如何在 C++ 中实现毫秒级的睡眠
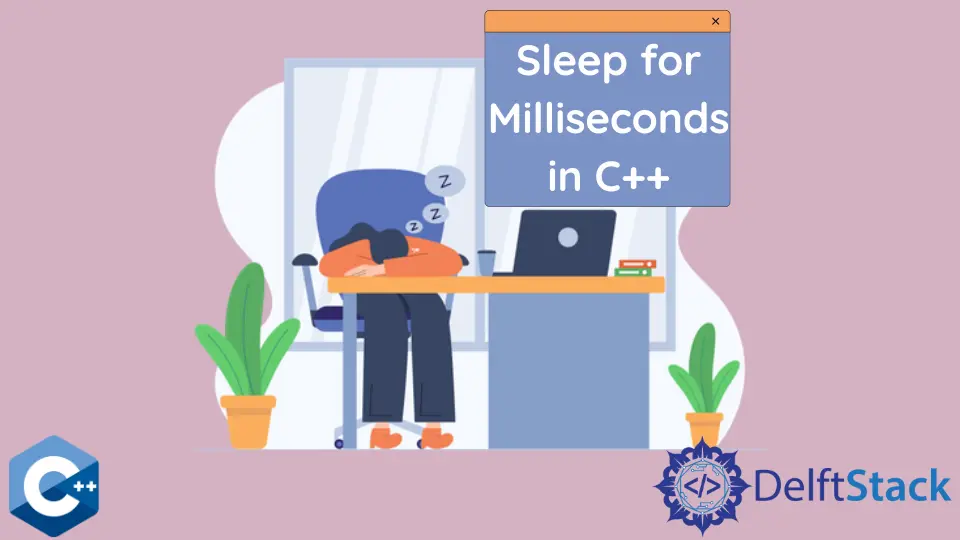
本文介绍了在 C++ 中睡眠毫秒的方法。
使用 std::this_thread::sleep_for
方法在 C++ 中睡眠
这个方法是 <thread>
库中 sleep
函数的纯 C++ 版本,它是 Windows 和 Unix 平台的可移植版本。为了更好地演示示例,我们暂停进程 3000 毫秒。
#include <chrono>
#include <iostream>
#include <thread>
using std::cin;
using std::cout;
using std::endl;
using std::this_thread::sleep_for;
constexpr int TIME_TO_SLEEP = 3000;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
sleep_for(std::chrono::milliseconds(TIME_TO_SLEEP));
}
}
return 0;
}
输出:
Started loop...
Iteration - 0
Iteration - 1
Iteration - 2
Iteration - 3
Iteration - 4
Sleeping ....
Iteration - 5
Iteration - 6
Iteration - 7
Iteration - 8
Iteration - 9
我们可以使用 std::chono_literals
命名空间重写上面的代码,使其更有说服力。
#include <iostream>
#include <thread>
using std::cin;
using std::cout;
using std::endl;
using std::this_thread::sleep_for;
using namespace std::chrono_literals;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
sleep_for(3000ms);
}
}
return 0;
}
在 C++ 中使用 usleep
函数进行睡眠
usleep
是在 <unistd.h>
头中定义的一个 POSIX 专用函数,它接受微秒数作为参数,它应该是无符号整数类型,能够容纳 [0,1000000]范围内的整数。
#include <unistd.h>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
constexpr int TIME_TO_SLEEP = 3000;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
usleep(TIME_TO_SLEEP * 1000);
;
}
}
return 0;
}
另外,我们也可以使用 usleep
函数定义一个自定义的宏,并做出一个更可重用的代码片段。
#include <unistd.h>
#include <iostream>
#define SLEEP_MS(milsec) usleep(milsec * 1000)
using std::cin;
using std::cout;
using std::endl;
constexpr int TIME_TO_SLEEP = 3000;
int main() {
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
SLEEP_MS(TIME_TO_SLEEP);
}
}
return 0;
}
使用 nanosleep
函数在 C++ 中休眠
nanosleep
函数是另一个 POSIX 特定版本,它提供了更好的处理中断的功能,并且对睡眠间隔有更精细的分辨率。也就是说,程序员创建一个 timespec
结构,分别指定秒数和纳秒数。nanosleep
也会取第二个 timespec
结构参数来返回剩余的时间间隔,以防程序被信号打断。注意,中断处理由程序员负责。
#include <ctime>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
constexpr int SECS_TO_SLEEP = 3;
constexpr int NSEC_TO_SLEEP = 3;
int main() {
struct timespec request {
SECS_TO_SLEEP, NSEC_TO_SLEEP
}, remaining{SECS_TO_SLEEP, NSEC_TO_SLEEP};
cout << "Started loop.." << endl;
for (int i = 0; i < 10; ++i) {
cout << "Iteration - " << i << endl;
if (i == 4) {
cout << "Sleeping ...." << endl;
nanosleep(&request, &remaining);
}
}
return 0;
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe