How to Round a Double to an Int in C++
-
Use the
round()
Function fordouble
toint
Rounding in C++ -
Use the
lround()
Function fordouble
toint
Rounding in C++ -
Use the
trunc()
Function fordouble
toint
Rounding in C++ - Conclusion
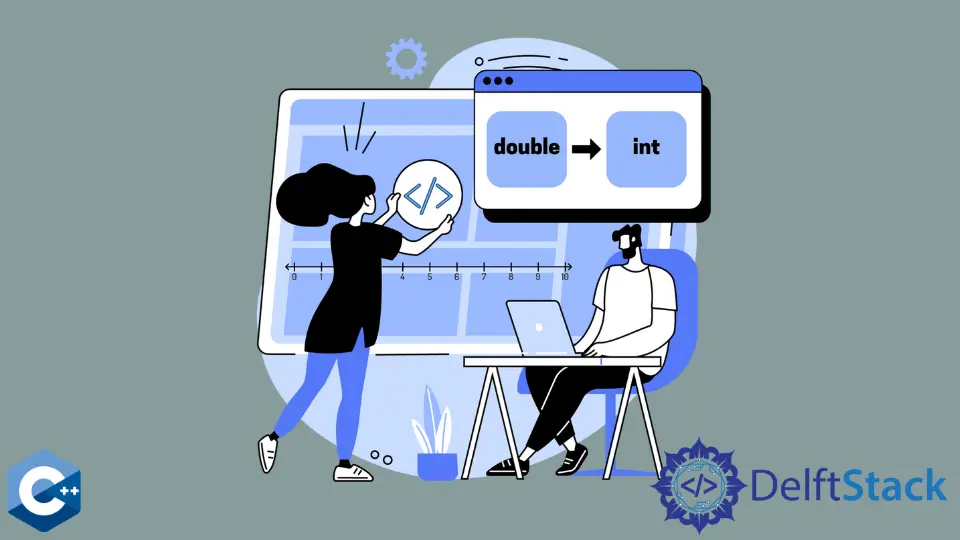
This article will explain several methods of how to round a double to an integer in C++.
By delving into the applications of the round()
, lround()
, and trunc()
functions from the <cmath>
header, we aim to provide you with code examples to help you effectively round double-precision floating-point numbers to integers.
Use the round()
Function for double
to int
Rounding in C++
The round()
function is defined in the <cmath>
header. It can compute the nearest integer value of the argument that the user passes.
Halfway cases are rounded away from zero, and the returned value is the same type as the argument. In the following example, we initialize an arbitrary vector of doubles and output the round()
function for each element.
Basic Syntax:
#include <cmath>
double roundedValue = std::round(someDouble);
This line rounds the someDouble
variable to the nearest integer using the round()
function from the <cmath>
header in C++.
Parameter:
someDouble
: This is the parameter representing thedouble
value that you want to round.
Code Example:
#include <cmath>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> dfloats{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
for (auto &item : dfloats) {
cout << "round(" << item << ") = " << round(item) << endl;
}
return EXIT_SUCCESS;
}
Output:
round(-3.5) = -4
round(-21.1) = -21
round(-1.99) = -2
round(0.129) = 0
round(2.5) = 3
round(3.111) = 3
In this code, we have declared a vector of doubles named dfloats
containing six double-precision floating-point values. The program then enters a for
loop, iterating over each element in the vector.
Within the loop, we use the round()
function from the <cmath>
header to round each double value to the nearest integer. The output is displayed using cout
, indicating the original double value and its corresponding rounded integer.
The loop prints the results for each element in the vector. Finally, the program returns EXIT_SUCCESS
, indicating successful execution.
The output of the program is a set of lines displaying the rounded values for each element in the dfloats
vector, showcasing the rounding operation applied to each double value in the provided vector.
Use the lround()
Function for double
to int
Rounding in C++
Alternatively, we can use the lround()
function, which behaves almost identically as the round()
with one difference: it returns the value in a long
integer format. Note that, for both functions, the return value can be unexpected, caused by different rounding errors, which are ubiquitous.
Corresponding scenarios should be handled as specified in the documentation here.
Basic Syntax:
#include <cmath>
long roundedValue = std::lround(someDouble);
Parameter:
someDouble
: This is the parameter representing thedouble
value that you want to convert to the nearest integer.
Code Example:
#include <cmath>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> dfloats{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
for (auto &item : dfloats) {
cout << "round(" << item << ") = " << lround(item) << endl;
}
return EXIT_SUCCESS;
}
Output:
round(-3.5) = -4
round(-21.1) = -21
round(-1.99) = -2
round(0.129) = 0
round(2.5) = 3
round(3.111) = 3
In this code, we initialize a vector named dfloats
containing six double-precision floating-point values. Subsequently, we enter a for
loop that iterates over each element in the vector.
Within the loop, we utilize the lround()
function from the <cmath>
header to round each double value to the nearest integer, and we print the original double value along with its corresponding rounded integer using cout
. The program concludes by returning EXIT_SUCCESS
, signifying successful execution.
The output of the program will display lines containing the rounded values for each element in the dfloats
vector, highlighting the rounding operation applied to each double value in the provided vector.
Use the trunc()
Function for double
to int
Rounding in C++
trunc()
is another useful function from the <cmath>
header, which computes the nearest integer not greater than the number passed as an argument. trunc()
calculates the return value in the floating-point format as round()
.
Note that these two functions don’t take any effect from the current rounding mode. Error handling cases are described on the manual page.
Basic Syntax:
#include <cmath>
double truncatedValue = std::trunc(someDouble);
Parameter:
someDouble
: This is the parameter representing thedouble
value that you want to truncate.
Code Example:
#include <cmath>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> dfloats{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
for (auto &item : dfloats) {
cout << "round(" << item << ") = " << trunc(item) << endl;
}
return EXIT_SUCCESS;
}
Output:
round(-3.5) = -3
round(-21.1) = -21
round(-1.99) = -1
round(0.129) = 0
round(2.5) = 2
round(3.111) = 3
In this code, we begin by declaring a vector named dfloats
containing six double-precision floating-point values. Moving on, we enter a for
loop that iterates over each element in the vector.
Inside the loop, we use the trunc()
function from the <cmath>
header to truncate each double value, effectively removing the fractional part and leaving only the integer part. We print the original double value alongside its truncated integer counterpart using cout
.
Finally, the program returns EXIT_SUCCESS
, indicating successful execution. The output of the program will display lines containing the truncated values for each element in the dfloats
vector, showcasing the truncation operation applied to each double value in the provided vector.
Conclusion
We’ve explored the application of the round()
function from the <cmath>
header, which computes the nearest integer value for a given double. The code example illustrated a for
loop iterating through a vector of doubles, applying the round()
function to each element and displaying the original and rounded values.
Additionally, the article introduced the lround()
function, which behaves similarly to round()
but returns the result as a long integer. The usage and output of lround()
were demonstrated in a code snippet parallel to the first example.
Furthermore, the article covered the trunc()
function, emphasizing its ability to truncate the fractional part of a double to produce an integer. Similar to the previous examples, a code snippet showcased the application of trunc()
in rounding doubles within a vector.
Overall, the article has presented concise syntax, parameters, code examples, and outputs for each method, offering a comprehensive guide for rounding double values in C++.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook