C++에서 알파벳순으로 문자열 정렬
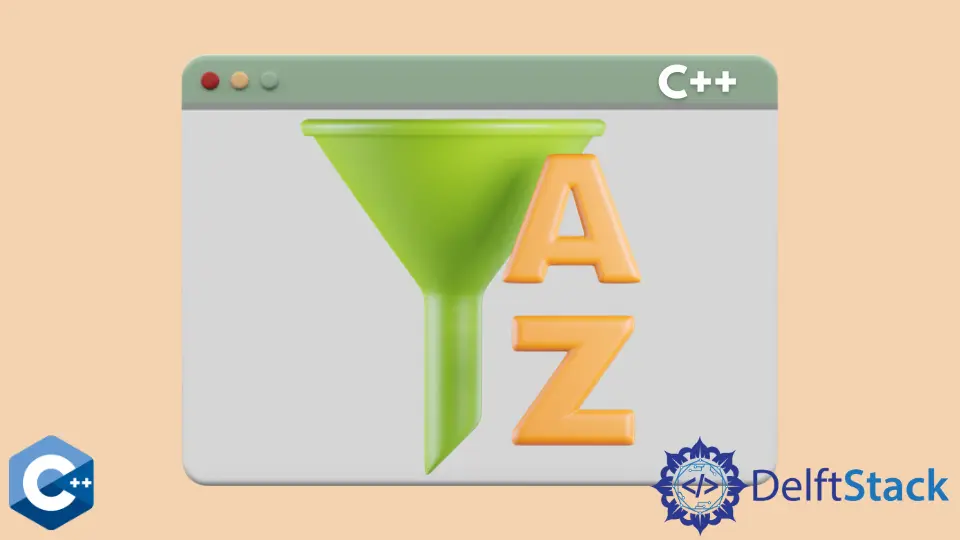
이 기사에서는 C++에서 문자열을 알파벳순으로 정렬하는 방법에 대한 여러 가지 방법을 보여줍니다.
std::sort
알고리즘을 사용하여 C++에서 알파벳순으로 문자열 정렬
std::sort
는 STL 알고리즘 라이브러리의 일부이며 범위 기반 구조에 대한 일반적인 정렬 방법을 구현합니다. 이 함수는 보통operator<
를 사용하여 주어진 시퀀스를 정렬합니다. 따라서이 기본 동작을 사용하여 문자열 개체를 알파벳순으로 정렬 할 수 있습니다. std::sort
는 범위 지정자 (첫 번째 및 마지막 요소 반복자) 만 사용합니다. 동일한 요소의 순서가 유지된다는 보장은 없습니다.
다음 예에서는 문자열의vector
가 정렬되고cout
스트림에 인쇄되는 기본 시나리오를 보여줍니다.
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
int main() {
vector<string> arr = {
"raid", "implementation", "states", "all", "the",
"requirements", "parameter", "a", "and", "or",
"execution", "participate"};
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
sort(arr.begin(), arr.end());
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
출력:
raid; implementation; states; all; the; requirements; parameter; a; and; or; execution; participate;
a; all; and; execution; implementation; or; parameter; participate; raid; requirements; states; the;
또는std::sort
메소드에서 선택적 세 번째 인수를 사용하여 정확한 비교 함수를 지정할 수 있습니다. 이 경우 이전 코드 예제를 다시 구현하여 문자열의 마지막 문자를 비교하고 그에 따라 정렬했습니다. 비교 함수에는 두 개의 인수가 있어야하며bool
값을 반환해야합니다. 다음 예제에서는 람다 식을 사용하여 비교 함수로 작동하고back
내장 함수를 사용하여 문자열의 마지막 문자를 검색합니다.
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
int main() {
vector<string> arr = {
"raid", "implementation", "states", "all", "the",
"requirements", "parameter", "a", "and", "or",
"execution", "participate"};
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
sort(arr.begin(), arr.end(),
[](string &s1, string &s2) { return s1.back() < s2.back(); });
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
출력:
raid; implementation; states; all; the; requirements; parameter; a; and; or; execution; participate;
a; raid; and; the; participate; all; implementation; execution; parameter; or; states; requirements;
std::sort
알고리즘을 사용하여 C++에서 길이별로 문자열 정렬
문자열 벡터를 정렬하는 또 다른 유용한 경우는 길이 기준입니다. 이전 예제 코드와 동일한 람다 함수 구조를 사용하여size
를 사용하여back
메소드를 변경합니다. 하지만 비교 함수는 전달 된 객체를 수정해서는 안됩니다.
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
int main() {
vector<string> arr = {
"raid", "implementation", "states", "all", "the",
"requirements", "parameter", "a", "and", "or",
"execution", "participate"};
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
sort(arr.begin(), arr.end(),
[](string &s1, string &s2) { return s1.size() < s2.size(); });
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
출력:
raid; implementation; states; all; the; requirements; parameter; a; and; or; execution; participate;
a; or; all; the; and; raid; states; parameter; execution; participate; requirements; implementation;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook