C++ で double を整数に丸める方法
胡金庫
2023年10月12日
C++
C++ Double
-
round()
関数を使用してdouble
を整数に丸める -
関数
lround()
を用いてdouble
を最も近い整数に変換する -
ダブルからインテットへの丸めには
trunc()
関数を利用する
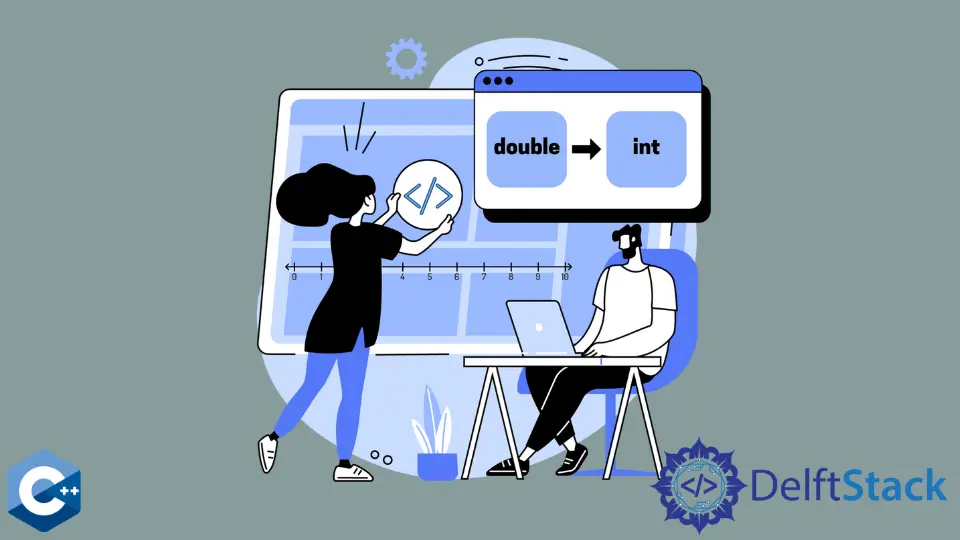
この記事では、C++ でダブルを整数に丸める方法をいくつか説明します。
round()
関数を使用して double
を整数に丸める
関数 round()
は <cmath>
ヘッダで定義されています。これはユーザが渡した引数の最も近い整数値を計算することができます。中途半端な場合はゼロから四捨五入され、返される値は引数と同じ型になります。次の例では、任意の倍数のベクトルを初期化し、各要素について round()
関数を出力します。
#include <cmath>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> dfloats{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
for (auto &item : dfloats) {
cout << "round(" << item << ") = " << round(item) << endl;
}
return EXIT_SUCCESS;
}
出力:
round(-3.5) = -4
round(-21.1) = -21
round(-1.99) = -2
round(0.129) = 0
round(2.5) = 3
round(3.111) = 3
関数 lround()
を用いて double
を最も近い整数に変換する
これは round()
とほぼ同じ振る舞いをしますが、1つだけ違いがあります: 値を long
整数形式で返します。どちらの関数も、異なる丸め誤差が原因で予期せぬ値が返ってくる可能性があることに注意してください。対応するシナリオは、ドキュメントはこちらで指定されている通りに処理すべきです。
#include <cmath>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> dfloats{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
for (auto &item : dfloats) {
cout << "round(" << item << ") = " << lround(item) << endl;
}
return EXIT_SUCCESS;
}
ダブルからインテットへの丸めには trunc()
関数を利用する
これは引数に渡された数値よりも大きくない最も近い整数を計算するものです。trunc()
は、round()
と同様に浮動小数点形式で戻り値を計算します。これら 2つの関数は現在の丸めモードの影響を受けないことに注意してください。エラー処理については、マニュアルページに記載されています。
#include <cmath>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> dfloats{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
for (auto &item : dfloats) {
cout << "round(" << item << ") = " << trunc(item) << endl;
}
return EXIT_SUCCESS;
}
round(-3.5) = -3
round(-21.1) = -21
round(-1.99) = -1
round(0.129) = 0
round(2.5) = 2
round(3.111) = 3
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: 胡金庫