Roman Numeral Converter in C++
- Roman Numeral Converter in C++
- Convert a Decimal Number to a Roman Numeral in C++
- C++ Code Example to Convert a Decimal Number to a Roman Numeral
- Conclusion
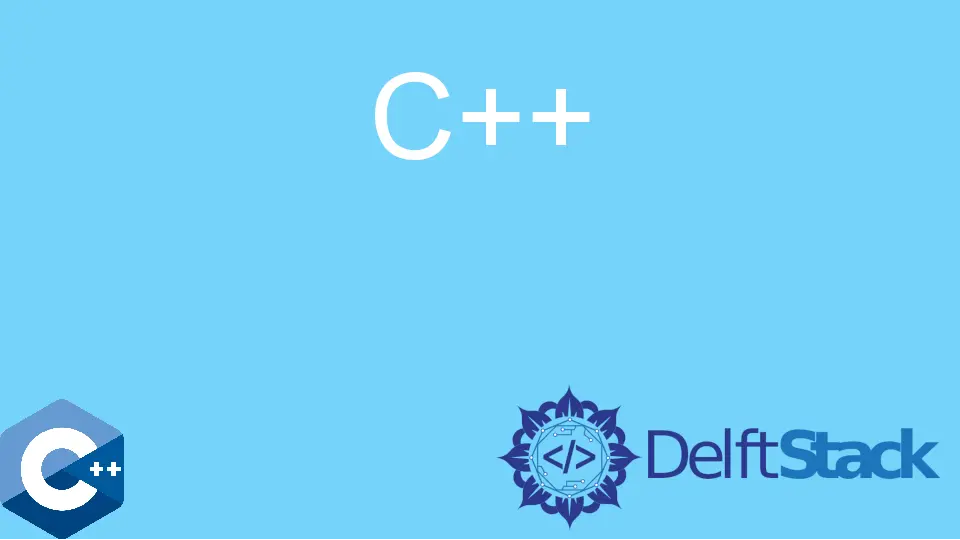
In today’s world, there are multiple number systems that we are dealing with help represent the numbers in different ways. One of them is the Roman number system.
Ancient Romans invented them for counting and performing day-to-day activities. This article will discuss the Roman number system and the program to convert a decimal number into a Roman number.
Roman Numeral Converter in C++
Roman numerals represent a number in a specific format for counting and other purposes.
You must have heard about these Roman numerals in schools and have seen its practical example in clocks. Roman numerals often represent the numbers in the clocks.
Roman Number System is represented by a combination of seven letters from the Latin alphabet. Each of the seven letters or symbols has a definite value attached to it.
The seven letters and their specific values are specified below.
I - 1
V - 5
X - 10
L - 50
C - 100
D - 500
M - 1000
However, one thing to remember is that since after 1000, we do not have any symbol for 10000; therefore, the highest number that the Roman numerals can represent is 3999.
Now, let us talk about how to convert decimal numbers into Roman numerals.
A number in a Roman numeral is written in descending order, from left to right; however, in some cases, to avoid repetition of the same four consecutive characters, such as (IIII or XXXX), the subtractive notation is followed. The subtractive notation is used with the numbers having 4 and 9 in them.
In the subtractive notation, I is placed before V
or X
to make it an IV
(four) or IX
(nine). Similarly, X
is placed before L
or C
to make it an XL
or XC
.
Therefore, symbols in the subtractive notation are made by combining two symbols. Therefore, let us look at the Roman numerals that also include the subtractive notation.
SYMBOL Number
I 1
IV 4
V 5
IX 9
X 10
XL 40
L 50
XC 90
C 100
CD 400
D 500
CM 900
M 1000
Convert a Decimal Number to a Roman Numeral in C++
The procedure to convert an integer into a Roman numeral is as follows:
- Repeat the below process until the given decimal number becomes zero.
- We need to compare the decimal number with the base values in the order specified as 1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1.
- If the base value is smaller than or equal to the given decimal number (largest base value), divide the decimal number by the base value.
- Now, append the corresponding symbol of the base value, the quotient number of times in a variable. For example: if the quotient comes out to be 3 and the base value is 10, then the corresponding representation of 10, that is,
X
, will be repeated thrice asXXX
in the answer. - However, the remainder becomes the new number for further divisions.
- The variable having the symbols appended until the decimal number becomes zero contains the answer.
Let us take the number 2954 as the decimal to be converted to a Roman numeral using the above algorithm.
Output:
MMCMLIV
Explanation:
Stage 1:
- Initially, the decimal number is 2954.
- Since 2954>=1000, the largest base value corresponds to 1000.
- Dividing 2954 by 1000 gives a quotient = 2 and remainder = 954. Since the corresponding symbol for 1000 is
M
, it will be repeated twice. - Therefore, the result, for now, is equal to
MM
. - The remainder becomes the new number for performing the further divisions.
