How to Sleep for Milliseconds in C++
-
Use
std::this_thread::sleep_for
Method to Sleep in C++ -
Use
usleep
Function to Sleep in C++ -
Use
nanosleep
Function to Sleep in C++
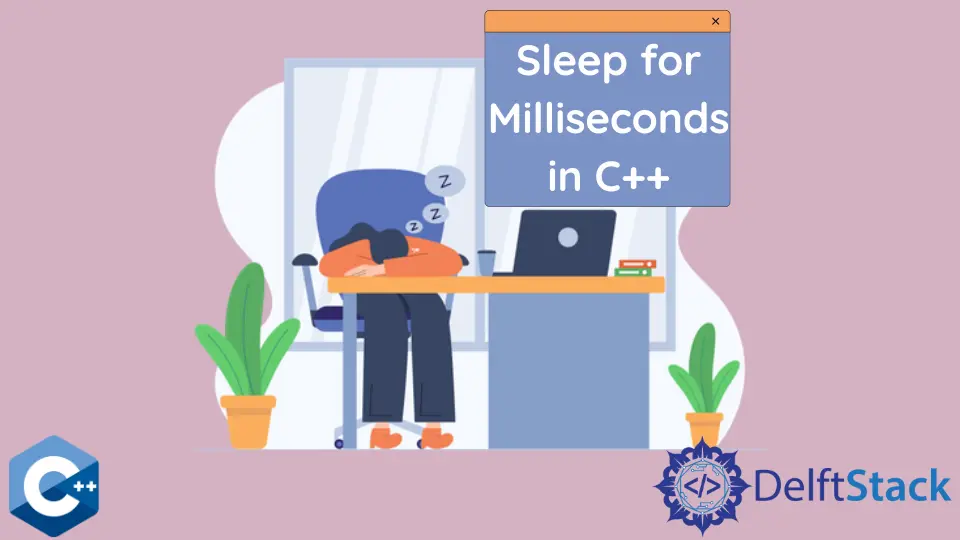
This article introduces methods to sleep for milliseconds in C++.
Use std::this_thread::sleep_for
Method to Sleep in C++
This method is a pure C++ version of the sleep
function from the <thread>
library, and it’s the portable version for both Windows and Unix platforms. For a better example demonstration, we are suspending the process for 3000 milliseconds.
#include <chrono>
#include <iostream>
#include <thread>
using std::cin;
using std::cout;
using std::endl;
using std::this_thread::sleep_for;
constexpr int TIME_TO_SLEEP = 3000;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
sleep_for(std::chrono::milliseconds(TIME_TO_SLEEP));
}
}
return 0;
}
Output:
Started loop...
Iteration - 0
Iteration - 1
Iteration - 2
Iteration - 3
Iteration - 4
Sleeping ....
Iteration - 5
Iteration - 6
Iteration - 7
Iteration - 8
Iteration - 9
We can rewrite the above code with more eloquent version using the std::chrono_literals
namespace:
#include <iostream>
#include <thread>
using std::cin;
using std::cout;
using std::endl;
using std::this_thread::sleep_for;
using namespace std::chrono_literals;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
sleep_for(3000ms);
}
}
return 0;
}
Use usleep
Function to Sleep in C++
usleep
is a POSIX specific function defined in the <unistd.h>
header, which accepts the number of microseconds as an argument, which should be unsigned integer type and is capable of holding integers in the range [0,1000000].
#include <unistd.h>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
constexpr int TIME_TO_SLEEP = 3000;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
usleep(TIME_TO_SLEEP * 1000);
;
}
}
return 0;
}
Alternatively, we can define a custom macro using the usleep
function and make a more reusable code snippet.
#include <unistd.h>
#include <iostream>
#define SLEEP_MS(milsec) usleep(milsec * 1000)
using std::cin;
using std::cout;
using std::endl;
constexpr int TIME_TO_SLEEP = 3000;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
SLEEP_MS(TIME_TO_SLEEP);
}
}
return 0;
}
Use nanosleep
Function to Sleep in C++
nanosleep
function is another POSIX specific version that offers better handling of interrupts and has a finer resolution of the sleep interval. Namely, a programmer creates a timespec
structure to specify the number of seconds and nanoseconds separately. nanosleep
also takes the second timespec
struct parameter to return the remaining time interval in case the program gets interrupted by a signal. Note that the programmer is responsible for interrupt handling.
#include <ctime>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
constexpr int SECS_TO_SLEEP = 3;
constexpr int NSEC_TO_SLEEP = 3;
int main() {
struct timespec request {
SECS_TO_SLEEP, NSEC_TO_SLEEP
}, remaining{SECS_TO_SLEEP, NSEC_TO_SLEEP};
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
nanosleep(&request, &remaining);
}
}
return 0;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook