HashMap in C#
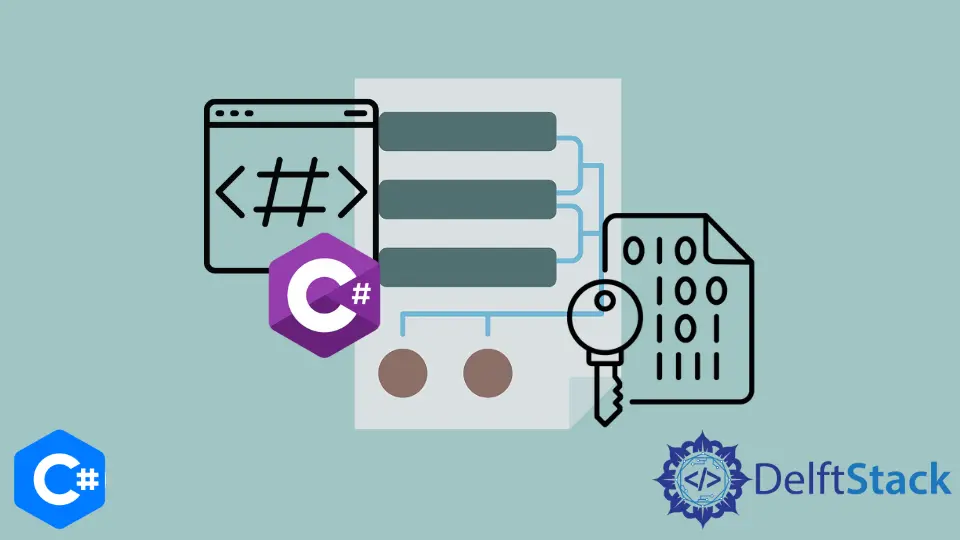
In the intricate landscape of C# programming, the Dictionary
collection stands out as a cornerstone for handling complex data structures efficiently. This detailed guide focuses on harnessing the full potential of the Dictionary<TKey, TValue>
as an equivalent to HashMap in other programming languages.
By delving into the mechanics of this collection, found in the System.Collections.Generic
namespace, we unravel how it elegantly handles key-value pairs, providing a robust framework for rapid data retrieval and manipulation.
Use the Dictionary
Collection as Hashmap Equivalent in C#
We will use the Dictionary
collection as hashmap equivalent in C#. It represents a collection of key-value pairs.
This collection, which resides in the System.Collections.Generic
namespace, is analogous to what many programming languages, such as Java, term as a HashMap. The primary purpose of a Dictionary<TKey, TValue>
is to store data in key-value pairs, offering rapid retrieval of values based on unique keys.
The key-value pair means that every value has a key. The correct syntax to create a dictionary is as follows.
Dictionary<TKey, TValue> dictionaryName = new Dictionary<TKey, TValue>();
The Dictionary<TKey, TValue>
requires two type parameters:
TKey
: The type of the keys in the dictionary.TValue
: The type of the values in the dictionary.
There are multiple methods to perform operations on the created dictionary such as Add()
, Clear()
, ContainsKey()
, ContainsValue()
, Equals()
, GetType()
, Remove()
, etc.
The program below shows how we can add elements to the dictionary.
using System;
using System.Collections.Generic;
class Program {
static void Main() {
// Creating a dictionary to store integer IDs and their corresponding names
Dictionary<int, string> employeeDirectory = new Dictionary<int, string>();
// Adding key-value pairs to the dictionary
employeeDirectory.Add(101, "Alice");
employeeDirectory.Add(102, "Bob");
employeeDirectory.Add(103, "Charlie");
// Accessing a value using its key
Console.WriteLine("Employee 102: " + employeeDirectory[102]);
// Iterating through the dictionary
foreach (KeyValuePair<int, string> kvp in employeeDirectory) {
Console.WriteLine($"ID: {kvp.Key}, Name: {kvp.Value}");
}
}
}
In our example, we create a Dictionary<int, string>
named employeeDirectory
, where each employee’s ID (an int
) is associated with their name (a string
). We add three key-value pairs to our dictionary using the Add
method. The keys are unique employee IDs, and the values are their respective names.
To demonstrate data retrieval, we access the name of the employee with ID 102
using employeeDirectory[102]
. This showcases the efficiency of a dictionary in fetching values with a known key.
Next, we iterate over the dictionary using a foreach
loop. The KeyValuePair<int, string>
represents each key-value pair in our dictionary, allowing us to print out each employee’s ID and name.
Output:
There are some limitations to the Dictionary
collection. We cannot add null keys to it. If we do, then it will throw ArgumentNullException
.
Conclusion
As we conclude our exploration of the Dictionary<TKey, TValue>
collection in C#, it’s evident that this data structure is much more than a mere equivalent to a HashMap. It’s a powerful tool that brings a high degree of efficiency and sophistication to data management in C#.
The Dictionary
collection not only offers rapid data access and manipulation through its key-value pair structure but also ensures that these operations are intuitive and adaptable to various scenarios. The practical example provided illustrates the ease and flexibility with which data can be stored, retrieved, and iterated, making it an invaluable asset for developers.