Stage 2:
- Now, the number becomes 954
- Comparing it with the base values 1000>954>=900. Therefore, the largest base value is 900.
- Dividing 954 by 900 gives quotient = 1 and remainder = 54. Since the corresponding symbol for 900 is
CM
, it will be appended once. - Therefore, the result now becomes
MMCM
. - The remainder becomes the new number for performing the further divisions.
Stage 3:
- Now, the number becomes 54.
- Comparing it with the base values 90>54>=50. Therefore, the largest base value is 50.
- Dividing 54 by 50 gives quotient = 1 and remainder = 4. Since the corresponding symbol for 50 is
L
, it will be appended once. - Therefore, the result now becomes
MMCML
. - The remainder becomes the new number for performing the further divisions.
Stage 4:
- Now, the number becomes 4.
- Comparing it with the base values 5>4>=4. Therefore, the largest base value is 4.
- Dividing 4 by 4 gives quotient = 1 and remainder = 0. Since the corresponding symbol for 4 is
IV
, it will be appended once. - Therefore, the result now becomes
MMCMLIV
. - Now, since the remainder becomes zero, the iterations will be stopped, and we have our required answer.
C++ Code Example to Convert a Decimal Number to a Roman Numeral
Let us see a code example to convert a decimal number to a Roman numeral in C++.
#include <bits/stdc++.h>
using namespace std;
int subDig(char n1, char n2, int i, char c[]) {
c[i++] = n1;
c[i++] = n2;
return i;
}
int digit(char ch, int n, int i, char c[]) {
for (int j = 0; j < n; j++) {
c[i++] = ch;
}
return i;
}
int convertRoman(int num) {
char c[10001];
int i = 0;
while (num != 0) {
if (num >= 1000) {
i = digit('M', num / 1000, i, c);
num = num % 1000;
} else if (num >= 500) {
if (num < 900) {
i = digit('D', num / 500, i, c);
num = num % 500;
} else {
i = subDig('C', 'M', i, c);
num = num % 100;
}
} else if (num >= 100) {
if (num < 400) {
i = digit('C', num / 100, i, c);
num = num % 100;
} else {
i = subDig('C', 'D', i, c);
num = num % 100;
}
} else if (num >= 50) {
if (num < 90) {
i = digit('L', num / 50, i, c);
num = num % 50;
} else {
i = subDig('X', 'C', i, c);
num = num % 10;
}
} else if (num >= 10) {
if (num < 40) {
i = digit('X', num / 10, i, c);
num = num % 10;
} else {
i = subDig('X', 'L', i, c);
num = num % 10;
}
} else if (num >= 5) {
if (num < 9) {
i = digit('V', num / 5, i, c);
num = num % 5;
} else {
i = subDig('I', 'X', i, c);
num = 0;
}
} else if (num >= 1) {
if (num < 4) {
i = digit('I', num, i, c);
num = 0;
} else {
i = subDig('I', 'V', i, c);
num = 0;
}
}
}
for (int j = 0; j < i; j++) {
cout << c[j];
}
}
int main() {
int number = 2954;
convertRoman(number);
return 0;
}
Output:
MMCMLIV
In the above code, we have compared the number num
with the base values using the if/else
conditions, and based on this, we have added the corresponding variable to the answer.
However, the numbers which follow subtractive notation have been dealt with separately with the subDig
function in which two letter symbols have been added to the answer.
The code discussed above is quite lengthy; therefore, let us discuss a different approach in which our code boils down to a much shorter than previously discussed.
Approach 2
This approach is based on storing the base values and their corresponding symbols separately in an array and using them while looping around.
Let us see the code for the same.
#include <bits/stdc++.h>
using namespace std;
int convertRoman(int num) {
int base_values[] = {1, 4, 5, 9, 10, 40, 50, 90, 100, 400, 500, 900, 1000};
string symbols[] = {"I", "IV", "V", "IX", "X", "XL", "L",
"XC", "C", "CD", "D", "CM", "M"};
int i = 12;
while (num > 0) {
int quot = num / base_values[i];
num = num % base_values[i];
while (quot--) {
cout << symbols[i];
}
i--;
}
}
int main() {
int number = 2543;
convertRoman(number);
return 0;
}
Output:
MMDXLIII
In the above code, we stored the base values and their corresponding symbols in an array and then compared the number with the base values in decreasing order.
Following this, the number is divided with the largest base value, and the quotient and remainder are found. Now, the corresponding symbol is printed several times using a while
loop.
Conclusion
In this article, we have discussed the conversion of decimal numbers into Roman numbers. Roman Numerals are a way to represent the decimal number with symbols.
Roman Numerals are written in decreasing order and from left to right. However, for a few cases, we use a subtractive notation, in which a smaller symbol is written before a larger one.
These cases arise with the numbers 4 and 9 and are explained in this article.