How to Find the Square Root Without Using the SQRT Function in C++
-
Find Square Roots in C++ Without Using the
sqrt
Function: Newton’s Method -
Find Square Roots in C++ Without Using the
sqrt
Function: Binary Search Method -
Find Square Roots in C++ Without Using the
sqrt
Function: Babylonian (Heron’s) Method -
Find Square Roots in C++ Without Using the
sqrt
Function: Exponential Function Method - Conclusion
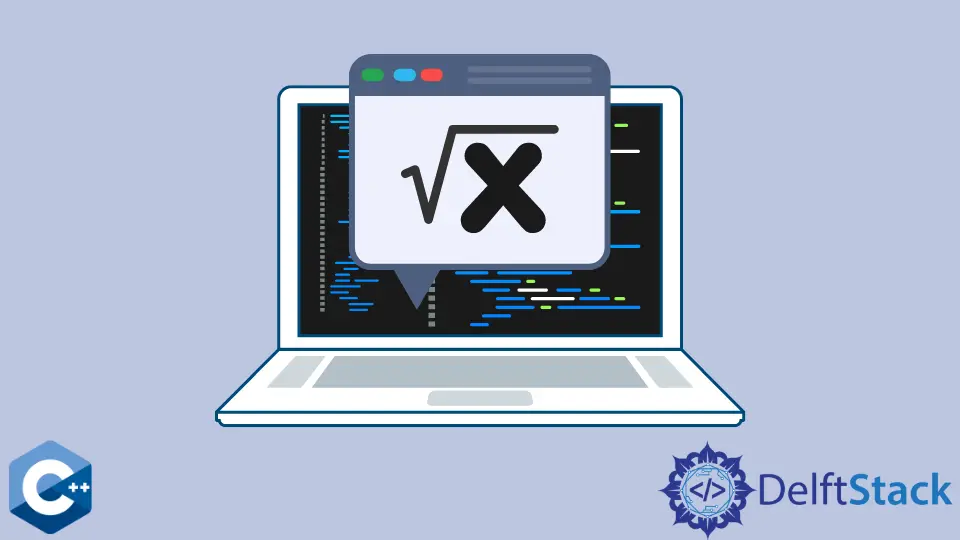
The sqrt()
function is a built-in C++ function that calculates the square root of a number. It accepts one argument, n
, and returns the square root of n
.
But did you know that we can find the square root of a number in C++ without using the sqrt()
function? In this article, we will explain four distinct approaches: Newton’s Method, Binary Search, Babylonian (Heron’s) Method, and the Exponential Function Method.
Find Square Roots in C++ Without Using the sqrt
Function: Newton’s Method
Another elegant numerical method for finding square roots is Newton’s Method. Also known as the Newton-Raphson method, this is an iterative numerical technique for finding the roots of real-valued functions.
The basic idea behind Newton’s Method is to start with an initial guess and iteratively refine that guess until it converges to the actual square root.
Here’s a breakdown of Newton’s Method algorithm for finding square roots in C++:
-
Start by initializing the variables for the algorithm. Set the initial guess (
x
) to the target number (n
). Define a small value (epsilon
) to determine the precision of the result. -
Choose a value for
epsilon
based on the desired precision of the square root calculation. This value determines when the iteration should stop. -
Enter a loop to iteratively refine the guess. The loop continues until the difference between
x * x
and the target numbern
is smaller than the defined precision (epsilon
). -
Inside the loop, update the guess (
x
) using the Newton’s formula:x = 0.5 * (x + n / x)
. This update is designed to bring the guess closer to the actual square root. -
Check if the difference between the square of the current guess and the target number is smaller than the specified precision. If it is, exit the loop as the iteration has converged.
-
After the loop, return the final value of
x
as the calculated square root.
Let’s implement Newton’s Method for finding square roots in C++:
#include <iostream>
double squareRoot(double n) {
double x = n;
double epsilon = 0.000001;
while ((x * x - n) > epsilon) {
x = 0.5 * (x + n / x);
}
return x;
}
int main() {
double number;
std::cout << "Enter a number: ";
std::cin >> number;
std::cout << "Square root: " << squareRoot(number) << std::endl;
return 0;
}
Output:
Enter a number: 5
Square root: 2.23607
In this example, we were prompted to enter a number, and in this case, we input 5
. The program then calculates the square root of 5
using Newton’s Method.
The result, approximately 2.23607
, is then displayed.
It’s important to note that the precision of the result depends on the chosen value of epsilon
. A smaller epsilon
will yield a more accurate result but might require more iterations.
This method is sensitive to the initial guess. Choosing a good initial guess can lead to faster convergence.
Find Square Roots in C++ Without Using the sqrt
Function: Binary Search Method
Binary search is a well-known algorithm primarily used for finding an element in a sorted array. Surprisingly, it can also be adapted to find square roots.
The core idea involves performing a binary search within a specified range, systematically narrowing down possibilities until the square root is found within the desired precision.
Let’s delve into the implementation of the Binary Search method for finding square roots in C++:
-
Set the initial range.
- Initialize two variables,
low
andhigh
, representing the lower and upper bounds of the search range, respectively. For square roots, these could be set to0.0
and the target number (n
). - Also, initialize variables
mid
andguess
for the midpoint and the square of the guess.
