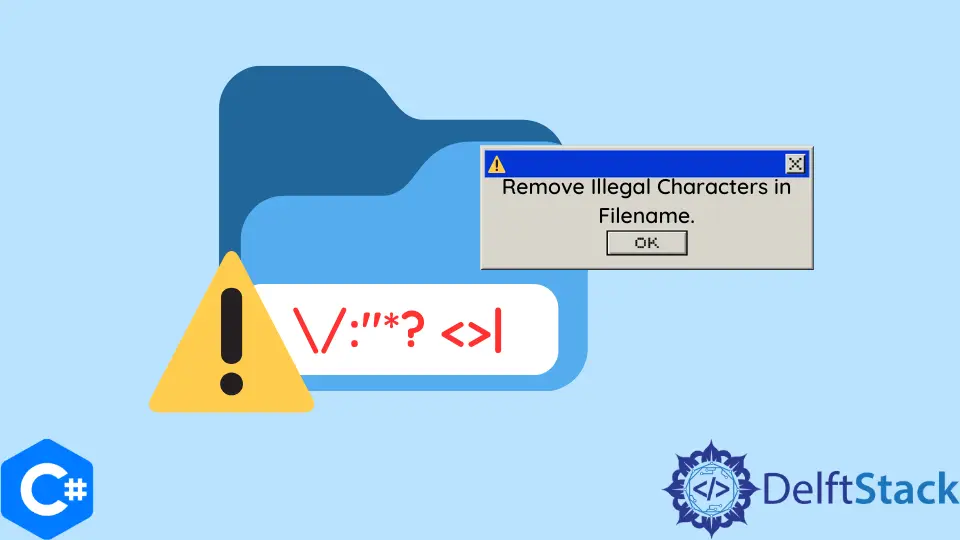
This article is a brief tutorial on getting filenames from the path using C#. It further discusses the method to remove illegal characters from the filenames.
Get Filename in C#
Some methods provided in the C# library are used for extracting the filename from a full path. The full path may contain the drive name, folder names hierarchy, and the actual file name with extension.
In many situations, we may need the filename from the paths. Therefore, we can use the GetFileName()
method in the Path
class of C# to extract the filename.
the GetFileName()
Function in C#
The syntax of the function getFileName()
is:
public static string GetFileName(string completePath);
Where completePath
is a string containing the full path from which we need to extract the filename, the function returns the filename with its extension in a string
variable.
Let’s look at the working example of GetFileName()
.
using System;
using System.IO;
using System.Text;
namespace mynamespace {
class FileNameExample {
static void Main(string[] args) {
string stringPath = "C:// files//textFiles//myfile1.txt";
string filename = Path.GetFileName(stringPath);
Console.WriteLine("Filename = " + filename);
Console.ReadLine();
}
}
}
Output:
Filename = myfile1.txt
Remove Invalid Characters From Filename in C#
The above-mentioned function may give ArgumentException
if there are some illegal characters found in the filename. These illegal characters are defined in the function GetInvalidPathChars()
and GetInvalidFilenameChars()
.
We can remove invalid or illegal characters from the filename using the following regular expression and the Replace
function.
using System;
using System.IO;
using System.Text;
using System.Text.RegularExpressions;
namespace mynamespace {
class FileNameExample {
static void Main(string[] args) {
string invalidFilename = "\"M\"\\y/Ne/ w**Fi:>> l\\/:*?\"| eN*a|m|| e\"ee.?";
string regSearch =
new string(Path.GetInvalidFileNameChars()) + new string(Path.GetInvalidPathChars());
Regex rg = new Regex(string.Format("[{0}]", Regex.Escape(regSearch)));
invalidFilename = rg.Replace(invalidFilename, "");
Console.WriteLine(invalidFilename);
}
}
}
Output:
MyNe wFi l eNam eee.
In the code snippet above, we have concatenated the invalid characters from both the functions (i.e., GetInvalidPathChars()
and GetInvalidFilenameChars()
) and created a regular expression of the resultant.
After that, we searched for all the invalid characters from our specified filename (which contains multiple invalid characters) and replaced them with blank using the Replace
function.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn