C++에서 밀리 초 동안 잠자는 방법
-
std::this_thread::sleep_for
메서드를 사용하여 C++에서 잠자기 -
usleep
함수를 사용하여 C++에서 잠자기 -
nanosleep
함수를 사용하여 C++에서 잠자기
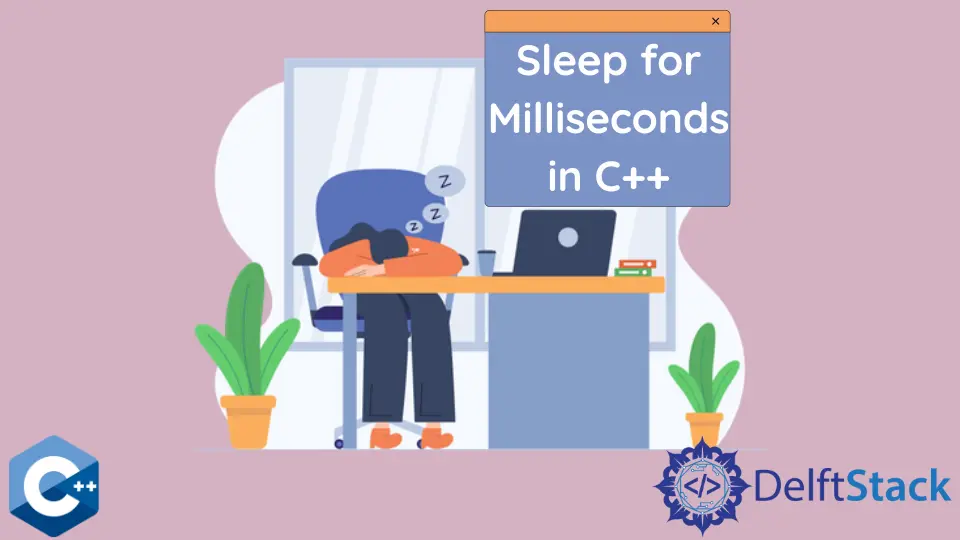
이 기사에서는 C++에서 밀리 초 동안 대기하는 방법을 소개합니다.
std::this_thread::sleep_for
메서드를 사용하여 C++에서 잠자기
이 메서드는<thread>
라이브러리의sleep
함수의 순수한 C++ 버전이며 Windows 및 Unix 플랫폼 모두를위한 이식 가능한 버전입니다. 더 나은 예제 데모를 위해 3000 밀리 초 동안 프로세스를 일시 중단합니다.
#include <chrono>
#include <iostream>
#include <thread>
using std::cin;
using std::cout;
using std::endl;
using std::this_thread::sleep_for;
constexpr int TIME_TO_SLEEP = 3000;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
sleep_for(std::chrono::milliseconds(TIME_TO_SLEEP));
}
}
return 0;
}
출력:
Started loop...
Iteration - 0
Iteration - 1
Iteration - 2
Iteration - 3
Iteration - 4
Sleeping ....
Iteration - 5
Iteration - 6
Iteration - 7
Iteration - 8
Iteration - 9
std::chrono_literals
네임 스페이스를 사용하여보다 웅변적인 버전으로 위 코드를 다시 작성할 수 있습니다.
#include <iostream>
#include <thread>
using std::cin;
using std::cout;
using std::endl;
using std::this_thread::sleep_for;
using namespace std::chrono_literals;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
sleep_for(3000ms);
}
}
return 0;
}
usleep
함수를 사용하여 C++에서 잠자기
usleep
은<unistd.h>
헤더에 정의 된 POSIX 특정 함수로, 인수로 마이크로 초 수를 허용하며, 부호없는 정수 유형이어야하며 [0,1000000]
범위의 정수를 보유 할 수 있습니다.
#include <unistd.h>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
constexpr int TIME_TO_SLEEP = 3000;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
usleep(TIME_TO_SLEEP * 1000);
;
}
}
return 0;
}
또는 usleep
함수를 사용하여 커스텀 매크로를 정의하고 더 재사용 가능한 코드 스 니펫을 만들 수 있습니다.
#include <unistd.h>
#include <iostream>
#define SLEEP_MS(milsec) usleep(milsec * 1000)
using std::cin;
using std::cout;
using std::endl;
constexpr int TIME_TO_SLEEP = 3000;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
SLEEP_MS(TIME_TO_SLEEP);
}
}
return 0;
}
nanosleep
함수를 사용하여 C++에서 잠자기
nanosleep
함수는 인터럽트 처리를 개선하고 절전 간격을 더 정밀하게 처리하는 또 다른 POSIX 특정 버전입니다. 즉, 프로그래머는 timespec
구조를 생성하여 초와 나노초를 별도로 지정합니다. nanosleep
은 또한 두 번째timespec
구조체 매개 변수를 사용하여 프로그램이 신호에 의해 중단되는 경우 남은 시간 간격을 반환합니다. 프로그래머는 인터럽트 처리를 담당합니다.
#include <ctime>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
constexpr int SECS_TO_SLEEP = 3;
constexpr int NSEC_TO_SLEEP = 3;
int main() {
struct timespec request {
SECS_TO_SLEEP, NSEC_TO_SLEEP
}, remaining{SECS_TO_SLEEP, NSEC_TO_SLEEP};
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
nanosleep(&request, &remaining);
}
}
return 0;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook