HashMap in C#
Minahil Noor
16 Februar 2024
Csharp
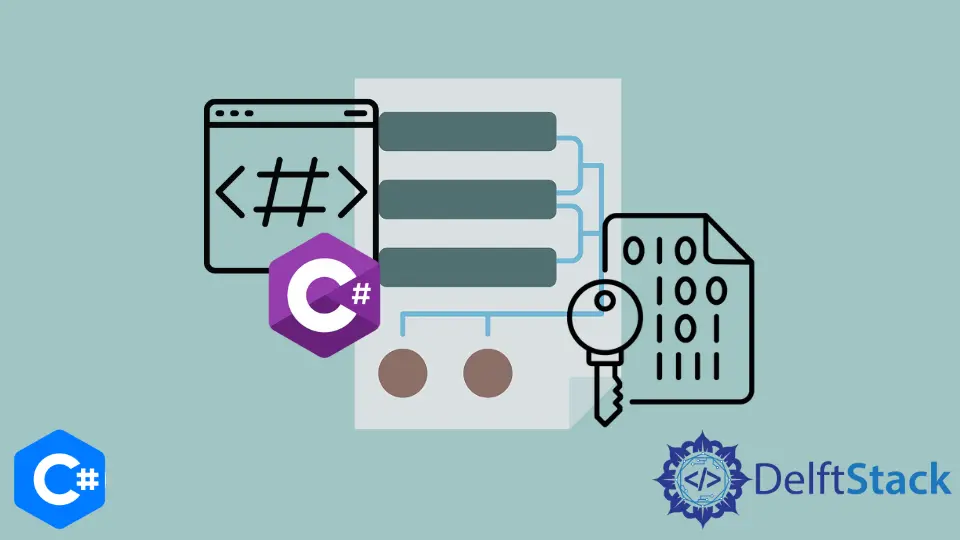
In diesem Artikel wird ein Hashmap-Äquivalent in C# eingeführt.
Verwendung von die Sammlung Dictionary
als Hashmap-Äquivalent in C#
Wir werden die Sammlung Dictionary
als Hashmap-Äquivalent in C# verwenden. Es repräsentiert eine Sammlung von Schlüssel-Wert-Paaren. Das Schlüssel-Wert-Paar bedeutet, dass jeder Wert einen Schlüssel hat. Die korrekte Syntax zum Erstellen ein Dictionary lautet wie folgt.
IDictionary<type, type> numberNames = new Dictionary<type, type>();
Es gibt mehrere Methoden, um Operationen für das erstellte Dictionary auszuführen, z. B. Add()
, Clear()
, ContainsKey()
, ContainsValue()
, Equals()
, GetType()
, Remove()
usw.
Das folgende Programm zeigt, wie wir dem Dictionary Elemente hinzufügen können.
using System;
using System.Collections.Generic;
public class Program {
public static void Main() {
IDictionary<int, string> flowerNames = new Dictionary<int, string>();
flowerNames.Add(1, "Rose");
flowerNames.Add(2, "Jasmine");
flowerNames.Add(3, "Lili");
foreach (KeyValuePair<int, string> kvp in flowerNames)
Console.WriteLine("Key: {0}, Value: {1}", kvp.Key, kvp.Value);
}
}
Ausgabe:
Key: 1, Value: Rose
Key: 2, Value: Jasmine
Key: 3, Value: Lili
Die Sammlung Dictionary
weist einige Einschränkungen auf. Wir können keine Nullschlüssel hinzufügen. Wenn wir dies tun, wird ArgumentNullException
ausgelöst.
Genießen Sie unsere Tutorials? Abonnieren Sie DelftStack auf YouTube, um uns bei der Erstellung weiterer hochwertiger Videoanleitungen zu unterstützen. Abonnieren