The #if DEBUG in C#
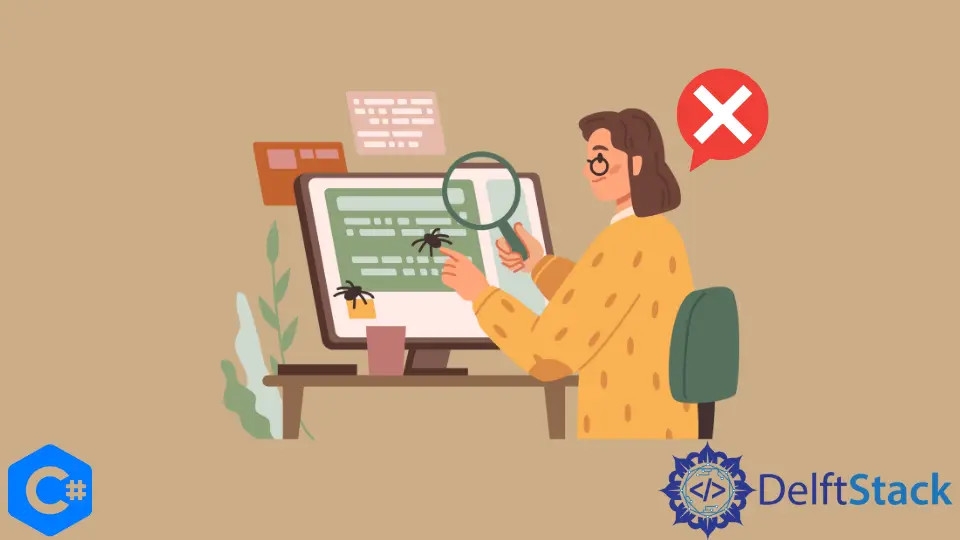
When programming in C#, developers often encounter situations where they need to differentiate between debug and release builds. One of the most effective ways to achieve this is through the use of the #if DEBUG
preprocessor directive. This powerful feature allows developers to include or exclude code segments based on the build configuration, making it an essential tool for debugging and testing.
In this article, we’ll explore the #if DEBUG
directive in detail, discussing its benefits, usage, and how it integrates with version control systems like Git. Whether you’re a novice or an experienced developer, understanding this directive can enhance your coding practices and improve the maintainability of your applications.
Understanding the #if DEBUG Directive
The #if DEBUG
directive is a preprocessor command in C# that allows developers to conditionally compile code based on whether the DEBUG symbol is defined. This is particularly useful for including diagnostic code that you only want to run during development, such as logging or debugging statements. When you compile your application in Debug mode, the DEBUG symbol is automatically defined, allowing the enclosed code to execute. Conversely, when you compile in Release mode, the code within the #if DEBUG
block is omitted, resulting in a cleaner and more optimized build.
Here’s a simple example of how the #if DEBUG
directive works:
#if DEBUG
Console.WriteLine("This code runs only in Debug mode.");
#endif
When you compile this code in Debug mode, it outputs the message. However, in Release mode, this line is ignored entirely. This feature is invaluable for maintaining clean production code while still allowing for extensive debugging during development.
Using #if DEBUG with Git
When working with Git, it’s essential to manage your code effectively, especially when switching between different build configurations. You can use Git to track changes in your codebase, including any modifications that involve the #if DEBUG
directive. Here’s how to handle this with Git commands.
-
Check Your Current Branch
Before making changes, ensure you’re on the correct branch. Use the following command:git branch
This command lists all branches and highlights the current one. Make sure you are on the branch where you want to implement or test changes related to the
#if DEBUG
directive. -
Create a New Branch for Debugging
If you want to experiment with debugging code, it’s a good practice to create a new branch. You can do this with:git checkout -b debug-feature
This command creates a new branch called
debug-feature
and switches to it, allowing you to make changes without affecting the main branch. -
Add and Commit Changes
After implementing your#if DEBUG
code, stage your changes and commit them. Use:git add . git commit -m "Added debug logging with #if DEBUG"
This command stages all changes and commits them with a descriptive message, making it easier to track what was modified.
-
Merge Changes
Once you’re satisfied with your debugging changes, you can merge them back into the main branch:git checkout main git merge debug-feature
This sequence switches back to the main branch and merges your debug changes, ensuring that your production code remains clean while still benefiting from the debugging features during development.
By using Git effectively, you can manage your debugging code, ensuring it remains isolated and does not clutter your production builds.
Conclusion
The #if DEBUG
directive in C# is an essential tool for developers, enabling them to manage debugging code efficiently. By conditionally compiling code, you can maintain a clear separation between development and production environments. Additionally, integrating this directive with Git allows for better version control and code management. Whether you’re logging errors, testing features, or optimizing performance, understanding how to use #if DEBUG
can significantly enhance your development workflow.
FAQ
-
What is the purpose of the #if DEBUG directive in C#?
The #if DEBUG directive allows developers to conditionally compile code that should only run in Debug mode, enabling easier debugging and testing. -
How does the #if DEBUG directive affect performance?
Code within the #if DEBUG block is excluded in Release mode, resulting in optimized performance and cleaner production builds. -
Can I use #if DEBUG with other preprocessor directives?
Yes, you can combine #if DEBUG with other directives like #else or #elif to create more complex conditional compilation scenarios. -
How does Git help manage changes related to #if DEBUG?
Git allows you to create branches, stage, and commit changes specifically related to debugging, ensuring your production code remains unaffected. -
Is it possible to define custom symbols for conditional compilation?
Yes, you can define custom symbols in your project settings, allowing for more tailored conditional compilation beyond just DEBUG and RELEASE.
#if DEBUG in C#, a powerful preprocessor directive that allows developers to conditionally compile code for debugging purposes. Learn how to effectively manage your code with Git while utilizing this directive to enhance your debugging process and maintain clean production builds. Discover practical examples and best practices for incorporating #if DEBUG into your development workflow.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn