Pre-Increment VS Post-Increment Operators in C++
- Difference Between Pre-Increment and Post-Increment Operations in C++
- Don’t Use Post-Increment and Pre-Increment Operations With Other Arithmetic Operations of the Same Variable
- When to Use Pre-Increment vs Post-Increment
- Conclusion
- FAQ
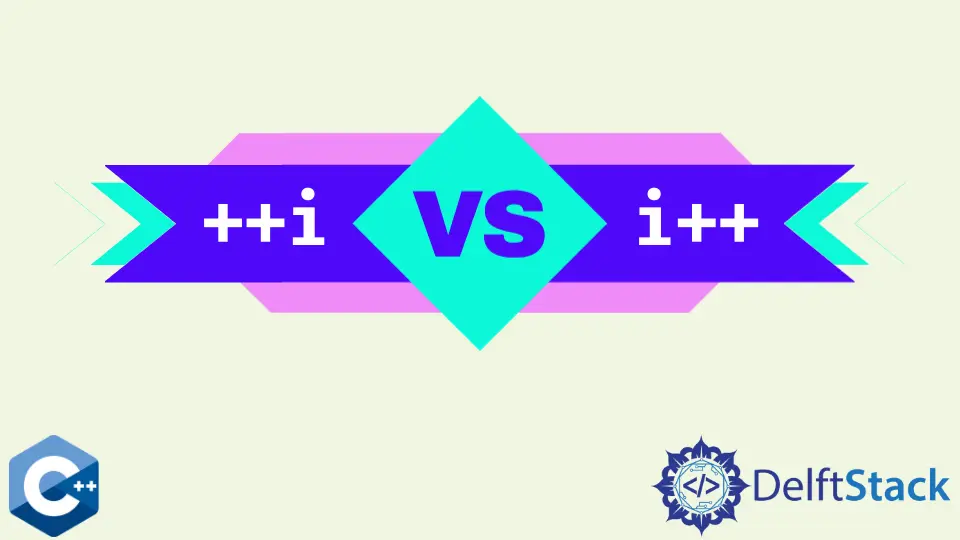
When diving into the world of C++, one of the fundamental concepts you’ll encounter is the use of increment operators. Understanding the difference between pre-increment and post-increment operators is crucial for writing efficient and effective code. These operators can significantly impact how your code behaves, especially in loops and conditional statements.
In this article, we’ll explore the nuances of pre-increment and post-increment operators, providing clear examples to illustrate their differences. Whether you’re a beginner or looking to refresh your knowledge, this guide will help clarify these essential operators in C++. So, let’s get started!
Difference Between Pre-Increment and Post-Increment Operations in C++
Both operations are based on the unary increment operator - ++
, which has slightly different behavior when used in expressions where the variable’s value is accessed. Generally, a variable can be combined with increment or decrement operator after or before that yields the value bigger/smaller by 1. Note though, pre-increment operation modifies the given variable at first and then accesses it. On the other hand, the post-increment operator accesses the original value and then increments it. Thus, when used in an expression where the value is accessed or stored, the operator can have different effects on the program. E.g., the following example code initializes the two integers to 0
values and then iterates five times while outputting the incremented values for each with different operators. Note that post-increment starts printing with 0
and ends with 4
, while pre-increment starts with 1
and finishes with 5
. Nevertheless, the final value stored in both integers is the same - 5
.
#include <iostream>
using std::cout;
using std::endl;
int main() {
int i = 0, j = 0;
while ((i < 5) && (j < 5)) {
cout << "i: " << i++ << " ";
cout << "j: " << ++j << endl;
}
cout << "End of the loop, both have equal values:" << endl;
cout << "i: " << i << " "
<< "j: " << j << endl;
exit(EXIT_SUCCESS);
}
Output:
i: 0 j: 1
i: 1 j: 2
i: 2 j: 3
i: 3 j: 4
i: 4 j: 5
End of the loop, both have equal values:
i: 5 j: 5
Alternatively, using the different notations within the for
loop’s conditional expression behaves the same. Both loops get executed 5
iterations. Although, it’s usually accepted style to use pre-increment, which can be a more performance efficient variant when the code is not optimized.
#include <iostream>
using std::cout;
using std::endl;
int main() {
int i = 0, j = 0;
while ((i < 5) && (j < 5)) {
cout << "i: " << i++ << " ";
cout << "j: " << ++j << endl;
}
cout << "End of the loop, both have equal values:" << endl;
cout << "i: " << i << " "
<< "j: " << j << endl;
cout << endl;
for (int k = 0; k < 5; ++k) {
cout << k << " ";
}
cout << endl;
for (int k = 0; k < 5; k++) {
cout << k << " ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
Output:
i: 0 j: 1
i: 1 j: 2
i: 2 j: 3
i: 3 j: 4
i: 4 j: 5
End of the loop, both have equal values:
i: 5 j: 5
0 1 2 3 4
0 1 2 3 4
Don’t Use Post-Increment and Pre-Increment Operations With Other Arithmetic Operations of the Same Variable
Note that post-increment and pre-increment operators can make arithmetic calculations entirely erroneous when used with the same variable. For example, combining the post-increment with the multiplication of the same variable makes unintuitive code to read and even error-prone if not treated with enough diligence.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> vec1 = {10, 22, 13, 41, 51, 15};
for (int &k : vec1) {
cout << k++ * k << ", ";
}
exit(EXIT_SUCCESS);
}
Output:
110, 506, 182, 1722, 2652, 240,
When to Use Pre-Increment vs Post-Increment
Choosing between pre-increment and post-increment can affect performance, especially in complex expressions or loops. In most cases, the performance difference is negligible. However, there are scenarios where pre-increment might be faster, particularly with iterators in C++ Standard Template Library (STL). Pre-increment can avoid unnecessary copies of objects, making it a better choice in performance-critical applications.
For instance, in a loop iterating through a container, using pre-increment can minimize overhead:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> numbers = {1, 2, 3, 4, 5};
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
cout << *it << " ";
}
return 0;
}
Output:
1 2 3 4 5
In this example, the pre-increment operator (++it) is used to move the iterator to the next position. This is generally preferred over post-increment because it avoids creating a temporary copy of the iterator, which can be more efficient, especially with larger data structures.
Conclusion
Understanding the differences between pre-increment and post-increment operators in C++ is vital for writing clear and efficient code. While both operators serve the same purpose of incrementing a variable, their timing in relation to the expression can lead to different outcomes. By mastering these operators, you can better control your code’s behavior and improve performance in your applications. Remember, practice makes perfect, so don’t hesitate to experiment with these concepts in your own coding projects.
FAQ
-
What is the main difference between pre-increment and post-increment operators?
The main difference lies in when the increment occurs in relation to the expression. Pre-increment increments the value before it is used, while post-increment increments it after. -
Which operator is generally faster in C++?
Pre-increment is often faster, especially when dealing with iterators in STL, as it avoids creating unnecessary copies. -
Can I use pre-increment and post-increment in loops?
Yes, both operators can be used in loops, but pre-increment is usually preferred for iterators to enhance performance. -
Are there any scenarios where post-increment is more beneficial?
Post-increment can be more intuitive in certain situations where you need the original value before incrementing, such as in certain arithmetic operations. -
Do pre-increment and post-increment work the same way in all programming languages?
No, while many languages have similar increment operators, the behavior may vary slightly, so it’s essential to refer to the specific language documentation.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook