C++ で文字列をアルファベット順に並べ替える
胡金庫
2023年10月12日
C++
C++ Sorting
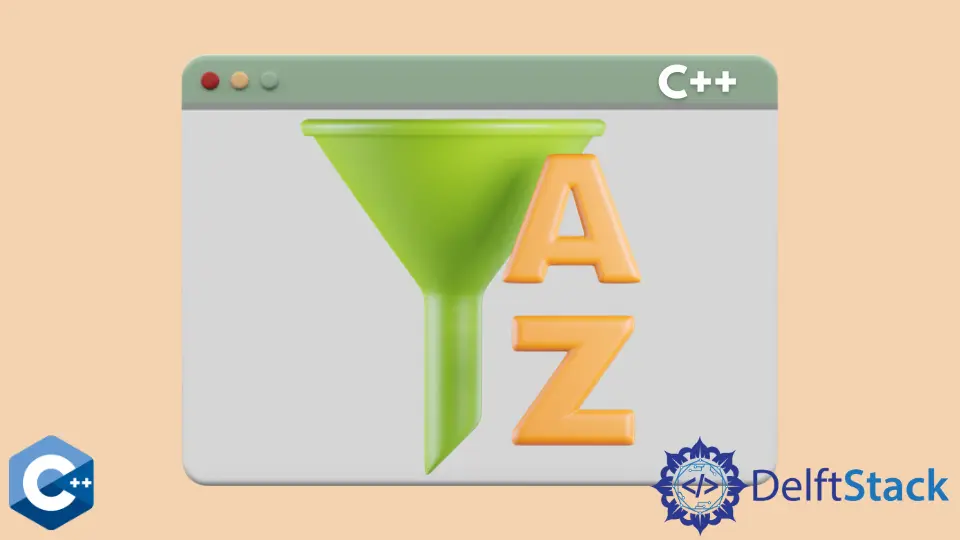
この記事では、C++ で文字列をアルファベット順に並べ替える方法の複数の方法を示します。
C++ で文字列をアルファベット順に並べるには std::sort
アルゴリズムを使う
std::sort
は STL アルゴリズムライブラリの一部であり、範囲ベースの構造の一般的なソート方法を実装しています。この関数は通常、operator<
を使用して指定されたシーケンスをソートします。したがって、文字列オブジェクトは、このデフォルトの動作でアルファベット順に並べ替えることができます。std::sort
は、範囲指定子(最初と最後の要素イテレータ)のみを取ります。等しい要素の順序が保持されるとは限らないことに注意してください。
次の例では、文字列のベクトル
がソートされ、cout
ストリームに出力される基本的なシナリオを示します。
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
int main() {
vector<string> arr = {
"raid", "implementation", "states", "all", "the",
"requirements", "parameter", "a", "and", "or",
"execution", "participate"};
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
sort(arr.begin(), arr.end());
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
出力:
raid; implementation; states; all; the; requirements; parameter; a; and; or; execution; participate;
a; all; and; execution; implementation; or; parameter; participate; raid; requirements; states; the;
または、std::sort
メソッドのオプションの 3 番目の引数を使用して、正確な比較関数を指定することもできます。この場合、前のコード例を再実装して、文字列の最後の文字を比較し、それに応じて並べ替えました。比較関数には 2つの引数があり、bool
値を返す必要があることに注意してください。次の例では、ラムダ式を使用して比較関数として機能し、back
組み込み関数を使用して文字列の最後の文字を取得します。
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
int main() {
vector<string> arr = {
"raid", "implementation", "states", "all", "the",
"requirements", "parameter", "a", "and", "or",
"execution", "participate"};
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
sort(arr.begin(), arr.end(),
[](string &s1, string &s2) { return s1.back() < s2.back(); });
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
出力:
raid; implementation; states; all; the; requirements; parameter; a; and; or; execution; participate;
a; raid; and; the; participate; all; implementation; execution; parameter; or; states; requirements;
C++ で std::sort
アルゴリズムを使用して文字列を長さで並べ替える
文字列のベクトルを並べ替えるもう 1つの便利なケースは、文字列の長さです。前のサンプルコードと同じラムダ関数の構造を使用しますが、size
で back
メソッドを変更するだけです。ただし、その比較関数は、渡されたオブジェクトを変更してはなりません。
#include <iostream>
#include <string>
#include <vector>
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
int main() {
vector<string> arr = {
"raid", "implementation", "states", "all", "the",
"requirements", "parameter", "a", "and", "or",
"execution", "participate"};
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
sort(arr.begin(), arr.end(),
[](string &s1, string &s2) { return s1.size() < s2.size(); });
for (const auto &item : arr) {
cout << item << "; ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
出力:
raid; implementation; states; all; the; requirements; parameter; a; and; or; execution; participate;
a; or; all; the; and; raid; states; parameter; execution; participate; requirements; implementation;
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: 胡金庫