- Initialize two variables,
-
Set a precision threshold (
epsilon
) to determine the level of accuracy you desire in the result. This value guides when the search should stop. -
Define the Binary Search loop. Enter a loop that continues until the difference between
high
andlow
is less thanepsilon
. -
Within the loop, calculate the midpoint (
mid
) and the square of the guess (guess
) using the formulamid = (low + high) / 2
andguess = mid * mid
. -
Based on the comparison between
guess
and the target number (n
), adjust the search range. Ifguess
is greater thann
, sethigh
tomid
; otherwise, setlow
tomid
. -
Once the loop exits, the final result is in the variable
mid
, and it represents the square root of the target number.
Here is the complete implementation:
#include <iostream>
double squareRoot(double n) {
double low = 0.0;
double high = n;
double mid, guess;
double epsilon = 0.000001; // Set your desired precision
while ((high - low) > epsilon) {
mid = (low + high) / 2;
guess = mid * mid;
if (guess > n) {
high = mid;
} else {
low = mid;
}
}
return low;
}
int main() {
double number;
std::cout << "Enter a number: ";
std::cin >> number;
std::cout << "Square root: " << squareRoot(number) << std::endl;
return 0;
}
For instance, let’s consider an input of 5
:
Enter a number: 5
Square root: 2.23607
In this example, the program prompts the user to enter a number (5
). The algorithm then utilizes Binary Search to calculate the square root, yielding a result of approximately 2.23607
.
Ensure the initial range (low
and high
) encapsulates the potential square root. The adjustment of the search range based on the comparison with the target number is crucial for the algorithm’s success.
Find Square Roots in C++ Without Using the sqrt
Function: Babylonian (Heron’s) Method
When it comes to calculating square roots in C++ without relying on the built-in square root function, the Babylonian Method, also known as Heron’s Method, is also a powerful iterative technique.
Named after the ancient Babylonian mathematicians and the Greek mathematician Heron of Alexandria, this method provides an efficient and accurate approach to finding square roots.
The Babylonian Method is an iterative algorithm based on the principle of refining an initial guess until it converges to the true square root. It’s particularly effective due to its simplicity and rapid convergence.
-
Begin by initializing a variable
x
with the target number for which the square root is to be calculated. -
Define a precision threshold (
epsilon
) to determine the level of accuracy you desire in the result. -
Enter a loop where the guess is updated iteratively using the formula
x = 0.5 * (x + n / x)
, wheren
is the target number. -
Check if the difference between the square of the current guess (
x * x
) and the target number (n
) is smaller than the defined precision (epsilon
). If the condition is met, exit the loop; otherwise, continue the iteration. -
After the loop, return the final value of
x
as the calculated square root.
Let’s implement the Babylonian (Heron’s) Method for finding square roots in C++:
#include <iostream>
double squareRoot(double n) {
double x = n;
double epsilon = 0.000001; // Set your desired precision
while ((x * x - n) > epsilon) {
x = 0.5 * (x + n / x);
}
return x;
}
int main() {
double number;
std::cout << "Enter a number: ";
std::cin >> number;
std::cout << "Square root: " << squareRoot(number) << std::endl;
return 0;
}
For instance, let’s consider an input of 5
:
Enter a number: 5
Square root: 2.23607
In this example, the program prompts the user to enter a number (5
). The Babylonian Method is then applied to calculate the square root, resulting in a precision of approximately 2.23607
.
The precision of the result depends on the chosen value of epsilon
. A smaller epsilon
will yield a more accurate result but might require more iterations.
While the method is robust, choosing a good initial guess can influence the convergence speed.
Find Square Roots in C++ Without Using the sqrt
Function: Exponential Function Method
The Exponential Function Method exploits the fact that the square root of a number n
can be expressed as n^(1/2)
or, equivalently, exp(0.5 * log(n))
in terms of the exponential and logarithmic functions. Leveraging these mathematical relationships, we can bypass the direct use of square root functions in C++.
-
Begin by obtaining user input for the target number (
n
) for which the square root needs to be calculated. -
Utilize the exponential function (
exp()
) in C++ to calculateexp(0.5 * log(n))
. This mathematical expression effectively yields the square root of the input number. -
Output the calculated square root to the user.
Let’s implement the Exponential Function Method for finding square roots in C++:
#include <iostream>
#include <cmath>
double squareRoot(double n) {
return exp(0.5 * log(n));
}
int main() {
double number;
std::cout << "Enter a number: ";
std::cin >> number;
std::cout << "Square root: " << squareRoot(number) << std::endl;
return 0;
}
Consider an input of 5
:
Enter a number: 5
Square root: 2.23607
In this example, the program prompts the user to enter a number (5
). The Exponential Function Method is then applied to calculate the square root, resulting in a precision of approximately 2.23607
.
Note that the method relies on the <cmath>
library for mathematical functions, so ensure proper inclusion.
While elegant, the Exponential Function Method may have limitations for extremely large or small numbers due to limitations in numerical precision.
Conclusion
This article explored various methods for calculating square roots in C++ without relying on the built-in sqrt
function. Each method—Newton’s Method, Binary Search, Babylonian (Heron’s) Method, and the Exponential Function Method—offers a unique approach to iteratively refine guesses until convergence to the actual square root is achieved.
It’s important to note that the precision of these methods depends on the chosen epsilon value, and the efficiency may vary based on the nature of the input and the selected algorithm. These methods not only present viable alternatives to the sqrt
function but also offer a deeper understanding of numerical methods, algorithmic principles, and the interplay between mathematics and programming.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook