The Addition Assignment Operator and Increment Operator in C++
-
Addition Assignment Operator
+=
in C++ -
Postfix Increment and Decrement Operators
++
in C++ -
the Difference Between
+=
and++
Operators in C++
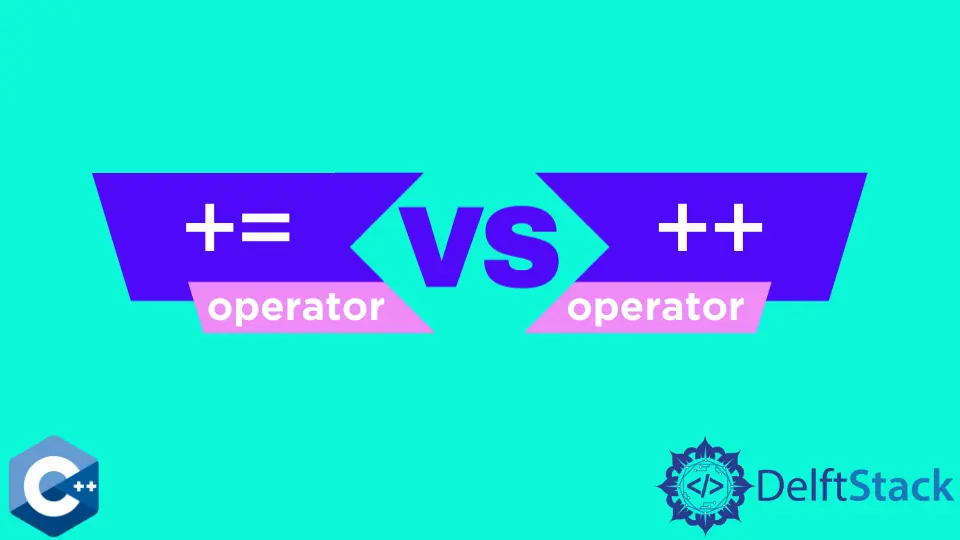
The article will discuss the concept and usage of addition assignment operators +=
and increment operators ++
in C++.
Addition Assignment Operator +=
in C++
The +=
addition assignment operator adds a value to a variable and assigns its result. The two types of operands determine the behavior of the +=
addition assignment operator.
Example:
#include <iostream>
using namespace std;
int main() {
int a = 10;
printf("Value of a is %d\n", a);
// Assigning value by adding 10 to variable a
// using "+=" operator
a += 10;
printf("Value of a is %d\n", a);
}
Output:
Value of a is 10
Value of a is 20
Postfix Increment and Decrement Operators ++
in C++
The operators appear after the postfix expression. The result of using the postfix increment operator ++
is that the value of the operand increases by one unit of the corresponding type.
Please note that the postfix increment or decrement expression evaluates its value before applying the corresponding operator.
Suppose the postfix operator is applied to the function argument. In that case, the increment or decrement of the argument value will not necessarily be performed before it is passed to the function.
An example of a postfix increment operator is shown below.
#include <iostream>
using namespace std;
int main() {
int i = 10;
i++;
cout << i << endl;
}
Output:
11
the Difference Between +=
and ++
Operators in C++
Both +=
and ++
operators increase the value of n by 1
.
The difference is that the return is the pre-increment value in the post-increment operator ++
. In contrast, the addition assignment operator +=
case returns the post-increment value.
First Case: post-increment ++
operator.
#include <iostream>
using namespace std;
int main() {
int n = 5;
int new_var;
new_var = n++;
printf("Output: ");
printf("%d", new_var);
}
Output:
Output: 5
Second Case: addition assignment +=
operator.
#include <iostream>
using namespace std;
int main() {
int n = 5;
int new_var;
n += 1;
new_var = n;
printf("Output: ");
printf("%d", new_var);
}
Output:
Output: 6