How to Sort Strings Alphabetically in C++
-
Use the
std::sort
Algorithm to Sort Strings Alphabetically in C++ -
Use
std::sort
Algorithm to Sort Strings by Length in C++
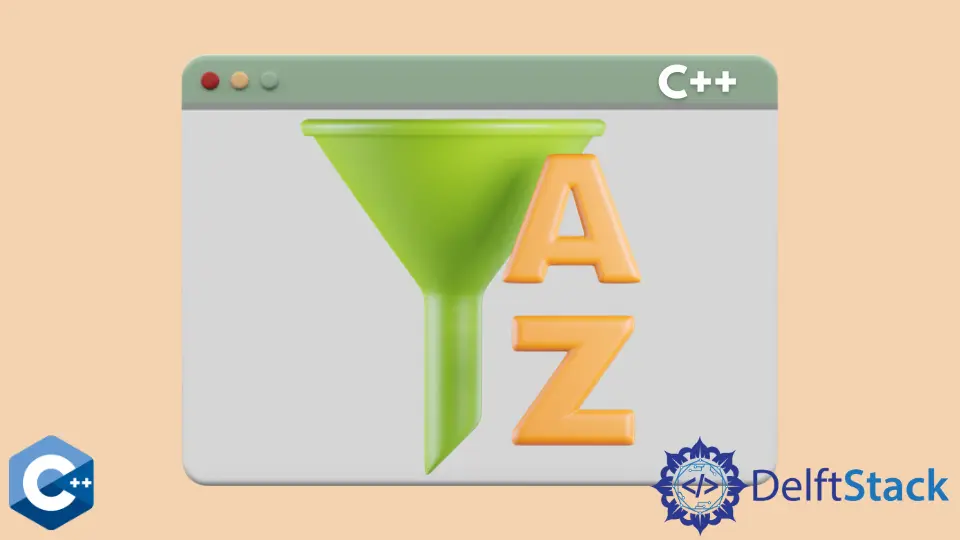
This article will demonstrate multiple methods of how to sort strings alphabetically in C++.
Use the std::sort
Algorithm to Sort Strings Alphabetically in C++
std::sort
is part of the STL algorithms library, and it implements a generic sorting method for the range-based structures. The function usually sorts the given sequence using the operator<
; thus, string objects can be alphabetically sorted with this default behavior. std::sort
takes only range specifiers - the first and the last element iterators. Note that the order of equal elements is not guaranteed to be preserved.
In the following example, we demonstrate the basic scenario, where the vector
of strings is sorted and printed to the cout
stream.
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
int main() {
vector<string> arr = {
"raid", "implementation", "states", "all", "the",
"requirements", "parameter", "a", "and", "or",
"execution", "participate"};
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
sort(arr.begin(), arr.end());
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
Output:
raid; implementation; states; all; the; requirements; parameter; a; and; or; execution; participate;
a; all; and; execution; implementation; or; parameter; participate; raid; requirements; states; the;
Alternatively, we can use the optional third argument in the std::sort
method to specify the exact comparison function. In this case, we reimplemented the previous code example to compare the last letters from the strings and sort them accordingly. Notice that the comparison function should have two arguments and return a bool
value. The next example uses the lambda expression to act as the comparison function, and it utilizes the back
built-in function to retrieve the last letters of the strings.
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
int main() {
vector<string> arr = {
"raid", "implementation", "states", "all", "the",
"requirements", "parameter", "a", "and", "or",
"execution", "participate"};
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
sort(arr.begin(), arr.end(),
[](string &s1, string &s2) { return s1.back() < s2.back(); });
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
Output:
raid; implementation; states; all; the; requirements; parameter; a; and; or; execution; participate;
a; raid; and; the; participate; all; implementation; execution; parameter; or; states; requirements;
Use std::sort
Algorithm to Sort Strings by Length in C++
Another useful case to sort the vector of strings is by their length. We will use the same structure of the lambda function as the previous example code, just changing the back
method with the size
. Mind though, that comparison function must not modify the objects passed to it.
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
int main() {
vector<string> arr = {
"raid", "implementation", "states", "all", "the",
"requirements", "parameter", "a", "and", "or",
"execution", "participate"};
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
sort(arr.begin(), arr.end(),
[](string &s1, string &s2) { return s1.size() < s2.size(); });
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
Output:
raid; implementation; states; all; the; requirements; parameter; a; and; or; execution; participate;
a; or; all; the; and; raid; states; parameter; execution; participate; requirements; implementation;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook