How to Use a PI Constant in C++
- Defining PI as a Constant Variable
- Using the Math Library for PI
-
Use
std::numbers::pi
Constant From C++20 - Creating a Custom PI Function
- Conclusion
- FAQ
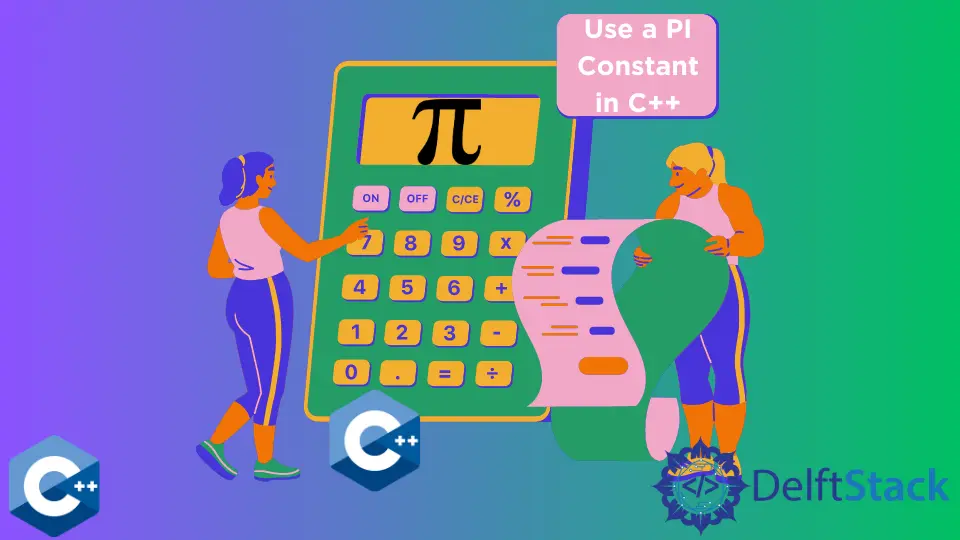
When it comes to programming in C++, using mathematical constants like PI can be essential for various computations, especially in fields such as physics, engineering, and computer graphics. PI, approximately equal to 3.14159, is vital for calculations involving circles, spheres, and other geometric shapes.
In this article, we will delve into the various ways to define and use the constant value of PI in your C++ programs. Whether you’re a beginner or an experienced developer, understanding how to effectively incorporate constants like PI can enhance your coding efficiency and accuracy. Let’s explore the different methods to implement this crucial mathematical constant in C++.
Defining PI as a Constant Variable
One of the simplest methods to use the value of PI in C++ is by defining it as a constant variable. This approach allows you to assign a specific value to PI that can be reused throughout your program without the risk of modification.
Here’s how you can define PI as a constant variable:
#include <iostream>
using namespace std;
const double PI = 3.14159;
int main() {
double radius = 5.0;
double area = PI * radius * radius;
cout << "Area of the circle: " << area << endl;
return 0;
}
Output:
Area of the circle: 78.53975
In this example, we first include the necessary headers and use the std
namespace. We then define PI as a constant variable using the const
keyword, ensuring that its value remains unchanged throughout the program. In the main
function, we calculate the area of a circle by multiplying PI with the square of the radius. Finally, we output the result. This method is straightforward and effective, making it a popular choice among C++ developers.
Using the Math Library for PI
Another efficient way to get the value of PI in C++ is by utilizing the cmath
library, which provides a predefined constant for PI starting from C++11. This method is particularly useful as it ensures that you are using a highly accurate value of PI.
Here’s how to use the cmath
library to access PI:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double radius = 5.0;
double circumference = 2 * M_PI * radius;
cout << "Circumference of the circle: " << circumference << endl;
return 0;
}
Output:
Circumference of the circle: 31.4159
In this code snippet, we include the cmath
library, which contains the constant M_PI
. This constant represents the value of PI with high precision. We calculate the circumference of a circle using the formula 2 * M_PI * radius
. This method is not only concise but also leverages the accuracy of the mathematical library, making it a robust choice for calculations involving PI.
Use std::numbers::pi
Constant From C++20
Since the C++20 standard, the language supports the mathematical constants defined in the <numbers>
header. These constants are supposed to offer better cross-platform compliance, but it is still in the early days, and various compilers might not support it yet. The full list of the constants can be seen here.
#include <iomanip>
#include <iostream>
#include <numbers>
using std::cout;
using std::endl;
int main() {
cout << "pi = " << std::setprecision(16) << std::numbers::pi << endl;
cout << "pi * 2 = " << std::setprecision(16) << std::numbers::pi * 2 << endl;
cout << endl;
return EXIT_SUCCESS;
}
pi = 3.141592653589793
pi * 2 = 6.283185307179586
Creating a Custom PI Function
If you prefer more flexibility, you can create a custom function to return the value of PI. This method allows you to encapsulate the logic of retrieving PI and can be helpful if you want to implement additional functionalities in the future.
Here’s how to create a custom function for PI:
#include <iostream>
using namespace std;
double getPI() {
return 3.14159;
}
int main() {
double radius = 5.0;
double area = getPI() * radius * radius;
cout << "Area of the circle: " << area << endl;
return 0;
}
Output:
Area of the circle: 78.53975
In this example, we define a function called getPI
that returns the constant value of PI. Inside the main
function, we call getPI
to calculate the area of the circle. This approach not only makes the code cleaner but also allows for easy updates to the PI value in the future if needed. By abstracting the constant value into a function, you maintain flexibility in your code.
Conclusion
In conclusion, using the constant value of PI in C++ is a fundamental skill that can greatly enhance your programming capabilities. Whether you choose to define PI as a constant variable, use the cmath
library, or create a custom function, each method has its advantages. The choice ultimately depends on your specific needs and coding style. By mastering these techniques, you’ll be well-equipped to handle a variety of mathematical computations in your C++ projects.
FAQ
-
What is the value of PI in C++?
The value of PI in C++ can be defined as approximately 3.14159, or you can use the predefined constantM_PI
from thecmath
library. -
Can I change the value of PI in my program?
If you define PI as a constant using theconst
keyword, you cannot change its value. However, if you use a variable, you can modify it, but this is generally not recommended for mathematical constants. -
Is it necessary to include the
cmath
library to use PI?
No, it’s not necessary, but including thecmath
library provides a more accurate predefined value of PI. -
What are the benefits of using a custom function for PI?
A custom function allows greater flexibility, making it easier to update the value of PI or add additional logic related to the constant in the future. -
Can I use PI in classes or structures in C++?
Yes, you can use PI in classes or structures just like any other variable or constant. You can define it as a member variable or a static constant.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook