Complex Numbers in C++
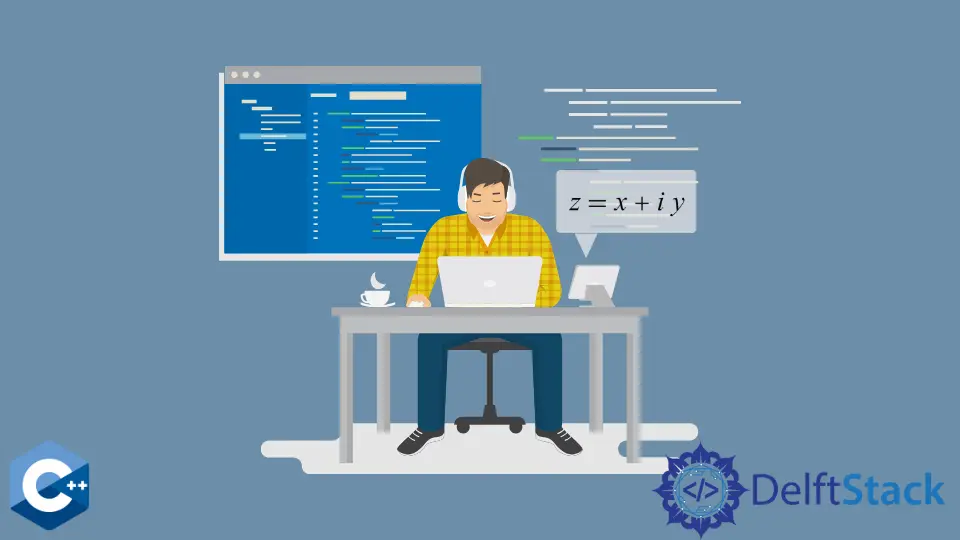
The imaginary number "i"
, which is denoted by the lowercase letter "i"
(or sometimes by the symbol √-1
), is a mathematical constant that’s defined as the square root of a negative one.
The imaginary number "i"
is used in C++ code to represent complex numbers. Complex numbers are composed of two parts, a real part and an imaginary part, and they’re written in the form a+bi
, where a
is the real part, and b
is the imaginary part.
In this post, we will go over the fundamentals of using complex numbers in C++.
Complex Numbers Library in C++
This library is a collection of complex number classes and functions for C++. It provides the following features:
- Complex numbers with arbitrary precision.
- Complex numbers with arbitrary precision and in polar form.
- Complex number arithmetic operations (addition, subtraction, multiplication, division).
- Complex number conversion between polar and rectangular forms.
- Complex number inversion (finding the real part of a complex number).
- Real part extraction from a complex number.
Complex Functions in C++
Complex functions in C++ are a set of functions that perform mathematical operations on complex numbers. They can be used in solving complex problems and equations.
Moreover, Complex functions in C++ are not just the more complex versions of the simple functions. They are different from their counterparts in that they can accept a variable number of arguments and return a value with a variable number of components.
It is important to note that these functions are not part of the standard C++ library but are usually implemented as an extension library.
Let’s discuss some of the C++ complex functions in detail.
- The
sqrt()
function is used to calculate the square root of a number. - The
abs()
function is used to find the absolute value of a number. - The
norm()
function calculates the magnitude of a vector. - The
polar()
functions calculate the magnitude of a vector in polar coordinates. - The
proj()
functions project a point onto a plane defined by two points. - The
arg(x,y)
function returns the angle from one point to another.
Use the Complex Numbers Library in C++
Let’s discuss how to use the Complex Numbers library in C++.
-
Include header files and declare a namespace.
-
Create an object of type complex number that includes two scalar values, one for real and one for the imaginary part.
-
Implement functions that perform arithmetic operations on complex numbers, such as addition, subtraction, multiplication, division and modulus.
-
Call the functions defined in step 3 to work with complex numbers.
Example Code:
#include <complex>
#include <iostream>
using namespace std;
int main() {
complex<double> demo(3.0, 6.0);
cout << "Real Number" << real(demo) << endl;
cout << "Imaginary Number " << imag(demo) << endl;
return 0;
}
Output:
Real Number3
Imaginary Number 6
Click here to check the live demonstration of the code above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook