在 C# 中刪除檔名中的非法字元
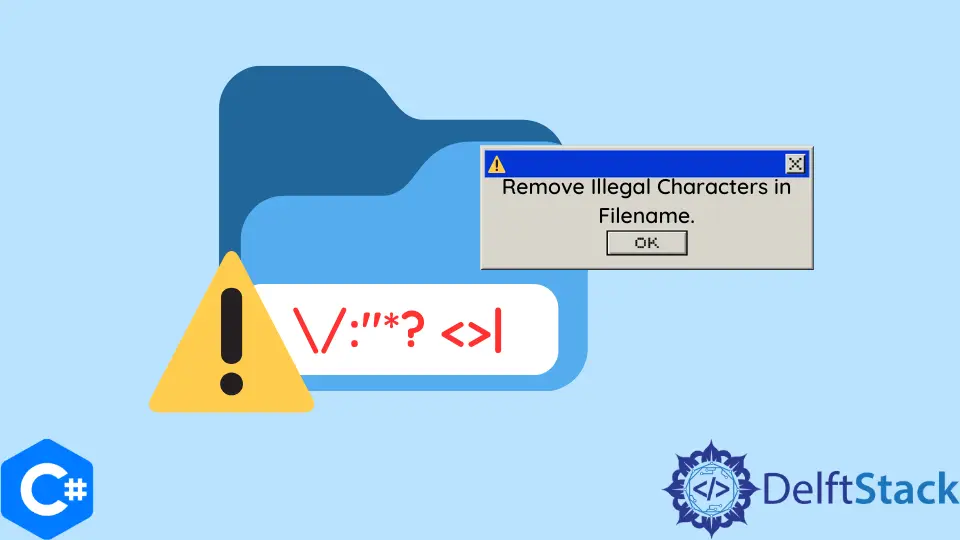
本文是關於使用 C# 從路徑中獲取檔名的簡短教程。它進一步討論了從檔名中刪除非法字元的方法。
在 C#
中獲取檔名
C# 庫中提供的一些方法用於從完整路徑中提取檔名。完整路徑可能包含驅動器名稱、資料夾名稱層次結構以及帶有副檔名的實際檔名。
在許多情況下,我們可能需要路徑中的檔名。因此,我們可以使用 C# 的 Path
類中的 GetFileName()
方法來提取檔名。
C#
中的 GetFileName()
函式
函式 getFileName()
的語法是:
public static string GetFileName(string completePath);
其中 completePath
是一個字串,其中包含我們需要從中提取檔名的完整路徑,該函式在 string
變數中返回檔名及其副檔名。
讓我們看一下 GetFileName()
的工作示例。
using System;
using System.IO;
using System.Text;
namespace mynamespace {
class FileNameExample {
static void Main(string[] args) {
string stringPath = "C:// files//textFiles//myfile1.txt";
string filename = Path.GetFileName(stringPath);
Console.WriteLine("Filename = " + filename);
Console.ReadLine();
}
}
}
輸出:
Filename = myfile1.txt
在 C#
中從檔名中刪除無效字元
如果在檔名中發現一些非法字元,上述函式可能會給出 ArgumentException
。這些非法字元在函式 GetInvalidPathChars()
和 GetInvalidFilenameChars()
中定義。
我們可以使用以下正規表示式和 Replace
函式從檔名中刪除無效或非法字元。
using System;
using System.IO;
using System.Text;
using System.Text.RegularExpressions;
namespace mynamespace {
class FileNameExample {
static void Main(string[] args) {
string invalidFilename = "\"M\"\\y/Ne/ w**Fi:>> l\\/:*?\"| eN*a|m|| e\"ee.?";
string regSearch =
new string(Path.GetInvalidFileNameChars()) + new string(Path.GetInvalidPathChars());
Regex rg = new Regex(string.Format("[{0}]", Regex.Escape(regSearch)));
invalidFilename = rg.Replace(invalidFilename, "");
Console.WriteLine(invalidFilename);
}
}
}
輸出:
MyNe wFi l eNam eee.
在上面的程式碼片段中,我們將兩個函式(即 GetInvalidPathChars()
和 GetInvalidFilenameChars()
)中的無效字元連線起來,並對結果建立一個正規表示式。
之後,我們從指定的檔名(包含多個無效字元)中搜尋所有無效字元,並使用 Replace
函式將它們替換為空白。
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn